Understanding Functions in Modular Programming
Functions in modular programming allow for hierarchical decomposition of problems into smaller tasks, with interfaces defining input parameters and output. Each function operates independently, following a specific structure for headers, parameters, and return statements. Proper function prototyping and handling of parameters are essential for the correct execution of code. It is crucial to define functions before calling them to ensure the correct number and types of arguments are used.
Uploaded on Sep 21, 2024 | 0 Views
Download Presentation
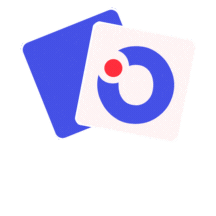
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Chapter 6 - Functions modular programming general function format defaults passing arguments function scope function prototyping variable # of parameters recursion
Modular programming Hierarchical decomposition: dividing problem into a number of functions, each performing a specific (single) task. Specify (and minimize) interfaces: define input parameters, output, data types, etc. Write functions based on interface, but otherwise independent of the details of all other functions!
Function general form Function header Function body Return statement (optional) Note: functions have the same scope and privacy rules as automatic variables!
Function header type function_name (type param1, ..., type paramn) the first type indicates the data type of the returned value. int is assumed (by default) if no type is specified. functions which do not return a value should be specified as void function_name is the name by which the function is invoked (called).
Function header - continued input arguments are defined in the parameter list following the function name. argument list is enclosed in parentheses. arguments are separated by commas. data types are given before argument name. if no input arguments required, use (void) arguments are not redefined later in function declaration statements. function header does not end with semicolon!
Function body body of statements enclosed in parentheses: { statement_1; ... statement_m; return value; }
return statement normally the last statement in function. optional, but preferred. function will terminate at right brace } if no return statement is used. value is the value returned to the calling function. Only one value can be returned. It can be an expression and will be implicitly cast, if necessary. A value cannot be given if the function type is void. It is not an error if the calling function ignores the returned value!
Function requirements A function must be defined (or prototyped) before it is invoked (called). the compiler checks to make sure the correct number of arguments is used. the compiler implicitly casts any mismatched argument data types, generates error message if casting can t be done (e.g. if an array is given instead of a scalar).
More on functions functions can be embedded as deeply as you like: printf( %f ,atof(gets(buff))) Stub functions are o.k. and useful in development: int new_function(float x, double y) { }
Arguments In general, programming languages can pass arguments in one of two ways: pass by reference: the called function gets direct access to the variable and can change its value in the calling function. pass by value (or copy): the called function gets only a copy of the variable and CANNOT change its value in the calling function.
Argument passing in C In C, arguments are ALWAYS passed by value!!! What can one do if one needs to modify the arguments in the function (e.g. scanf) ??? If the argument which is passed (by copy) is the address of a variable, then the variable can be modified. This is called simulated call by reference .
Simulated call by reference This is the main way that a called function can modifiy more than one variable in the calling function. We have already seen the application of this technique via arrays and scanf, but we will delay a comprehensive discussion until you get to pointers in ENEE 150.
Variable argument lists An ellipsis (...) can be used to indicate that a function should expect to get a variable number of arguments. printf is an excellent example, because the number of arguments depends on the formatting in the character array : printf(const char[],...); We will not require you to write any functions with variable argument lists this semester.
Function prototypes Prototypes required if function defined after invocation. prototypes placed at beginning of calling function. prototypes can be generated by making a copy of the function header and placing a semicolon at end, though argument names are not required. scope of prototype: same rules as variables. prototypes can occur more than once, as long as they are consistent.
Default prototypes NOTE: C will try to make a prototype of the function at the first invocation if it is not already defined. This often causes an error. The return value is assumed to be int. The arguments may be promoted: shorts and chars are promoted to ints floats are promoted to doubles
math.h prototypes double acos (double __x); double asin (double __x); double atan (double __x); double atan2 (double __y, double __x); double ceil (double __x); double cos (double __x); double fmod (double __x, double __y); double frexp (double __x, int *__exponent); double ldexp (double __x, int __exponent); double modf (double __x, double *__ipart); double pow (double __x, double __y); double log (double __x); double log10 (double __x); double sin (double __x); double sinh (double __x); double sqrt (double __x); double tan (double __x); double tanh (double __x); double exp (double __x); double fabs (double __x); double floor (double __x); double cosh (double __x);
Program 6.1 A projectile is fired from earth with an initial velocity v (m/s) and at an initial angle Q from the surface. Calculate how high it reaches, h, how far it goes, d, and how long it remains in flight, t. This problem requires knowledge from PHYS 161, or equivalent. z v h y Q d
Solution to Program 6.1 in the z (vertical) direction: in the y (horizontal) direction: az= g = 9.81 m/s2 vz= v sin(Q) - g t z = v t sin(Q) - g t2/2 maximum time: when z=0 (again): tmax= 2 v sin(Q) / g maximum distance (y at tmax): d = 2sin(Q)cos(Q) v2/ g maximum height (z at vz= 0): h = v2sin2(Q)/(2g) because vz= 0 when t = tmax/ 2 = v sin(Q) / g ay= vy= v cos(Q) y = v t cos(Q)
Program 6.1 - specification start declare variables prompt for initial velocity and angle convert angle from degrees to radians calculate d, h, and t via functions. print d, h, and t. stop
Program 6.1 - part 1 /* trajectory program */ #include <stdio.h> #include <math.h> #define GRAVITY 9.81 #define PI 3.141592654 int main (void) { double dist(double,double),time(double,double),height(double,double); double theta, velocity; const double thcnv=PI/180.; printf("Enter initial velocity (m/s): "), scanf("%lf",&velocity); printf("Enter initial angle (degrees): "), scanf("%lf",&theta); theta*=thcnv; printf("Maximum distance = %f m\n",dist(velocity,theta)); printf("Maximum time = %f s\n",time(velocity,theta)); printf("Maximum height = %f m\n",height(velocity,theta)); return 0; }
Program 6.1 - part 2: functions double dist (double velocity, double theta) { return (velocity*velocity*sin(2*theta)/GRAVITY); } double time (double velocity, double theta) { return (2.0*velocity*sin(theta)/GRAVITY); } double height (double velocity, double theta) { return (velocity*velocity*sin(theta)*sin(theta)/2.0/GRAVITY); }
Recursion In C, a function can call itself! This often leads to elegant, compact codes It may not be the most efficient way in terms of execution time and/or space. A new, distinct copy of the function is made for each call. It can also get rather confusing... you may use recursion in your programs if you wish, but you will not be responsible for it on any exam.
Program 6.2 - factorials via recursion use a recursive function, based on the formula: n! = n*(n-1)! to compute the factorial.
Program 6.2 - specification start declare variables prompt for integer and read calculate factorial print stop
Recursive function - coding double factorial (double n) { if (n>1) return (n*factorial(n-1)); else return 1.; }
Main coding /* factorial program */ #include <stdio.h> #include <stdlib.h> int main (void) { double factorial (double); int n; char buff[BUFSIZ]; while(1) { printf("Enter Value for n: "); gets(buff); n=atoi(buff);
Main coding part 2 if (n<1) break; printf("%d ! = %14.6g\n",n,factorial(n)); } return 0; }