Enhancing Programming Skills: Iteration and Problem Solving in CS Course
Central concepts of iteration and problem-solving techniques in a Computer Science course are explored and valued by students in Chapter 6. Feedback highlights the effectiveness of TA support, engaging teaching methods, and practical exercises. Suggestions for improvement include better alignment of chapters and assignments, clearer instructions for beginners, and more hands-on collaborative activities. Techniques like loops, recursion, and stack memory are taught through practical examples, emphasizing the importance of avoiding repetitive code. The Problem Specification task involves calculating the snow volume based on the radius of snowballs, encouraging students to write efficient code and extract functions for reusability.
Download Presentation
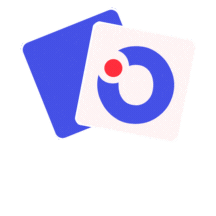
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Chapter 6 Paul Bodily
Midterm Eval Feedback What aspects of this course are most useful or valuable? "Discord and the availability of TA's" "It find it helpful that the instructor asks the students questions; it makes the course more engaging." "Doing small projects that are similar to the exercises." "I love this class, everything about it is very useful, valuable, and there are plenty of resources provided so I never have to feel behind." "The exercises (even though they are hard) and the textbook as well as the in class coding examples." "I really enjoy the textbook. I wish it explained some terms early on before reading the first chapter as someone who has never seen code before it was a little disorienting." "The way Dr Paul present the coding in class."
Midterm Eval Feedback How would you improve this course? Other comments? "Maybe if the chapter discussed and the assignment due the same week would synchronize." "It seems like it is expected that we have prior coding experience." "More time working with others in person." "Instead of having a big exercises due every week, maybe space them out with simpler ones. For example, the vending machine and fly walk seems like a lot back to back." "Maybe in a computer lab setting. Also the projects are not written with zero pre existing knowledge in mind. Even the directions are written as if you already knew what you should be doing." "I'd like to see more tactile use of objects to explain things like the stacks and the plates." "Easier exercises that aren't time consuming."
Iteration Central concept: avoid repetitive code Helps avoid bugs Saves time and space Iteration = Doing (nearly) the same thing over and over We did this with recursion. Now we show how to using loops
Example #include <iostream> using namespace std; 4 3 2 1 Blastoff! void countdown (int n) { while (n > 0) { cout << n << endl; n = n - 1; } cout << "Blastoff!" << endl; } countdown n: 9 n: 0 n: 1 n: 2 n: 3 n: 4 int main() { int start = 4; countdown (start); } main start: 4 stack (memory) multiple assignment
Problem Spec: Snowman Write a program that prompts the user for a number n representing the number of balls Then compute the volume of snow needed for that many balls where the ith ball has a radius of i Example output: How many snowballs? 4 radius volume 1 in 4.1887902048 in^3 2 in 33.5103216383 in^3 3 in 113.09733552923 in^3 4 in 268.08257310633 in^3 Total volume: 418.8790204786 in^3 When complete, extract a function for calculating volume given the radius Then change the program to allow the user to specify the n radii
TAPPS: Table of squares Write a program that prints the integers 0 to 10 each with their square Example output: 0^2= 0 1^2= 1 2^2= 4 3^2= 9 4^2= 16 5^2= 25 6^2= 36 7^2= 49 8^2= 64 9^2= 81 10^2= 100
Anon question Hi, I'm really struggling with PrarieLearn. I can get almost all of my codes to work in CLion and when I convert them over they no longer function. Any ideas on what I should do?
Print a chess board X O X O X O X O O X O X O X O X X O X O X O X O O X O X O X O X X O X O X O X O O X O X O X O X X O X O X O X O O X O X O X O X
Print Ascii Football Table ------------------------------------------- | | | DUCKS | | | ------------------------------------------- - - - - ------------------------------------------- - - - - 10 ------------------------------------- 10 - - - - ------------------------------------------- With arbitrary dimensions _ _ _ _ _ _ _ _ _ _ _ _