Understanding Spring IoC Container and Bean Scopes
Learn about Spring IoC container, beans, scopes, and annotations in the context of Spring MVC. Explore the concept of beans, their creation, assembly, and management within Spring. Understand the various scopes available for beans and their implications, such as Singleton and Prototype. Discover the importance of avoiding stateful services in web applications and how to leverage the power of Spring annotations for dependency injection and configuration.
Download Presentation
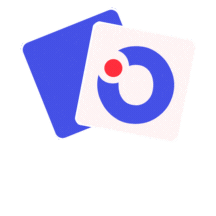
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Spring MVC Beans, Sessions, Listeners, Filters Dr Solange Karsenty Spring Java Course
More on annotations @Component Spring Component annotation is used to denote a class as Component. It means that it will autodetect these classes for dependency injection when annotation-based configuration and classpath scanning is used. A simple component is called a Bean (beans are registered in the application context). It has members and getters/setters. @Service and @Repository are special cases of @Component. @Service Beans that hold business logic @Repository Database operations
Stateful services We learned previously that we can store data (for example in Application context, sessions) Objects to store application data are called beans In spring you have 6 types of scopes for example to store in application context you use the @Bean annotation and AnnotationConfigWebApplicationContext.getBean( myvariable") However this may lead to stateful applications: States are stored on server Why are stateful services not recommended? What can we do to avoid stateful services? Web Browser (has state) <-> Web Server (stateless) <-> Database (has state)
What is a Bean? A bean is an object that is instantiated, assembled, and managed by a Spring IoC (inversion of control) container. This is also called wiring . beans are created with the configuration metadata that you supply to the container, using annotations. You can control not only the various dependencies and configuration values that are to be plugged into an object that is created from a particular bean definition, but also the scope of the objects created from a particular bean definition. Any class can serve for Beans creation, usually beans contain mostly getter/setters
Beans scopes / @Scope() Singleton: the container creates a single instance of that bean per ApplicationContext, and all requests for that bean name will return the same object. Not thread safe! Prototype: a different instance every time it is requested from the container. Request Session Application (known as ServletContext) What s the difference with Singleton? application scoped bean is singleton per ServletContext, whereas singleton scoped bean is singleton per ApplicationContext. There can be multiple application contexts for a single application. Websocket WebSockets is a bi-directional, full-duplex, persistent connection between a web browser and a server. Note that singleton and application scopes may require you to write thread safe code! (synchronized sections) Reference: https://howtodoinjava.com/spring5/core/spring-bean-scopes-tutorial/ @Bean @Scope("singleton") public Person personSingleton() { return new Person(); }
Example: session bean // create the bean @Bean @Scope(value = WebApplicationContext.SCOPE_SESSION, proxyMode = ScopedProxyMode.TARGET_CLASS) public TodoList todos() { return new TodoList(); } The bean TodoList is configured as a session-scoped bean Need to add a param because Bean is created by target class (session scope exists only in controller) @Controller @RequestMapping("/mytodos") public class TodoController { @Resource(name = "todos") private TodoList todos; // . . . } Use it in the controller: use the same name as in @Bean examples: https://www.journaldev.com/21039/spring-bean-scopes https://www.baeldung.com/spring-bean-scopes
Spring sessions By default Apache Tomcat stores HTTP session objects in memory. However you can choose among the following implementations: Spring Session Core - provides core Spring Session functionalities and APIs Spring Session Data Redis - provides SessionRepository and ReactiveSessionRepository implementation backed by Redis and configuration support (see the course example) Spring Session JDBC - provides SessionRepository implementation backed by a relational database and configuration support (example on moodle) Spring Session Hazelcast - provides SessionRepository implementation backed by Hazelcast and configuration support
Typical application (maven based) pom.xml contains package dependencies (Maven is a build and dependency management tool/ There are other tools available like Gradle) java is the source code We usually separate classes into packages: controllers, db stuff, utils etc.. resources contains html pages Templates: views of the template engine Application.properties is a config file You can put anything you want Spring use it for various packages, for example to define the jdbc url and credentials
Interceptors (previously filters) Interceptors handle requests before controllers interceptors handle responses before views Useful for logging, multilingual apps, etc Example available on course website Configuration annotation @Configuration public class MyConfig implements WebMvcConfigurer { @Override public void addInterceptors(InterceptorRegistry registry){ registry.addInterceptor(new LoggingInterceptor()).addPathPatterns("/*"); }
Spring Events (listeners) Events we already know: Session creation/destroy, Context creation/destroy Spring allows you to create custom events Rules to handles events in Spring the event should extend ApplicationEvent the publisher should inject an ApplicationEventPublisher object the listener should implement the ApplicationListener interface Example: https://www.baeldung.com/spring-events
Listeners Attach handlers to events you define classes that extend HttpSessionListener, ServletContextListener etc Then Register the listener classes in your application configuration class Recent version of Spring simplified with @EventListener Type of listener is defined by the type of param Listener annotation @EventListener public void myHandler(ContextStartedEvent ctxStartEvt) { System.out.println("Context Start Event received." + ctxStartEvt.toString()); } Can also specify the type as: @EventListener({ ContextStartedEvent.class, ApplicationReadyEvent.class})
Using Redis Session listeners do not work with Session based on JPA/mysql You must use Redis (another server) for sessions Short guide howto: in pom.xml: <dependency> <groupId>com.github.kstyrc</groupId> <artifactId>embedded-redis</artifactId> <version>0.6</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> <version>2.1.5.RELEASE</version> </dependency> and in your configuration class: @Configuration public class MyConfiguration {private final redis.embedded.RedisServer redisServer; public MyConfiguration(@Value("${spring.redis.port}") final int redisPort) throws IOException { this.redisServer = new redis.embedded.RedisServer(redisPort); } @PostConstruct public void startRedis() { this.redisServer.start(); } in the properties: spring.session.store-type=redis spring.session.redis.flush-mode=on_save spring.redis.host=localhost spring.redis.password= spring.redis.port=6379 @PreDestroy public void stopRedis() { this.redisServer.stop(); }
Other libraries There are many more libraries that extend Spring For example Lombok is a library to inject constructors and lots of boilerplate code See also logging with Log4j2
lombok A popular library to inject setter/getters Add to your pom.xml: <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> Allows you to write: @Getter @Setter private String name; Note for IntelliJ: you may need to install lombok in the plugins section Also tick the annotations checkbox at: 1.) Preferences -> Compiler -> Annotation Processors 2.) File -> Other Settings -> Preferences -> Compiler -> Annotation Processors
References https://spring.io/guides https://docs.spring.io/spring/docs/current/spring-framework- reference/index.html https://howtodoinjava.com/spring-5-tutorial/ REST: https://www.baeldung.com/rest-with-spring-series