Understanding Swift Generics and Data Structures
In this content, we explore Swift generics through examples of swapping integers, strings, and doubles using generic functions. We also delve into implementing a generic type for a queue data structure in Swift. The concepts are illustrated with code snippets and visual aids, making it easier to grasp the concepts.
Download Presentation
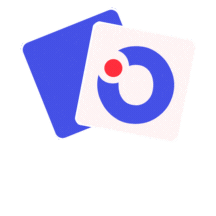
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
17.1.1 // swap two integer numbers func swapInts(a: inout Int, b: inout Int) { let temp = a a = b b = temp }
part1 // swap using generic function func swapData<T>(a: inout T, b: inout T) { let temp = a a = b b = temp } var oneString = "Hello" var anotherString = "Swift" print("Before swapped: ") print("oneInt = \(oneString), anotherInt = \(anotherString) ") swapData(a: &oneString, b: &anotherString) print("After swapped: ") print("oneInt = \(oneString), anotherInt = \(anotherString) ") var oneInt = 100 var anotherInt = 200 print("Before swapped: ") print("oneInt = \(oneInt), anotherInt = \(anotherInt) ") swapData(a: &oneInt, b: &anotherInt) print("After swapped: ") print("oneInt = \(oneInt), anotherInt = \(anotherInt) ")
part2 var oneDouble = 123.456 var anotherDouble = 654.321 print("Before swapped: ") print("oneInt = \(oneDouble), anotherInt = \(anotherDouble) ") swapData(a: &oneDouble, b: &anotherDouble) print("After swapped: ") print("oneInt = \(oneDouble), anotherInt = \(anotherDouble) ")
Before swapped: oneInt = 100, anotherInt = 200 After swapped: oneInt = 200, anotherInt = 100 Before swapped: oneString = Hello, anotherString = Swift After swapped: oneString = Swift, anotherString = Hello Before swapped: oneInt = 123.456, anotherInt = 654.321 After swapped: oneInt = 654.321, anotherInt = 123.456
TT <T> T
17.1.2 (queue) (stack)
part1 // generic type struct Queue<T> { var items = [T]() mutating func insert(item: T) { items.append(item) } mutating func delete() -> T { return items.remove(at: 0) } } var queueOfInt = Queue<Int>() queueOfInt.insert(item: 100) queueOfInt.insert(item: 200) queueOfInt.insert(item: 300) queueOfInt.insert(item: 400) queueOfInt.insert(item: 500) print("The integer queue has following elements: ") for i in queueOfInt.items { print("\(i) ", terminator: "") } print("")
part2 queueOfInt.delete() print("After delete 100, the queue has following elements: ") for i in queueOfInt.items { print("\(i) ", terminator: "") } print("") queueOfInt.insert(item: 600) print("After insert 600, the queue has following elements: ") for i in queueOfInt.items { print("\(i) ", terminator: "") } print("\n")
The integer queue has following elements: 100 200 300 400 500 After delete 100, the queue has following elements: 200 300 400 500 After insert 600, the queue has following elements: 200 300 400 500 600
17.2 (type constraint)
17.2.1 func searchData(array: [String], valueToSearch: String) ->Int? { for (index, value) in array.enumerated() { if value == valueToSearch { return index } } return nil }
part1 func searchData<T: Equatable>(array: [T], valueToSearch : T) -> Int? { for (index, value) in array.enumerated() { if value == valueToSearch { return index } } return nil } print("The index of Kiwi is \(found)") let found2 = searchData(array: arrayofStrings, valueToSearch: "Pineapple") print("The index of Pineapple is \(found2)") let arrayOfInt = [11, 22, 33, 44, 55] let found3 = searchData(array: arrayOfInt, valueToSearch: 55) print("\nThe index of 55 is \(found3)") let found4 = searchData(array: arrayOfInt, valueToSearch: 66) print("The index of 66 is \(found4)") let arrayofStrings = ["Apple", "Guava", "Banana", "Kiwi", "Orange"] let found = searchData(array: arrayofStrings, valueToSearch: "Kiwi") let arrayOfDouble = [11.1, 22.2, 33.3, 44.4, 55.5] let found5 = searchData(array: arrayOfDouble, valueToSearch: 22.2)
part2 print("\nThe index of 22.2 is \(found5)") let found6 = searchData(array: arrayOfDouble, valueToSearch: 66.6) print("The index of 66.6 is \(found6)")
The index of Kiwi is Optional(3) The index of Pineapple is nil The index of 55 is Optional(4) The index of 66 is nil The index of 22.2 is Optional(1) The index of 66.6 is nil
//sorting integer numbers var arrOfInt = [10, 30, 5, 7, 2, 8, 18, 12] print("Before sorted: ") for i in arrOfInt { print("\(i) ", terminator: "") } arr[j] = arr[j+1] arr[j+1] = temp } } if flag == false { break } } func bubbleSort(arr: inout [Int]) { var flag: Bool for i in 0..<arr.count-1 { flag = false for j in 0..<arr.count-i-1 { if arr[j] > arr[j+1] { } bubbleSort(arr: &arrOfInt) print("\n\nAfter sorted: ") for j in arrOfInt { print("\(j) ", terminator: "") } print("") flag = true let temp = arr[j]
Before sorted: 10 30 5 7 2 8 18 12 After sorted: 2 5 7 8 10 12 18 30
17.3 associatedtype Swift 3 typealias
part1 protocol ExtraInformation { associatedtype ItemType var count: Int {get} subscript(i: Int) -> ItemType {get} } //comformance to the ExtraInformation Protocol typealias ItemType = T var count: Int { return items.count } subscript(i: Int) -> T { return items[i] } } struct QueueType<T>: ExtraInformation { var items = [T]() mutating func insert(item: T) { items.append(item) } mutating func delete() { items.remove(at: 0) }
part2 var queueOfData = QueueType<Int>() queueOfData.insert(item: 100) queueOfData.insert(item: 200) queueOfData.insert(item: 300) queueOfData.insert(item: 400) queueOfData.insert(item: 500) print(" \(queueOfData.count) ") for i in queueOfData.items { print("\(i) ", terminator: "") } print("\n") for i in queueOfData.items { print("\(i) ", terminator: "") } print("\n") queueOfData.insert(item: 600) print(" 600 \(queueOfData.count) ") for i in queueOfData.items { print("\(i) ", terminator: "") } print("\n") queueOfData.delete() print(" 100 \(queueOfData.count) ") print("queueOfData[2] = \(queueOfData[2])") print("")
5 100 200 300 400 500 100 4 200 300 400 500 600 5 200 300 400 500 600 queueOfData[2] = 400