Overview of 8086 Assembly Language Arithmetic Operations
The 8086 assembly language provides instructions for arithmetic operations such as addition, subtraction, and comparison. These operations are essential for manipulating data in memory and registers. The instructions support various operand types, including registers, memory locations, and immediate values. After performing arithmetic operations, the result is stored in the first operand. Flags such as Carry Flag (CF), Zero Flag (ZF), Sign Flag (SF), Overflow Flag (OF), and Parity Flag (PF) are affected by most arithmetic and logic instructions. Understanding these concepts is crucial for programming in 8086 assembly language.
Download Presentation
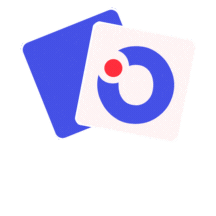
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Arithmetic Operations Addition Subtraction comparison System Programming Lab Computer Engineering Department College of Engineering
Objectives Write programs in 8086 assembly language for adding, subtraction, comparison by using different size of data as bytes or words. Use arithmetic instructions to accomplish simple binary, BCD, andASCII arithmetic. and
Theory The arithmetic instructions found in any microprocessor include addition, subtraction, and comparison. Also shown are their uses in manipulating register and memory data. These operations used any addressing mode with 8-bit or 16 bit, most arithmetic instructions can be divided into two groups of instructions: First Group : ADD, ADC, SUB, SBB, CMP Second group: INC, DEC
Theory These types of operands are supported: REG, memory memory, REG REG, REG memory, immediate REG, immediate REG:AX, BX, CX, DX, DI, SI, BP, SP. Memory: [BX], [BX+SI+7], variable, etc... Immediate: 5, -24, 3Fh, 10001101b, etc...
Theory After operation between operands, result is always stored in first operand. CMP instructions effect flags only and do not store a result. These instructions affect these flags only: CF, ZF, SF, OF, PF, AF
First Group ADD - add second operand to first. ADC - add carry flag (C) to the operand data. SUB - Subtract second operand to first. SBB - subtract carry flag (C) from the deference. CMP - Subtract second operand from first for flags only.
Second Group INC, DEC instructions affect these flags only ZF, SF, OF, PF, AF INC - add 1 to a register or the content of a memory location. DCR- subtract 1 from a register or the content of a memory location.
Flags Most Arithmetic and Logic Instructions affect the processor status register (Flags)
Flags Carry Flag (CF) - set to 1 when there is an unsigned overflow. Zero Flag (ZF) - set to 1 when result is zero. Sign Flag (SF) - set to 1 when result is negative. Overflow Flag (OF) - set to 1 when there is a signed overflow. Parity Flag (PF) - set to 1 when there is even number of one bits in result. Even if result is a word only 8 low bits are analyzed!
Flags Auxiliary Flag (AF) - set to 1 when there is an unsigned overflow for low nibble (4 bits). Interrupt enable Flag (IF) - when this flag is set to 1 CPU reacts to interrupts from external devices. Direction Flag (DF) instructions to process data chains, when this flag is set to 0 - the processing is done forward, when this flag is set to 1 the processing is done backward. - used by some
Add Instr. Operands Description Algorithm: REG , Memory Memory , REG REG , REG Memory , Immediate REG , Immediate operand1 = operand1 + operand2 ADD Example: MOV AL, 5 ; AL = 5 ADD AL, -3 ; AL = 2 RET Flags: C, Z, S, O, P, A
Add with Carry Instr. Operands Description Algorithm: operand1 = operand1 + operand2 + CF Example: STC ; set CF = 1 MOV AL, 5 ; AL = 5 ADC AL, 1 ; AL = 7 RET Flags: C, Z, S, O, P, A REG , Memory Memory , REG REG , REG Memory , Immediate REG , Immediate ADC
Subtract Instr. Operands Description Algorithm: operand1 = operand1 - operand2 REG , Memory Memory , REG REG , REG Memory , Immediate REG , Immediate Example: MOV AL, 5 SUB AL, 1 ; AL = 4 RET SUB Flags: C, Z, S, O, P, A
Subtract with Borrow Instr. Operands Description Algorithm: operand1 = operand1 - operand2 - CF Example: STC MOV AL, 5 SBB AL, 3 ; AL = 5 - 3 - 1 = 1 RET REG , Memory Memory , REG REG , REG Memory , Immediate REG , Immediate SBB Flags: C, Z, S, O, P, A
Compare Instr. Operands Description Algorithm: operand1 - operand2 result is not stored anywhere, flags are set (OF, SF, ZF, AF, PF, CF) according to result. REG , Memory Memory , REG REG , REG Memory , Immediate REG , Immediate CMP Example: MOV AL, 5 MOV BL, 5 CMP AL, BL ; AL = 5, ZF = 1
Increment Instr. Operands Description Algorithm: operand = operand + 1 REG Memory INC Example: MOV AL, 4 INC AL ; AL = 5 RET Flags: Z, S, O, P, A
Decrement Instr. Operands Description Algorithm: operand = operand 1 REG Memory DEC Example: MOV AL, 255 ; AL = 0FFh DEC AL ; AL = 0FEh RET Flags: Z, S, O, P, A
Examples Add two consecutive bytes of data stored at the data segment offset location NUMB & NUMB+1, the result is stored in memory location NUMB+2. ORG 100 MOV DI, OFFSET NUMB ;address NUMB. MOV AL, 00H ;clear sum. ADD AL, [DI] ;add NUMB ADD AL, [DI+1] ;add NUMB+1 MOV [DI+2], AL ;result in NUMB+2 HLT NUMB DB 4AH, 2FH, 00H
Array Addition Memory array are sequential list of data. Array is a variable has block of data from element 0 through n. An array of data contains 10 bytes numbers from element 0 through 9, write program to add the content of array elements 3, 5 & 7. ORG 100 MOV AL, 00H MOV SI, 3 ADD AL, ARRAY[SI] ;address NUMB. ADD AL, ARRAY[SI+2] ;address NUMB. ADD AL, ARRAY[SI+4] ;address NUMB. HLT ARRAY DB 00H, 10H, 20H, 30H, 40H, 50H, 60H, 70H, 80H, 90H
Examples Write a complete program to find F where: F=112345 + 4466h 31122, Store the result at memory location offset 300h. ORG 100 SBB BX, 00H MOV AX, 0B6D9H MOV SI, OFFSET F ; 112345 = 1B6D9h MOV BX, 0001H MOV DI, 0300H MOV CX, 4466h MOV [SI], AX MOV DX, 7992h MOV [DI], AX ; 31122 = 7992h ADD AX, CX MOV [SI+2], BX ADC BX, 00 MOV [DI+2], BX SUB AX, DX HLT F DW 0000h, 0000h
Examples Repeat the program by using 2 s complement for the subtraction: F=112345 + 4466h 31122 ORG 100 ADC BX, 00H MOV AX, 0B6D9H MOV SI, OFFSET F ; 112345 = 1B6D9h MOV BX, 0001H MOV DI, 0300H MOV CX, 4466h MOV [SI], AX MOV DX, 7992h MOV [DI], AX ; 31122 = 7992h ADD AX, CX MOV [SI+2], DX ADC BX, 00 MOV [DI+2], DX NEG DX HLT ADD AX, DX F DW 0000h, 0000h
Loop Instruction Instr. Operands Description Decrease CX, jump to label if CX not zero Algorithm: CX = CX - 1 if CX <> 0 then jump else no jump, continue ORG 100h MOV CX, 5 label1: PRINTN 'loop!' LOOP label1 RET Flags: NON LOOP label
Examples Write 8086 program that sum the contents of BLOCK1 and BLOCK2 and store the results overtop of date in BLOCK2. Assume each block contains 50 elements. MOV SI, OFFSET BLOCK1 MOV [DI+BX], AL MOV DI, OFFSET BLOCK2 INC BX MOV BX, 00H LOOP L1 MOV CX, 50 HLT L1: BLOCK1 DB 50 DUP (01H) MOV AL, [SI+BX] BLOCK2 DB 50 DUP (02H) ADC AL, [DI+BX]
Examples Write an 8086 assembly program to implement the Pascal segment. SUM:=0 FOR I=0 TO 15 DO SUM:= SUM + A(I) MOV SI, OFFSET A MOV SUM, AL MOV CX, 0FH HLT MOV AL, 00H A DB 15DUP(02H) L1: SUM DB 00H ADC AL, [SI] INC SI LOOP L1