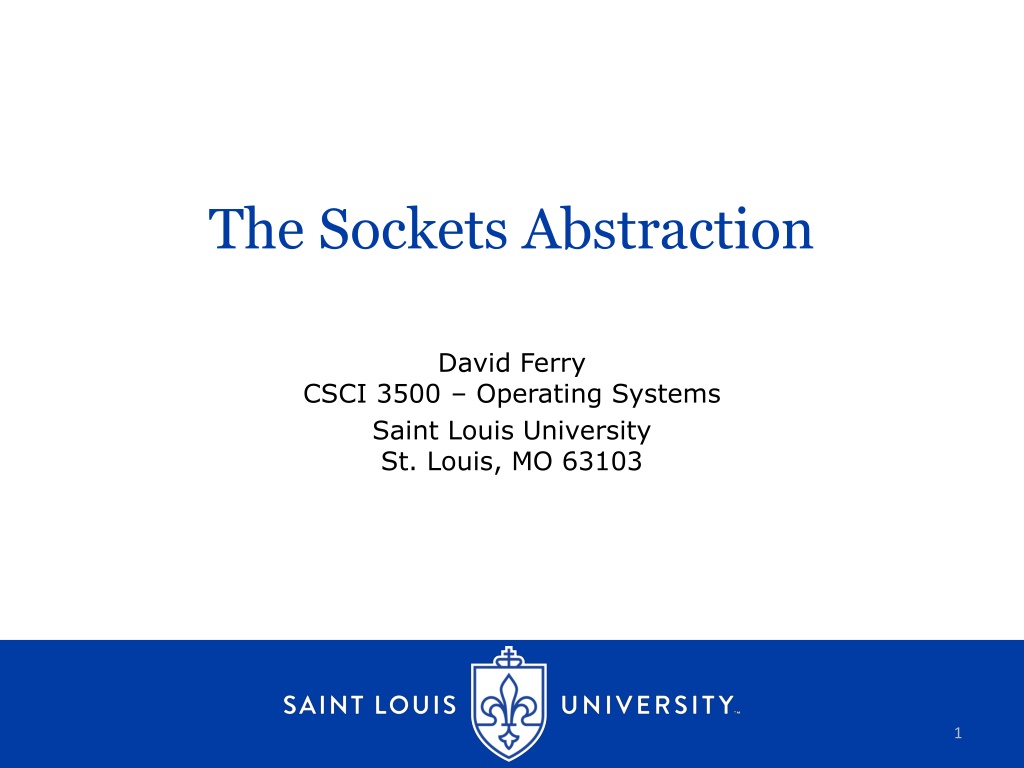
Understanding Sockets Abstraction in Operating Systems
Explore the sockets abstraction in operating systems, connecting processes independently of underlying technology. Learn about creating TCP/IP communication channels using sockets for server and client sides. Discover how processes interact with sockets and the role of the OS in managing communication endpoints.
Download Presentation
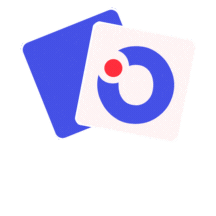
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
The Sockets Abstraction David Ferry CSCI 3500 Operating Systems Saint Louis University St. Louis, MO 63103 1
Recall: 7-Layer OSI Model Application to Application Application Application Protocol to Protocol Presentation Presentation Session Session Entity to Entity User Space Transport Transport Process to Process Kernel Space Network Network Interface to Interface Data-Link Data-Link Sockets Hardware to Hardware Physical Physical Physical Connection Last time: The transport layer converts the ability to send individual messages into a communication channel with semantics and guarantees Today: The sockets abstraction, which is the interface between the kernel space transport layer and the user space layers CSCI 3500 - Operating Systems 2
Sockets Sockets are communication endpoints that connect processes Independent of the underlying technology Follows the everything is a file philosophy Processes have a single read/write interface to sockets, and the OS is responsible for making it work Processes don t need to know how a socket is implemented, other than specifying what kinds of semantics are wanted TCP/IP UDP/IP Process B Process A Memory HAM Radio Processes can swap out underlying technology just by changing the socket configuration (good for testing, etc.)
Creating a TCP/IP Communication Channel with Sockets: Server Side Follows the client/server model, or could call it the connector/listener model: Server: 1. Create socket with socket() system call 2. bind() address to that socket For TCP/IP this means a port number Other methods have different address conventions- memory mapped uses a filesystem path 3. listen() on socket Configures incoming connections queue and tells OS to start taking connections 4. Wait for connection attempts with accept() Blocks by default CSCI 3500 - Operating Systems 4
Creating a TCP/IP Communication Channel with Sockets: Client Side Follows the client/server model, or could call it the connector/listener model: Client side: 1. Create socket with socket() 2. connect() to remote a remote socket Notes: Both sides need a port number for IP address, but only the client needs an IP address UDP server does not listen() or accept(), UDP client does not connect() Once configured, TCP/UDP is transparent, but it does effect how communications are handled! UDP can fail silently! CSCI 3500 - Operating Systems 5
Address Configuration with Sockets Address formats differ between technologies, but we only have one set of system calls: Recall that Pthreads had to use a lot of void* pointers Socket system calls use a generic struct sockaddr pointer You should specialize this to an appropriate type, e.g. TCP/IP uses struct sockaddr_in, while Unix domain sockets (delivered via memory) use struct sockaddr_un Documented at man 7 ip, man 7 unix, etc. Address formats must be in network-byte order: Use htons() to convert port number Use inet_aton() to convert IP address string a.b.c.d CSCI 3500 - Operating Systems 6
Address Configuration Example Suppose we want to connect to 1.2.3.4 on port 12345: char* ipAddr = 1.2.3.4 ; int port = 12345; struct sockaddr_in address; //Creates address struct //Initialize address struct to zeros memset( &address, 0, sizeof( struct sockaddr_in ) ); address.sin_family = AF_INET; //Set family address.sin_port = htons( port ); //Set port inet_aton( ipAddrString, &address.sin_addr ); //Set IP addr CSCI 3500 - Operating Systems 7