Advanced Programming of Web Applications - PHP
Explore advanced topics in PHP programming such as Traits, Generators, Reflection, and Namespaces. Learn about code reuse mechanisms, custom iterators, dynamic inspection of types, and encapsulation of identifiers using namespaces.
Download Presentation
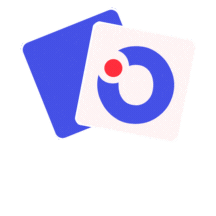
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
PHP NSWI153 NSWI153 - - Advanced Advanced Programming of Web Applications Programming of Web Applications 202 2023/2024 3/2024 Petr Petr koda koda https://github.com/skodapetr https://github.com/skodapetr https://www.ksi.mff.cuni.cz https://www.ksi.mff.cuni.cz This work is licensed under a Creative Commons Attribution 4.0 International License.
Outline PHP 2
Traits Class-like mechanism for code reuse / horizontal composition of behavior, like Mixins. Trait Special class that cannot be instantiated May contain both member variables and methods It can be added to regular classes trait OmniSaluter { public function helloWorld() { echo 'Hello World'; } } class myClass { use OmniSaluter; ... since 2012 3
Generators Detached custom iterators The foreach construct is powerful, but it iterates over structures (arrays and objects) Custom iterator can be built (Iterator interface), both memory demanding and tedious Generator is a function that yields values It can be used in foreach construct function range($from, $to, $step) { for ($i = $from; $i < $to; $i += $step) yield $i; } foreach ( range(1,10,2) as $value) ... since 2013 4
Reflection A mechanism implemented in (dynamic) languages to inspect types, objects, classes, etc. at runtime PHP holds a specific reflector class for each entity: ReflectionType, ReflectionFunction, ReflectionClass, ReflectionObject, ReflectionProperty, ReflectionParameter API also provides access to doc comments /** */ /** * @Type */ class MyType { ... #[AttrCommas('foo'), AttrCommas('foo )] class MyType { ... since 2022 5
Namespaces Another way how to encapsulate space of identifiers Affect classes, traits, interfaces, functions, constants , NOT global variables Similar to directories and files namespace App\Presenters; use App\Model\Entity\User; use DateTime; class UserDetailPresenter { private function loginExpired(User $user, DateTime $ts): bool ... } since 2011 6
Error handling Types: User errors Temporary/Soft Errors Hard Errors Error Levels: E_ERROR, E_WARNING, E_NOTIVE, E_USER_xxx, E_STRICT Controlled in php.ini or by error_reporting() trigger_error() Error Control (Silence) Operator try { ... throw new Exception('Error '); } catch (MyException $ex) { ... My exception handler ... } catch (Exception $ex) { ... Generic exception handler ... } Exceptions Uncaught exception causes Fatal Error Destructors should not throw exceptions Throwing-catching process is somewhat slow 7
8 DEMO https://github.com/linkedpipes/etl/issues/544 2018 - 2021
PHP 8.0 Named arguments Order independent and optional. echo match (8.0) { '8.0' => "Oh no!", 8.0 => "This is what I expected", }; //> This is what I expected Attributes Instead of PHPDoc annotations. Constructor property promotion $country = $session?->user?->getAddress()?->country; Match expression Nullsafe operator class PostsController { #[Route("/api/posts/{id}", methods: ["GET"])] public function get($id) { /* ... */ } } since 2020 9
PHP 8.1 Enumerations Readonly properties $arrayA = ['a' => 1]; $arrayB = ['b' => 2]; First-class Callable Syntax We can get reference to a class function. $result = [ 'a' => 0, ...$arrayA, ...$arrayB ]; Improved type hinting never return type Final class constants // ['a' => 1, 'b' => 2] Array unpacking support for string-keyed arrays since 2021 10
PHP 8.3 PHP 8.2 Deprecate dynamic properties interface I { const string PHP = 'PHP 8.3'; } PHP 8.3 since 2023 Typed class constants class MyClass extends Parent { #[\Override] protected function action() { // } } New #[\Override] attribute New json_validate() function since 2023 11
Data structures Data Structures SplDoublyLinkedList SplQueue SplPriorityQueue SplFixedArray SplObjectStorage Functions spl_object_hash() spl_object_id() Unique for given object existence. Iterators ArrayIterator DirectoryIterator GlobIterator 13
Files Low Level C-like API fopen(), fread(), fwrite(), fclose(), Newer OOP API SplFileObject, SplTempFileObject File Properties (Size, Type, Creation Time, ) filesize(), filetype(), filectime(), stat(), lstat(), POSIX Access Rights (rwx) is_file(), is_readable(), is_writeable(), Directories opendir(), readdir(), closedir(), scandir(), dir(), glob() dirname(), basename(), realpath() 14
Files Compression ZIP Binary data Images (GD2, Cairo, Gmagic, ImageMagick, ) 15
Serialization and Deserialization serialize(), unserialize() Converts (almost) any type to string (and back) json_decode(), json_encode(), json_last_error(), json_last_error_msg() JsonSerializable interface with jsonSerialize() DOMDocument, , DOMXPath, XSLTProcessor, , SimpleXMLElement XML (SAX, DOM, XPath, XSLT) fgetcsv() , fputcsv() Uses file handlers from fopen() YAML 16
17 PHP Frameworks and Libraries
Framework Interop Group PSR-1, PSR-12 Coding style guidelines use Monolog\Logger; use Monolog\Handler\StreamHandler; PSR-3 Logger interface PSR-4 Autoloading (classes) // create a log channel $log = new Logger('name'); $log->pushHandler( new StreamHandler( 'path/to/your.log , Logger::WARNING)); PSR-6 Caching Interface PSR-7 HTTP message interface PSR-11 Container interface (DI) // Add records to the log $log->warning('Foo'); $log->error('Bar'); PSR-5 PHPDoc Standard, 19 PHPDoc tags 18
Takeaway Language features Standard (PHP) library PHP Framework Interop Group