Week 5 -Monday
Scala is a versatile programming language that emphasizes reducing coupling within code and writing in a modular style. The importance of placing code into packages and managing dependencies is highlighted, showcasing how Scala handles structures like unnamed packages and multiple packages. The discussion delves into aspects such as traits, package organization, and code modularity, emphasizing the need for reducing coupling to enhance program flexibility and maintainability. Scala's similarities with Java and its consistent differences come to the forefront, showcasing its robust capabilities for developing scalable and efficient applications.
Download Presentation
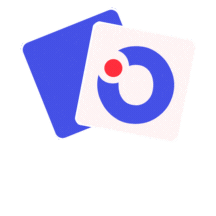
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
The extent to which various parts of a program rely on each other is known as coupling When working on larger programs the tendency for coupling to occur when changing small portions of code within them grows Programmers should work to reduce coupling within the code they work on Writing in a modular style
Although most of what the chapter covers is very similar to Java, the differences are consistent compared to the previous versions
Most 0f the code we have written in Scala so far has been in the unnamedpackage There are two ways to place code into packages package rocketsthatgobrrrr.nav class Navigator package notbrrrrrrockets.nav{ class Navigator }
Fitting multiple packages is also still a thing! package rocketsthatgobrrrr { package nav { class Navigator package tests { class SuiteLifeofNavigatorTesting } } }
Fitting multiple packages is also still a thing! package rocketsthatgobrrrr package nav
Concise access to related packages package rocketsthatgobrrrr { package nav { class Navigator{ val map = new SuperMarioGalaxy3DMap } class SuperMarioGalaxy3DMap{ } class Ship{ val nav = new nav.Navigator } package fleets { class Fleet{ def addShip() = {new Ship} } } } }
package rocketsthatgobrrrr { class Ship } package rocketsthatgobrrrr.fleets{ class Fleet{ def addShip() = {new Ship} } } This doesn t compile!
package rocketsthatgobrrrr package fleets class Fleet{ def addShip() = {new Ship} } This does!
import supermeatboy.Meat import supermeatboy.Meat._ import supermeatboy._ Wildcards are great!
package supermeatboy abstract class Meat( val name: String, val color: String ) object Meats{ object BadMeat extends Meat ( That was some bad meat , pretty bad ) object DecentMeat extends Meat ( That meat was decent , decent ) object Brisket extends Meat ( I need more brisket , Yes ) }
The ways that Scala does import clauses differ in that they can be more flexible than in Java They can appear anywhere! Can refer t0 objects alongside packages Let you rename and hide members
In Scala you can hide or rename members via the import selector clause which are done with curly braces {} import Fruits.{Apple, Orange}//grabbing only what is inside braces import Fruits.{Apple=>iMissYouSteve, Orange=>aBetterAnalogyThanTheLastOne}//renames Apple to iMissYouSteve etc import Fruits.{_} //same as import Fruits._ import Fruits.{Apple=>iMissYouSteve,_} //imports all from Fruits and import Fruits.{Pear=>_,_}//imports everything except Pear
Clauses Renaming: x => y (x member will be visible under the name y) Hiding: x => _ (this excludes x from the set of imported names) A catch-all: _ (imports all members except those mentioned in the previous clause, always comes last in import selectors)
Scala has these for you automatically import java.lang._ import scala._ Import Predef._
Labeling a member private makes it visible only inside the class/object with the members definition class WallMaria{ class WallRosa{ private def f() = { println( f ) } class InnerMost { f() //this is fine } } (new WallRosa).f() //this is not so fine }
A bit more restricted than in Java Only accessible from subclasses of the class where the member is defined package notReal { class Super { protected def f() = { println( f ) } } class Sub extends Super { f() } class Other { (new Super).f() //this doesn t work! F is unaccessible } }
There is no explicit modifier for public, any member that is not labeled is public Public members can be accessed from anywhere
Modifiers can be augmented with qualifiers Ex. private[x] or protected[x] means the access to those members is private/protected up to X, X being the enclosing package or class package rocketsthatgobrrrr { package navigation { private[rocketsthatgobrrrr] class Navigator { protected[navigation] def useStarChart() = {} class LegOfJourney { private[Navigator] val distance = 100 } private [this] var speed = 200 } } package launch { import navigation._ object Vehicle { private[launch] val guide = new Navigator } }
There are no static members in Scala, in Java static and instance members belong to the same class Instead, there is a companion object that contains members that exist only once class Rocket { import Rocket.fuel private def canGoHomeAgain = fuel > 20 } object Rocket { private def fuel = 10 def chooseStrategy(rocket: Rocket) = { if(rocket.canGoHomeAgain) goHome() else goBrrr() } def goHome() = {} def goBrrr() = {} }
Any kind of definition that can be put into a class can also be at the top of the package To do so we need to put said definitions into a package object Each package is allowed one package object Any definitions inside the package are considered members of the package itself
package object abesdelight { def showFruit (fruit: Fruit) = { import fruit._ println(name + s are + color) } } package printmenu import abesdelight.Fruits import abesdelight.showFruit object PrintMenu { def main(args: Array[String]) = { for (fruit <- Fruits.menu) { showFruit(fruit) } } }
Assertions and Tests Actually, I ve been Fired!
First Energy Career Day Virtual event on February 18, 2021, 9:30 a.m. to noon Registration deadline of February 16, 2021 Register here: https://www.cvent.com/d/7jqkwh