Introduction to Coding Ethics and Control Structures
Explore the fundamental concepts of coding ethics, including respect for privacy and confidentiality. Dive into control structures in programming, such as conditionals, logical operators, and relational operators. Gain insights into different types of control structures like sequential, selection/conditional, and iteration, along with examples and illustrations. Learn how to apply logical and relational operators effectively in coding practices.
Download Presentation
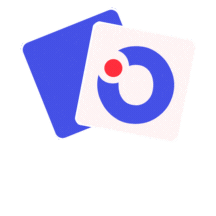
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Grade VIII Introduction to Coding Version 1.0
Ethics of coding Respect others privacy Honor confidentiality
Chapter 1: Conditionals in detail At the end of this this chapter you will get to understand conditionals in detail. You will know: IF ELSE and ELSE IF statement Using logical operators with IF ELSE statement Nested Condition
Types of control structures In programming, control structure is a block of program that accepts and analyses different variables and chooses the direction in which to go based on the given parameters. There are three basic types of control structures in programming: Sequential In a sequential control structure, the statements in a program are executed sequentially, i.e., step-by-step in an order that they are written. Selection / Conditional A selection (conditional) control structure is used to test a condition in a program. This control structure takes a decision to execute one statement/operation over another statement/operation depending on the condition. Iteration This control structure executes a set of statements for a certain number of times till the mentioned condition is true. Loops are examples of iterative statements.
IF ELSE and ELSE IF Simple illustration of conditionals using flowchart, to determine if a number is odd or even.
Logical Operators The three most important logical operators are AND, OR and NOT. The AND operator is used to see if two or more conditions are true. If all the conditions are true, the AND operator returns TRUE. If any one of the conditions fail, the AND operator returns FALSE. AND The OR operator is used to see if either one of two or more conditions is TRUE. If any of the condition is true, the OR operator returns TRUE. If all the conditions fail, the OR operator simply returns FALSE. OR We use the NOT operator to reverse or negate a condition. If the condition is true, NOT will return false and vice-versa. NOT
Relational Operators Operator Greater than Equal to Less than Greater than or equal to Symbol > == < >= Example x > y x == y x < y x >= y Meaning x greater than y x is equal to y x is less than y x is greater than or equal to y Less than or equal to <= x <= y x is less than or equal to y Not equal to != x! = y x not equal to y
Combined use of logical & relational operators Can you create a Triangle?
Precedence of Logical Operators Just like arithmetic operators, logical operators also have precedence that determines how the operations are grouped in the absence of parentheses. In an expression, the operator with highest precedence is grouped with its operands first, then the next highest operator will be grouped with its operands, and so on. In a situation where there are many logical operators of the same precedence, they will always be examined from left to the right. The figure of the left demonstrates the precedence of logical operators. Operator Precedence NOT (!) High AND (&&) Medium OR (||) Low
Chapter 2: Get creative with loops At the end of this this chapter you will understand Loops in programming. You will know: What are the different types of Loops? What is the definition of different types of Loops? What are Break and Continue Loops?
What are Loops? Everyday there are some tasks which need to be done repeatedly. Loops are programming elements which repeat a portion of the code for a set number of times till the desired process is complete. Repetitive tasks are common in programming and loops are important to save time and minimize errors.
Types of loops Loops make our code more manageable and organized. Let us now see what the different types of loops are: While Loop For Loop Nested Loop
For example - Print from 1 to 10 Here, if we want to derive the loop from this scenario, we have the following conditions: Condition: Write from 1 to 10 And the decision we are deriving is: Decision: Have we reached 10 The While loop The While loop can execute a set of commands till the condition is true While Loops are also called conditional loops. Once the condition is met then the loop is finished X=0 While X is not 10 X=X+1 Print (X)
For example We need to calculate the square of numbers present in a list Numbers = [1,3,5,7,13] The For loop #List of Integer Numbers A for loop is used for iterating over a sequence. The loop continues to execute until it reaches the end of the sequence. Numbers= [1,3,5,7,13] #Variable to store square of a number sq=0 for x in numbers: sq=x*x Print(sq)
Statements break and continue may be used within the loops to alter the control flow. Break & continue in loops Break statement terminates the loop and causes the execution to resume after the loop. Continue leaves the current iteration and executes with the next value in the loop. Continue skips the statements after the continue statement and keeps looping.
Nested loop The program shown below demonstrates the use of nested loops. Nested loop is a loop that occurs in another loop. When we nest one loop within another, the outer loop takes control of the total number of complete repetition of the inner loop.
Exit Criteria Exit criteria is defined as a condition that must be met before completing a specific task. It is a set of conditions that must exist before you can declare a program to be complete. Exit criteria is one of the most important components while defining a loop. As without an exit criterion, the program tends to enter in an infinite loop. These criteria differ from program to program as per the requirement.
Chapter 3: Functions in depth At the end of this chapter, you will understand functions in programming. You will have a better understanding of functions. You will know: How using functions helps us? Create and use functions in block coding
What are functions? If there is a block of code that you want your computer to repeat lots of times, then you might want to use a function A function is a block of code made up of a set of steps that results in a single specific action
Advantages of using functions Increases readability Code reusability increases By making code organized and easy to understand Redundant code is removed and replaced by functions
Parameters and return types Function to calculate area of a circle Variables accepted by a function to perform a set of actions defined in its body are called function parameters Return type Parameter Type of value returned by a function are called return types
Function to calculate sum of area & perimeter of a square by calling other functions Functions called within another function One or more functions can be defined within a program. These functions can then be called from other functions in the same program.
Chapter 4: Programming with arrays In this chapter we will see how we can work with large set of data in programming. What are arrays and its practical implementation in computers? Overview of collections
What are arrays: Arrays in programming are a collection of similar data type variables. Arrays do not support different data types in same collection. Arrays improve readability of code by using a single variable for a large set of data. Arrays in programming Limitations of using arrays are: You can only store variables with homogenous data types in an array. Arrays are always static and fixed in length and size. The variables in an array are always ordered sequentially with index starting with 0.
Arrays in programming Shown above is a character array of length 7
Suppose we have three variables to store three different colors: Color1 = red Color2 = green Color3 = blue Arrays in programming We can use an array to store three different colors in a single variable. Shown below is how we can store three different colors using a single variable using an array: Color = [ red , green , blue ]
Suppose we have an array named Color. Color = [ red , green , blue ] We can access the elements of the Color array the following way: Arrays in programming Color[0] = red Color[1] = green Color[2] = blue We can alter the value at an index of an array by assigning it a new value as shown below: Color[1] = yellow
Sorting an array can be defined as ordering the elements in the array either in ascending or descending order. 1 5 4 3 2 (SORT) Sorting an array 1 2 3 4 5 In the above scenario, we are sorting the elements of an integer array in ascending order. Some of the algorithms used to sort an array are: Bubble sort Quick sort
Bubble sort is a method of sorting that works by repeatedly swapping adjacent elements if they are in incorrect order. Let us consider a set of numbers 1, 5, 4, 3, 2; that needs to be sorted in ascending order. Sorting an array using bubble sort We use an integer array of length 5 to store these numbers. 1 5 4 3 2
To start with, we compare the first two numbers and find out which one is greater. We start with 1 and 5. Since 5 is already greater than 1, no change is made Sorting an array using bubble sort 1 5 4 3 2 Then we compare the numbers 5 and 4 Since 5 is greater than 4, we will swap these two numbers. 1 5 4 3 2
Next, we compare 5 and 3 Since 5 is greater than 3, we will swap the two numbers. 1 4 5 3 2 Sorting an array using bubble sort Lastly, we compare 5 and 2 Since 5 is greater than 2, we will swap these two numbers 1 4 3 5 2
The list of the numbers is rearranged as follows: Notice 5 is the largest number in this list and arranged at the last position. 1 4 3 2 5 Sorting an array using bubble sort We again start from the beginning and compare 1 with 4. Since 4 is greater than 1, no change is made. 1 4 3 2 5
Then we compare the numbers 4 and 3 Since 4 is greater than 3, we will swap these two numbers. 1 4 3 2 5 Sorting an array using bubble sort Next, we compare 4 and 2 Since 4 is greater than 2, we will swap the two numbers. 1 3 2 5 4
Lastly, we compare 4 and 5. Since, 5 is greater than 4, no change is made. 1 3 2 4 5 Sorting an array using bubble sort We again start from the beginning and compare 1 with 3. Since 3 is greater than 1, no change is made. 1 3 4 5 2
Then we compare the numbers 3 and 2 Since 3 is greater than 2, we will swap these two numbers. 1 3 2 4 5 Sorting an array using bubble sort Next, we compare the numbers 3 and 4. Since 4 is greater than 3, no change is made. 2 1 4 5 3
We again start from the beginning and compare 1 with 2. Since 2 is greater than 1, no change is made. 1 2 3 4 5 Sorting an array using bubble sort The numbers are now all sorted in ascending order. 1 2 3 4 5 The exercise of sorting is done until no more numbers need to be swapped.
Chapter 5: Advanced Sequencing In this chapter we will learn what is advanced sequencing in programming. What is a sequence? Why is sequencing important in programming? Sequencing with Loops and Conditions
What is a Sequence? A series of actions performed in a specific order is called sequence. We follow sequence in many things that we do on daily basis. Our daily routine is also a series of actions that we perform like: Wake up Have breakfast Take a shower Go to School Attend classes Come back home Do homework Have dinner Go to bed However, this routine might vary from person to person but for a specific person this might be the routine.
What is a Sequence? (Contd.) An algorithm is a set of steps to solve a problem. A program is written based on an algorithm to get the required result. Sequencing in algorithms is arranging the steps of an algorithm in a correct sequence so that we get the correct outcome. Sequencing is important in algorithms. If the steps of an algorithm are not in correct sequence, we will not get the required result.
Algorithm to calculate sum of two numbers Consider a simple algorithm to calculate the sum of two numbers. The steps of this algorithm would look like as shown in the flowchart on the left. However, we need to note that if we swap 2 steps and add Num1 and Num2 before taking Num1 as the input, the program will not give the required result or give an error. This is called as a bug.
Sequencing with Loops and Conditions Most programs consist of 3 main components Sequence, Selections (Conditions) and Loops. If we write a program to get all the numbers divisible by 3 between 0 and 100. The flowchart for the algorithm would be as shown in the image on the left. In this example, we see that this program has a sequence of conditions and loops.