Exception Handling in Java: Basics, Examples, and Importance
Understanding the concept of exception handling in Java, including what exceptions are, the difference between errors and exceptions, reasons for exceptions, how to handle them, and the advantages of exception handling. This topic covers the basics of handling runtime errors in Java programming and the importance of maintaining the normal flow of applications.
Download Presentation
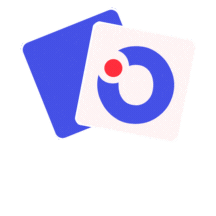
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Dr.Umayal Ramanathan College for Women Affiliated to Alagappa University, Karaikudi Accredited with B+ Grade by NAAC Department of Information TechnologY Java programming 7BIT2C1 Unit IV- Exception Handling Dr.L.Visalatchi Associate Professor Department of IT Department of INformation Technology,URCW
Exception Handling What is an Exception? Difference between error and exception? How to do Exception handling? - Keywords Important Exceptions. Exception Hierarchy Example program Department of INformation Technology,URCW
What is an Exception in java? Exception is an abnormal condition. In Java, an exception is an event that disrupts the normal flow of the program. When an exception occurs program execution gets terminated. In such cases we get a system generated error message. Department of INformation Technology,URCW
Why an exception occurs? There can be several reasons that can cause a program to throw exception. For example: Opening a non-existing file in your program, Network connection problem, bad input data provided by user etc. Department of INformation Technology,URCW
Why do we handle Exception Exception Handling is a mechanism to handle runtime errors such as ClassNotFoundException, IOException, SQLException, RemoteException, etc. It is handled as an object which is thrown at runtime. Advantage of Exception Handling The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application; that is why we need to handle exceptions. Department of INformation Technology,URCW
Difference between Error and Exception Error: Error is irrecoverable. Some example of errors are OutOfMemoryError, VirtualMachineError, AssertionError etc. Exception: Exceptions are recoverable and could be handled. Department of INformation Technology,URCW
Keywords in handling Exception try: The "try" keyword is used to specify a block where we should exception code. It means we can't use try block alone. The try block must be followed by either catch or finally. catch: The "catch" block is used to handle the exception. It must be by try block which means we can't use catch block block later. finally:The "finally" block is used to execute the necessary code of program. It is executed whether an exception is handled or throw: The "throw" keyword is used to throw an exception. throws: The "throws" keyword is used to declare exceptions. It specifies that there may occur an exception in the method. It doesn't throw an exception. It is always used with method signature. place an preceded alone. It can be followed by finally the not. Department of INformation Technology,URCW
Steps in Exception handling Syntax of try catch in java try { //statements that may cause an exception } catch (exception(type) e(object)) { //error handling code } Department of INformation Technology,URCW
public class JavaExceptionExample{ public static void main(String args[]) { try{ //codethat may raise exception int data=100/0; }catch(ArithmeticException e) { System.out.println(e); } //rest code of the program System.out.println("restof the code..."); } } Output: Exception in thread main java.lang.ArithmeticException:/ by zero rest of the code... Department of INformation Technology,URCW
Multiple catch blocks in Java A single try block can have any number of catch blocks. A generic catch block can handle all the exceptions. ArrayIndexOutOfBoundsException ArithmeticException ,NullPointerException or any other type of exception, this handles all of them. Department of INformation Technology,URCW
Flow chart for multiple catch Department of INformation Technology,URCW
class Example2{ public static void main(String args[]){ try{ int a[]=new int[7]; a[4]=30/0; System.out.println("First print statement in try block"); } catch(ArithmeticException e){ System.out.println("Warning:ArithmeticException"); } catch(ArrayIndexOutOfBoundsException e) { System.out.println("Warning:ArrayIndexOutOfBoundsExceptio n"); } catch(Exception e){ System.out.println("Warning: Some Other exception"); } System.out.println("Out of try-catch block..."); } } Output: Warning: ArithmeticException Out of try-catch block.. Department of INformation Technology,URCW
Java Nested try block In Java, using a try block inside another try block is permitted. It is called as nested try block. For example, the inner try block can be used to handle ArrayIndexOutOfBoundsException while the outer try block can handle the ArithemeticException (division by zero). Why use nested try block Sometimes a situation may arise where a part of a block may cause one error and the entire block itself may cause another error. In such cases, exception handlers have to be nested. Department of INformation Technology,URCW
//main try block try { statement 1; statement 2; //try catch block within another try block try { statement 3; statement 4; //try catch block within nested try block try { statement 5; statement 6; } catch(Exception e2) { //exception message } } catch(Exception e1) { //exception message } } //catch block of parent (outer) try block catch(Exception e3) { //exception message } .... Department of INformation Technology,URCW
public class NestedTryBlock{ public static void main(String args[]){ try{ try{ System.out.println("going to divide by 0"); int b =39/0; } catch(ArithmeticException e) { System.out.println(e); } //inner try block 2 try{ int a[]=new int[5]; a[5]=4; } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); } System.out.println("other statement"); } catch(Exception e) { System.out.println("handled the exception (outer catch)"); } System.out.println("normal flow.."); } } Department of INformation Technology,URCW
Output Department of INformation Technology,URCW
Java finally block Java finally block is a block used to execute important code such as closing the connection, etc. Java finally block is always executed whether an exception is handled or not. Therefore, it contains all the necessary statements that need to be printed regardless of the exception occurs or not. Department of INformation Technology,URCW
Why use Java finally block? finally block in Java can be used to put "cleanup" code such as closing a file, closing connection, etc. The important statements to be printed can be placed in the finally block Department of INformation Technology,URCW
Flowchart of finally block Department of INformation Technology,URCW
Java throw keyword The Java throw keyword is used to throw an exception explicitly. We specify the exception object which is to be thrown. The Exception has some message with it that provides the error description. These exceptions may be related to user inputs, server, etc. We can throw either checked or unchecked exceptions in Java by throw keyword. It is mainly used to throw a custom exception. Department of INformation Technology,URCW
public class TestThrow1 { //function to check if person is eligible to vote or not public static void validate(int age) { if(age<18) { //throw Arithmetic exception if not eligible to vote throw new ArithmeticException("Person is not eligible to vote"); } else { System.out.println("Person is eligible to vote!!"); } } //main method public static void main(String args[]){ //calling the function validate(13); System.out.println("rest of the code..."); } } Department of INformation Technology,URCW
Output Department of INformation Technology,URCW
Java throws keyword The throw and throws is the concept of exception handling where the throw keyword throw the exception explicitly from a method or a block of code whereas the throws keyword is used in signature of the method. Department of INformation Technology,URCW
Java throws Example public class TestThrows { //defining a method public static int divideNum(intm, int n) throws ArithmeticException{ int div = m / n; return div; } //main method public static void main(String[] args){ TestThrows obj = newTestThrows(); try { System.out.println(obj.divideNum(45,0)); } catch (ArithmeticException e){ System.out.println("\nNumber cannot be dividedby 0"); } System.out.println("Restof the code.."); } } Department of INformation Technology,URCW
Important Exceptions ArithmeticException It is thrown when an exceptional condition has occurred in an arithmetic operation. ArrayIndexOutOfBoundsException It is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array. ClassNotFoundException This Exception is raised when we try to access a class whose definition is not found FileNotFoundException This Exception is raised when a file is not accessible or does not open. IOException It is thrown when an input-output operation failed or interrupted InterruptedException It is thrown when a thread is waiting, sleeping, or doing some processing, and it is interrupted. NoSuchFieldException It is thrown when a class does not contain the field (or variable) specified NoSuchMethodException It is thrown when accessing a method which is not found. NullPointerException This exception is raised when referring to the members of a null object. Null represents nothing NumberFormatException This exception is raised when a method could not convert a string into a numeric format. RuntimeException This represents any exception which occurs during runtime. StringIndexOutOfBoundsException It is thrown by String class methods to indicate that an index is either negative or greater than the size of the string Department of INformation Technology,URCW
Exception Hierarchy Department of INformation Technology,URCW
ArrayIndexOutOfBound Exception // Java program to demonstrate ArrayIndexOutOfBoundException class ArrayIndexOutOfBound_Demo { public static void main(String args[]) { try{ int a[] = new int[5]; a[6] = 9; // accessing 7th element in an array of } catch(ArrayIndexOutOfBoundsException e){ System.out.println ("Array Index is Out Of Bounds"); } } } Department of INformation Technology,URCW // size 5