Imperative Programming Languages Overview
Imperative programming languages support basic data types, arithmetic operators, assignment, looping, branching, control structures, I/O commands, procedures, functions, error handling, and library support for data structures. Changes in imperative programming are most useful when combined in a specific order to accomplish tasks efficiently. Runtime storage organization in imperative programming is crucial for managing data effectively. Understanding computer memory and addressing concepts, such as pointers, is essential for manipulating variables and memory contents in imperative languages like C.
Download Presentation
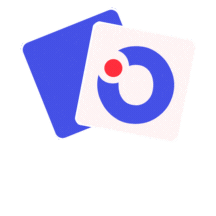
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
CS314 Section 5 Recitation 6 Long Zhao (lz311@rutgers.edu) Imperative Programming Languages Pointer in C Slides available at http://www.ilab.rutgers.edu/~lz311/CS314
Imperative Programming Languages Imperative languages support basic data types (e.g. integers), basic arithmetic operators, assignment, sequencing, looping and branching. Modern imperative languages generally also include features such as Expressions and assignment Control structures (loops, decisions) I/O commands Procedures and functions Error and exception handling Library support for data structures
Imperative Programming Languages Changes are most useful when we can chain them together That means we need some way of specifying that We want to do several things in a row And we want them done in a specific order
Imperative Programming Languages Run-time storage organization
Computer Memory Revisited Computers store data in memory slots Each slot has an unique address Variables store their values like this: Addr 1000 Content i: 37 Addr 1001 Content j: 46 Addr 1002 Content k: 58 Addr 1003 Content m: 74 1004 a[0]: a 1005 a[1]: b 1006 a[2]: c 1007 a[3]: \0 1008 ptr: 1001 1009 1010 1011 5
Computer Memory Revisited Altering the value of a variable is indeed changing the content of the memory e.g. i = 40; a[2] = z ; Addr 1000 Content i: 40 Addr 1001 Content j: 46 Addr 1002 Content k: 58 Addr 1003 Content m: 74 1004 a[0]: a 1005 a[1]: b 1006 a[2]: z 1007 a[3]: \0 1008 ptr: 1001 1009 1010 1011 6
Addressing Concept Pointer stores the address of another entity It refers to a memory location int i = 5; int *ptr; /* declare a pointer variable */ ptr = &i; /* store address-of i to ptr */ printf( *ptr = %d\n , *ptr); /* refer to referee of ptr */ 7
What actually ptr ptr is? ptr i int i = 5; 5 int *ptr; int i = 5; ptr i int *ptr; 5 ptr = &i; 8
What actually ptr ptr is? ptr is a variable storing an address ptr is NOT storing the actual value of i ptr address of i int i = 5; int *ptr; i 5 ptr = &i; printf( i = %d\n , i); Output: printf( *ptr = %d\n , *ptr); i = 5 value of ptr = address of i in memory printf( ptr = %p\n , ptr); *ptr = 5 ptr = effff5e0 9
Twin Operators &: Address-of operator Get the address of an entity e.g.ptr = &j; Addr 1000 Content i: 40 Addr 1001 Content j: 33 Addr 1002 Content k: 58 Addr 1003 Content m: 74 1004 ptr: 1001 1005 1006 1007 10
Twin Operators *: De-reference operator Refer to the content of the referee e.g. *ptr = 99; Addr 1000 Content i: 40 Addr 1001 Content j: 99 Addr 1002 Content k: 58 Addr 1003 Content m: 74 1004 ptr: 1001 1005 1006 1007 11
Example: Pass by Reference Modify behaviour in argument passing void f(int j, int k) { j = 5; k = 6; } void g() { int i = 3, h = 4; f(i, h); } void f(int *ptrj, int *ptrk) { *ptrj = 5; *ptrk = 6; } void g() { int i = 3, h = 4; f(&i, &h); } i = ? h = ? i = 5, h = 6 i = ? h = ? i = 3, h = 4 12
An Illustration int i = 5, j = 10; int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j Type int int Value 5 10 ptr = &j; integer variable integer variable **pptr = 9; *pptr = &i; *ptr = -2; 13
An Illustration int i = 5, j = 10; int *ptr; /* declare a pointer-to-integer variable */ int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr Type int int int * Value 5 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; *ptr = -2; 14
An Illustration int i = 5, j = 10; int *ptr; int **pptr; /* declare a pointer-to-pointer-to-integer variable */ ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr Type int int int * int ** integer pointer pointer variable Value 5 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; *ptr = -2; Double Indirection 15
An Illustration int i = 5, j = 10; int *ptr; int **pptr; ptr = &i; /* store address-of i to ptr */ pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *ptr Type int int int * int ** integer pointer pointer variable int de-reference of ptr Value 5 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i *ptr = -2; 5 16
An Illustration int i = 5, j = 10; ptr j pptr i 10 5 int *ptr; int **pptr; ptr = &i; pptr = &ptr; /* store address-of ptr to pptr */ Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *pptr Type int int int * int ** integer pointer pointer variable int * de-reference of pptr Value 5 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i address of ptr value of ptr (address of i) *ptr = -2; 17
An Illustration (Exercise) int i = 5, j = 10; ptr j pptr i 10 5 int *ptr; int **pptr; ptr = &i; pptr = &ptr; How does the above picture change if we run the rest code one by one? *ptr = 3; **pptr = 7; ptr = &j; **pptr = 9; *pptr = &i; *ptr = -2; 18
An Illustration int i = 5, j = 10; ptr j pptr i 10 3 int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *ptr Type int int int * int ** integer pointer pointer variable int de-reference of ptr Value 3 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i address of ptr 3 *ptr = -2; 19
An Illustration int i = 5, j = 10; ptr j pptr i 10 7 int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr **pptr Type int int int * int ** integer pointer pointer variable int de-reference of de-reference of pptr Value 7 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i address of ptr 7 *ptr = -2; 20
An Illustration int i = 5, j = 10; ptr j pptr i 10 7 int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *ptr Type int int int * int ** integer pointer pointer variable int de-reference of ptr Value 7 10 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of j address of ptr 10 *ptr = -2; 21
An Illustration int i = 5, j = 10; ptr j pptr i 9 7 int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr **pptr Type int int int * int ** integer pointer pointer variable int de-reference of de-reference of pptr Value 7 9 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of j address of ptr 9 *ptr = -2; 22
An Illustration int i = 5, j = 10; ptr j pptr i 9 7 int *ptr; int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *pptr Type int int int * int ** integer pointer pointer variable int * de-reference of pptr Value 7 9 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i address of ptr value of ptr (address of i) *ptr = -2; 23
An Illustration int i = 5, j = 10; ptr j pptr i 9 int *ptr; -2 int **pptr; ptr = &i; pptr = &ptr; Data Table Description *ptr = 3; **pptr = 7; Name i j ptr pptr *ptr Type int int int * int ** integer pointer pointer variable int de-reference of ptr Value -2 9 ptr = &j; integer variable integer variable integer pointer variable **pptr = 9; *pptr = &i; address of i address of ptr -2 *ptr = -2; 24
Pointer Arithmetic What s ptr + 1? The next memory location! What s ptr - 1? The previous memory location! What s ptr * 2 and ptr / 2? Invalid operations!!! 25
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? ? ? ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; *ptr = 6.0; ? ptr += 2; *ptr = 7.0; 26
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? ? ? ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[2] ? *ptr = 6.0; ptr += 2; *ptr = 7.0; 27
Pointer and Array (Exercise) How does the data table change if we run the rest code one by one? float a[4]; float *ptr; ptr = &(a[2]); Data Table Description *ptr = 3.14; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? ? ? ptr++; float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 9.0; ptr = ptr - 3; *ptr = 6.0; ptr += 2; address of a[2] ? *ptr = 7.0; 28
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? 3.14 ? ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[2] 3.14 *ptr = 6.0; ptr += 2; *ptr = 7.0; 29
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? 3.14 ? ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[3] ? *ptr = 6.0; ptr += 2; *ptr = 7.0; 30
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? 3.14 9.0 ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[3] 9.0 *ptr = 6.0; ptr += 2; *ptr = 7.0; 31
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value ? ? 3.14 9.0 ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[0] ? *ptr = 6.0; ptr += 2; *ptr = 7.0; 32
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value 6.0 ? 3.14 9.0 ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[0] 6.0 *ptr = 6.0; ptr += 2; *ptr = 7.0; 33
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value 6.0 ? 3.14 9.0 ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[2] 3.14 *ptr = 6.0; ptr += 2; *ptr = 7.0; 34
Pointer Arithmetic and Array float a[4]; Data Table Description float *ptr; Name a[0] a[1] a[2] a[3] ptr *ptr Type float float float float float * float pointer variable float de-reference of float pointer variable Value 6.0 ? 7.0 9.0 ptr = &(a[2]); float array element (variable) float array element (variable) float array element (variable) float array element (variable) *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; address of a[2] 7.0 *ptr = 6.0; ptr += 2; *ptr = 7.0; 35
Pointer Arithmetic and Array Type of a is float * a[2] *(a + 2) ptr = &(a[2]) ptr = &(*(a + 2)) ptr = a + 2 a is a memory address constant ptr is a pointer variable float a[4]; float *ptr; ptr = &(a[2]); *ptr = 3.14; ptr++; *ptr = 9.0; ptr = ptr - 3; *ptr = 6.0; ptr += 2; *ptr = 7.0; 36
More Pointer Arithmetic What if a is a double array? A doublemay occupy more memory slots! Given double *ptr = a; What s ptr + 1 then? Addr 1000 Content a[0]: 37.9 Addr 1001 Content Addr 1002 Content Addr 1003 Content 1004 1005 1006 1007 1008 a[1]: 1.23 1009 1010 1011 1012 1013 1014 1015 37
More Pointer Arithmetic Arithmetic operators + and auto-adjust the address offset According to the type of the pointer: 1000 + sizeof(double) = 1000 + 8 = 1008 Addr 1000 Content a[0]: 37.9 Addr 1001 Content Addr 1002 Content Addr 1003 Content 1004 1005 1006 1007 1008 a[1]: 1.23 1009 1010 1011 1012 1013 1014 1015 38
Summary A pointer stores the address (memory location) of another entity Address-of operator (&) gets the address of an entity De-reference operator (*) makes a reference to the referee of a pointer Pointer and array Pointer arithmetic 39