Understanding fork() System Call in C Programming
Demonstrates the concept of fork() system call in C programming, which creates a new process called child process that runs concurrently with the parent process. The child process inherits the program counter, CPU registers, and open files from the parent process. The return values of fork() indicate the success of creating the child process. The number of times 'Hello' is printed is determined by the number of processes created through fork(). Includes examples and explanations.
Download Presentation
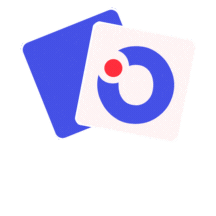
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
fork() in C Practical No 1
Fork System Call creates a new process called child process runs concurrently with process called parent process. both processes will execute the next instruction following the fork() system call. A child process uses the same pc(program counter), same CPU registers, same open files used by the parent process. It takes no parameters and returns an integer value. Negative Value creation of a child process was unsuccessful. Zero Returned to the newly created child process. Positive value Returned to parent. The value contains process ID of child process.
#include <stdio.h> #include <sys/types.h> #include <unistd.h> int main() { // make two process which run same // program after this instruction fork(); printf("Hello world!\n"); return 0; }
Calculate number of times hello is printed #include <stdio.h> #include <sys/types.h> int main() { fork(); fork(); fork(); printf("hello\n"); return 0; } Number of times hello printed is equal to number of process created. Total Number of Processes = 2n where n is number of fork system calls. So here n = 3, 23 = 8
#include <stdio.h> #include <sys/types.h> #include <unistd.h> int main() { forkexample(); return 0; } void forkexample() { // child process because return value zero if (fork() == 0) printf("Hello from Child!\n"); // parent process because return value non- zero. else printf("Hello from Parent!\n"); }