Exception Handling in Java: Understanding Errors and Exceptions
Exception handling in Java is crucial for dealing with errors and exceptions that can occur during program execution. Errors and exceptions are conditions that disrupt the normal flow of a program, and understanding their differences is key to effective error management. This chapter covers the concepts of errors, exceptions, exception hierarchy, classification of exceptions, built-in exceptions, user-defined exceptions, and more in the context of Java programming. Learn how to identify, handle, and differentiate between errors and exceptions to build robust and reliable Java applications.
Download Presentation
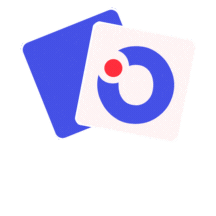
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Chapter 6 Exception Handling
Agenda Introduction Errors and Exception Exception Hierarchy Classification of Exceptions Built in Exceptions Exception Handling in Java User defined Exceptions ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Introduction An exception is a condition that cannot be resolved within the context of the operation that caused it. To throw an exception is to signal that such a condition has occurred. To catch an exception is to transfer control to an exception handling routine, altering the normal execution flow of the program. Because exceptions are outside the normal operation of a program the normal, default action is to write out an error message and terminate the offending process. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Questions What is an Error? What is the Difference between Error and Exception? ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Answers Error: An Error is a subclass of Throwable that indicates serious problems that a reasonable application should not try to catch. Errors can not be caught. Examples: Stackoverflow and underflow ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Answers Exception: The class Exception and its subclasses are a form of Throwable that indicates conditions that a reasonable application might want to catch. Exception can be caught using key words such as try and catch block. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exceptions Exceptions can occur at many levels: Hardware/operating system level Language level Program level Type conversion; illegal values, improper casts. Arithmetic exceptions; divide by 0, under/overflow. Bounds violations; illegal array indices. User defined exceptions. Memory access violations; stack over/underflow. Bad references; null pointers. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Example public class UnhandledException { public static void main(String[] args) { int num1 =0,num2 =5; int num3 = num2/num1; System.out.println(" Value = " +num3); } } ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Hierarchy ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Classification of Exceptions Exceptions can be classified as Built-in Exceptions These exceptions are define by Java and they reside in java.lang package . User-Defined Exceptions Exceptions defined by users. Examples of Exception: ArrayIndexOutOfBoundsExc eption NullPointerException IOException ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Built-in Exceptions Built-in Exceptions in Java Unchecked Exception: Checked Exception: They are run time errors that occur because of programming errors such as invalid arguments passed to a public method. Examples: ArrayIndexOutOfBoundsException NullPointerException The invalid conditions that occur in java program due to invalid user input, database problems etc. Examples: IOException SQLException ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Checked Exception Exception Name Description ClassNotFoundException Class not found Attempt to create an object of an abstract class or interface. Access to a class is denied. InstantiationException IllegealAccessException NoSuchMethodException A requested method does not exist. NoSuchFieldException A requested field does not exist. One thread has been interrupted by another thread. Attempt to clone an object that does not implement Cloneable interface. InterruptedException CloneNotSupportedException ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Unchecked Exception Exception Name Description Arithmetic error, such as divide by zero. Array created with a negative size. ArithmeticException NegativeArraySizeException NullPointerException Invalid use of a null reference. Illegeal argument used to invoke a method. Invalid class cast Arithmetic error, such as divide by zero. Array created with a negative size. IllegealArgumentException ClassCastException ArithmeticException NegativeArraySizeException ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Handling exceptions General Example Snippet of a pseudocode follows: IF B IS ZERO GO TO ERROR C=A/B PRINT C GO TO EXIT Block that handles error ERROR: DISPLAY DIVISION BY ZERO EXIT: END ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Handling in Java Exceptions in Java can be handled by five keywords Catch Throw Try Throws Finally ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Handling in Java Contd., used with the code that might throw an exception. TRY This statement is used to specify the exception to catch and the code to execute if the specified exception is thrown. CATCH is used to define a block of code that we always want to execute, regardless of whether an exception was caught or not. FINALLY THROW Typically used for throwing user-defined exceptions THROWS Exception Lists the types of exceptions a method can throw, so that the callers of the method can guard themselves against the exception ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Handling Mechanism The basic concepts of exception handling are throwing an exception and catching it. Try Block Exception object creator Throws exception object Statement that causes an exception catch Block Exception Handler Statement that handles the exception ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Exception Handling syntax try { } statements catch (Exception class object) { statements } finally { //Close file handles //Free the resources } ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Try and catch public class TryCatchDemo public static void main(String [] args) int x,y; try { x = 0; y = 10/x; System.out.println( Now What ??? ); } catch(ArithmeticException e) System.out.println( Division by zero ); } System.out.println( Hi I am back !!! ); } } { { { TryCatchDemo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Using multiple Catch Statements try catch(exception-class1 object) { { //blocks of code //blocks of code } } catch(exception-class1 object) { //blocks of code } A single try block can have many catch blocks. Multiple catch statements are used when a try block has statements that raise different types of exceptions. MultipleCatchBlockDemo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Handling the Unreachable Code Problem If in an program the first catch block contains the Exception class object then subsequent catch blocks are never used. The excetion class being the super class, java gives a compiler error stating that the subsequent catch blocks have not been reached. This phenomenon is termed as unreachable code problem . UnreachableCodeDemo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Finally Block Ensures that all cleanup work is taken care of when an exception occurs Used in conjunction with a try block Guaranteed to run whether or not an exception occurs Syntax finally { // Blocks of code} finally No exception try block catch block finally Exception Finally Demo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Rules for Try-Catch-Finally For each try block there can be one or more catch block but only one finally block. A finally block cannot execute without a try block. Try block can be nested with catch blocks. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Throw and Throws Keyword "throws" declares that your method is capable of throwing an exception. "throw" actually does the work , of throwing the exception. Example : public void doSomething() throws ApplicationException { try{ } catch(Exception e){ // catch all excpetions here throw ApplicationException("An error occurred while trying connect to DB"); } } ThrowsAndThrowKeyWordDemo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
User Defined Exceptions UserDefined Exceptions are any subcalss of Exception which is defined by a user to define their own exceptional condition. Any violation of Business rules of an application can be handled using User Defined Exception. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
User Defined Exceptions Contd., For example, If the person is in the business of selling motorcycles and need to validate an order which has been placed by a customer, creating a user- defined exception in the Java programming language can be done by a creating a new class which can be named TooManyBikesException. if someone tries to place an order for more motorcycles than you can ship, you can simply throw a user-defined exception. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
User Defined Exception Contd., Rules for writing UserDefinedException Class: a. Userdefined Exception class has to exend an Exception class . b. When an business rule is violated, a userdefined exception object has to be created and thrown using throw key word. c. Any userdefined Exception thrown using the throw key word has to be handled in respective catch block of userdefined Exception. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
User Defined Exception Contd., Example: Class UserDefinedException extends Exception { String getMessage() { Return logical message related to error raised ; } } UserDefinedExceptionDemo ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Summary An exception is a run time error that can be defined as an abnormal event that occurs during the execution of a program and disrupts the normal flow of instructions. In Java, the Throwable class is the superclass of all the exception classes. The Exception class and the Error class are two direct subclasses of the Throwable class. The built-in exceptions in Java are divided into two types on the basis of the conditions where the exception is raised: Checked Exceptions or Compiler-enforced Exceptions Unchecked exceptions or Runtime Exceptions ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Summary Contd., You can implement exception handling in your program by using the following keywords: try catch throw throws Finally You use multiple catch blocks to throw more than one type of exception. ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
Summary Contd., The finally clause is used to execute the statements that need to be executed whether or not an exception has been thrown. The throw statement causes termination of the normal flow of control of the Java code and stops the execution of subsequent statements after the throw statement. The throwsclause is used by a method to specify the types of exceptions the method throws. You can create your own exception classes to handle the situations specific to an application ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII
References http://java.sun.com/docs/books/tutorial/essential/exceptions/index.html http://www.javabeginner.com/java-exception-handling.htm http://mindprod.com/jgloss/exception.html http://www.tutorialhero.com/tutorial-71-java_exceptions.php ICT Academy of Tamil Nadu A Consortium of Govt of India, Govt of Tamil Nadu and CII