BCA 504 : Java Programming
Java provides special features such as short-circuit logical operators, assignment operators, bitwise and shift operators, and the ternary operator. These features enhance efficiency and functionality in code writing. Short-circuit operators help save time by evaluating operands only when necessary, assignment operators simplify assigning values to variables, bitwise operators manipulate data at the bit level, and the ternary operator offers a concise way to construct conditional expressions.
Download Presentation
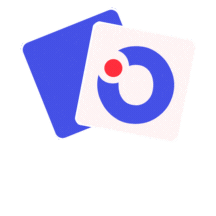
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
BCA 504 : Java Programming
Short-Circuit Logical Operators Java supplies special short-circuit versions of its AND and OR logical operators that can be used to produce more efficient code In an AND operation, if the first operand is false, the outcome is false no matter what value the second operand has. In an OR operation, if the first operand is true, the outcome of the operation is true no matter what the value of the second operand. Thus, in these two cases there is no need to evaluate the second operand. By not evaluating the second operand, time is saved and more efficient code is produced. The short-circuit AND operator is &&, and the short-circuit OR operator is ||. Their normal counterparts are & and |. The only difference between the normal and short-circuit versions is that the normal operands will always evaluate each operand, but short-circuit versions will evaluate the second operand only when necessary.
Here is a program that demonstrates the short-circuit AND operator. //Demonstrates the short-circuit operators. class Scops { static void main (String args[ ]) { int n, d, q; n=10; d=2; if (d !=0 && (n % d) = = 0) System.out.println(d + is a factor of + n); d=0; //now, set d to zero//Since d is zero, the second operand is not evaluated. if (d !=0 && ( n % d) = = 0) system.out.println(d + is a factor of + n); /* Now, try same thing without short-circuit operator. This will cause a divide-by-zero error. */ if (d != 0 & (n % d) = = 0) System.out.println( d + is a factor of +n); }
Assignment Operators Assignment operators are used to assign the value of an expression to a variable. We have seen the usual assignment operator = . In addition, Java has a set of 'shorthand' assignment operators which are used in the form v op= exp; Where v is a variable, exp is an expression and op is a Java binary operator. The operator op = is known as the shorthand assignment operator. The assignment statement v op= exp; is equivalent to v = v op (exp) with v accessed only once. Statement with simple assignment operator a= a+1 a= a-1 a= a* (n+1) a= a/(n+1) a= a%b
Bitwise and Shift Operator Java has a distinction of supporting special operators known as bitwise operators for manipulation of data at values of bit level. These operators are used for testing the bits, or shifting them to the right or left. Bitwise operators may not be applied to float or double. Operator Meaning & Bitwise AND | Bitwise OR ^ Bitwise exclusive OR ` One s Complement << Shift left >> Shift right >>> Shift right with zero fill
Ternary Operator: The ?: operator The character pair ?: is a ternary operator available in Java. This operator is used to construct conditional expressions of the form exp1 ? exp2 : exp3 Where expl, exp2, and exp3 are expressions. The operator?: works as follows: expl is evaluated first. If it is nonzero (true), then the expression exp2 is evaluated and becomes the value of the conditional expression. If expl is false, exp3 is evaluated and its value becomes the value of the conditional expression. Note that only one of the expressions (either exp2 or exp3) is evaluated. a = 10; b = 15; max = (a > b) ? a : b; In this example, x will be assigned the value of b.
Shorthand Assignment Java provides special shorthand assignment operators that simplify the coding of certain assignment statements. Let s begin with an example. The assignment statement shown here x= x + 10 can be written using java short-hand as x+=10 Statement with shorthand operator a +=1 a -=1 a*= n+1 a/= n=1 a%=b
Type Conversion in Assignments When a data type is converted into another type is called type conversion. In programming, it is common to assign one type of variable to another. For example, you might want to assign an int value to a float variable, as shown here: Ex: int i; float f; i=10; f=i //assigns an int to a float When compatible types are mixed in an assignment, the value of the right side is automatically converted to the type of the left side. However, because of Java s strict type checking, not all types are compatible. Conversion can be of two types 1) Implicit Conversion 2) Explicit Conversion
Implicit Conversion: In implicit conversion compiler will automatically change one type of data into another. It will happen if both type are compatible and target is larger than source type. byte short int long float double widening Eg:- short a = 2000; int b; b = a; Explicit Conversion: When we want to convert a value having larger type to smaller type. In this case casting needs to be performed explicitly. double float long int short byte narrowing Eg:- int a = 10; byte b = (int) a; Here size of source type int is 32 bits and size of destination type byte is 8 bits. Here source type is larger than destination type such conversion is known as narrowing conversion.
Operator Precedence Operator Description . ( ) [ ] Array element reference - ++ -- ! (type ) * / % Modulus + - Subtraction << >> >>> Right shift with zero fill < <= > >= instance of == != Inequality & Bitwise AND Associativity Left to right Rank 1 Member selection Function call Each operator in Java has a precedence associated with it.This precedence is used to determine how an expression involving more than one operator is evaluated. Unary Minus Increment Decrement Logical negation Ones complement Casting Right to Left 2 The operators at the higher level of precedence are evaluated first. The operators of the same precedence are evaluated either from left to right or from right to left, depending on the level. This is known as the associativity property of an operator. Multiplication Division Left to right 3 Addition Left to right 4 Left to right 5 Left shift Right shift Less than Less than or equal to Greater than Greater than or equal to Type comparison Left to right 6 Equality Left to right 7 Left to right 8 ^ Bitwise XOR Left to right 9 | Bitwise OR Left to right 10 && Logical AND Left to right 11 || Logical OR Left to right 12
Expressions Operators, variables, and literals are constituents of expressions. Expressions are evaluated using an assignment statement of the form variable=expression; Variable is any valid Java variable name. When the statement is encountered, the expression is evaluated first and the result then replaces the previous value of the variable on the left-hand side. All variables used in the expression must be assigned values before evaluation is attempted. Examples of evaluation statements are x= a*b-c; y = b/c*a; z= a-b/c+d; Type Conversion in Expressions: Within an expression, it is possible to mix two or more different types of data as long as they are compatible with each other. For example, you can mix short and long within an expression because they are both numeric types. When different types of data are mixed within an expression, they are all converted to the same type