Android Background Tasks: Notifications, Timers, Alarms, and Location Tracking
Learn how to create notifications, schedule tasks, set timers, use alarms, and track location in the background on Android. This comprehensive guide covers NotificationManager, Handlers, AlarmReceiver, and IntentServices for performing tasks efficiently without constant user interaction.
Download Presentation
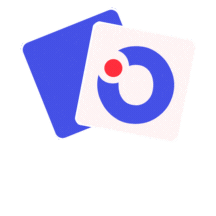
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
NotificationManager manager = (NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE); Notifying from the Background Intent launchIntent = new Intent(getApplicationContext(), NotificationActivity.class); PendingIntent contentIntent = PendingIntent.getActivity(getApplicationContext(), 0, launchIntent, 0); //Create notification with the time it was fired Notification note = new Notification(R.drawable.icon, "Something Happened", System.currentTimeMillis()); //Set notification information note.setLatestEventInfo(getApplicationContext(), "We're Finished!", "Click Here!", contentIntent); note.defaults |= Notification.DEFAULT_SOUND; note.flags |= Notification.FLAG_AUTO_CANCEL; manager.notify(NOTE_ID, note); FLAG_INSISTENT - Repeats the Notification sounds until the user responds. FLAG_NO_CLEAR - Does not allow the Notification to be cleared with the user s Clear Notifications button; only through a call to cancel().
private Handler mHandler = new Handler(); private Runnable timerTask = new Runnable() { @Override public void run() { Calendar now = Calendar.getInstance(); mClock.setText(String.format("%02d:%02d:%02d", now.get(Calendar.HOUR), now.get(Calendar.MINUTE), now.get(Calendar.SECOND)) ); Creating Timed and Periodic Tasks //Schedule the next update in one second mHandler.postDelayed(timerTask,1000); } }; @Override public void onResume() { super.onResume(); mHandler.post(timerTask); } @Override public void onPause() { super.onPause(); mHandler.removeCallbacks(timerTask); } }
Scheduling a Periodic Task public class AlarmReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { //display the current time Calendar now = Calendar.getInstance(); DateFormat formatter = SimpleDateFormat.getTimeInstance(); Toast.makeText(context, formatter.format(now.getTime()), Toast.LENGTH_SHORT).show(); } }
Scheduling a Periodic Task Intent launchIntent = new Intent(this, AlarmReceiver.class); mAlarmIntent = PendingIntent.getBroadcast(this, 0, launchIntent, 0); public void onClick(View v) { AlarmManager manager = (AlarmManager)getSystemService(Context.ALARM_SERVICE); long interval = 5*1000; //5 seconds switch(v.getId()) { case R.id.start: Toast.makeText(this, "Scheduled", Toast.LENGTH_SHORT).show(); manager.setRepeating(AlarmManager.ELAPSED_REALTIME, SystemClock.elapsedRealtime()+interval, interval, mAlarmIntent); break; case R.id.stop: Toast.makeText(this, "Canceled", Toast.LENGTH_SHORT).show(); manager.cancel(mAlarmIntent); break; default: break; }
Creating Sticky Operations IntentService is a wrapper around Android s base Service implementation, the key component to doing work in the background without interaction from the user. Running Persistent Background Operations running in the background indefinitely, performing some operation or monitoring certain events to occur. public void startTracking() { if(!manager.isProviderEnabled(LocationManager.GPS_PROVIDE R)) { return; } Toast.makeText(this, "Starting Tracker", Toast.LENGTH_SHORT).show(); manager.requestLocationUpdates(LocationManager.GPS_PROVI DER, 30000, 0, this); isTracking = true; } public void stopTracking() { Toast.makeText(this, "Stopping Tracker", Toast.LENGTH_SHORT).show(); manager.removeUpdates(this); isTracking = false; } public void onCreate() { manager = (LocationManager)getSystemService( LOCATION_SERVICE); storedLocations = new ArrayList<Location>(); Log.i(LOGTAG, "Tracking Service Running..."); }
Launching Other Applications private void viewPdf(Uri file) { Intent intent; intent = new Intent(Intent.ACTION_VIEW); intent.setDataAndType(file, "application/pdf"); try { startActivity(intent); } catch (ActivityNotFoundException e) { //No application to view, ask to download one AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("No Application Found"); builder.setMessage("We could not find an application to view PDFs." +" Would you like to download one from Android Market?"); builder.setPositiveButton("Yes, Please", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Intent marketIntent = new Intent(Intent.ACTION_VIEW); marketIntent.setData(Uri.parse("market://details?id=com.adobe.reader")); startActivity(marketIntent); } }); builder.setNegativeButton("No, Thanks", null); builder.create().show(); } }
Launching System Applications Contact Picker SMS Intent pickIntent = new Intent(); pickIntent.setAction(Intent.ACTION_PICK); pickIntent.setData(URI_TO_CONTACT_TABLE); Intent smsIntent = new Intent(); smsIntent.setAction(Intent.ACTION_VIEW); smsIntent.setType( vnd.android-dir/mms-sms ); smsIntent.putExtra( address , 8885551234 ); smsIntent.putExtra( sms_body , Body Text ); To display a web page Intent pageIntent = new Intent(); pageIntent.setAction(Intent.ACTION_VIEW); pageIntent.setData(Uri.parse( http://WEB_ADDRESS_TO_VIEW ));