Priority Queues and Heap Data Structures
Priority queues play a key role in computer science algorithms by managing data based on priority levels. The use of heap data structures enhances the efficiency of priority queue operations. This tutorial covers the basics of priority queues, their applications, different implementations such as list-based and heap-based, and potential implementations for optimal performance. Additionally, it explores the concepts of balanced binary trees and their relevance in maintaining the structure of data in an organized manner.
Download Presentation
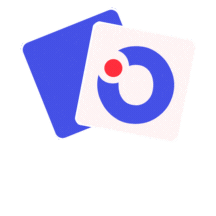
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Priority Queue with Heap Priority Queue with Heap CS 330 Intro to the Design and Analysis of Algorithms Lecture note Sajedul Talukder
Priority Queue ADT 1. PQueue data : collection of data with priority 2. PQueue operations insert deleteMin 3. PQueue property: for two elements in the queue, x and y, if x has a lower priority value than y, x will be deleted before y 1
Applications of the Priority Queue Select print jobs in order of decreasing length Forward packets on routers in order of urgency Select most frequent symbols for compression Sort numbers, picking minimum first Anythinggreedy 2
List-based Priority Queue Unsorted list implementation Store the items of the priority queue in a list-based sequence, in arbitrary order sorted list implementation Store the items of the priority queue in a sequence, sorted by key 4 5 2 3 1 1 2 3 4 5 Performance: insertItem takes O(n) time since we have to find the place where to insert the item removeMin, minKey and minElement take O(1) time since the smallest key is at the beginning of the sequence Performance: insertItem takes O(1) time since we can insert the item at the beginning or end of the sequence removeMin, minKey and minElement take O(n) time since we have to traverse the entire sequence to find the smallest key
Potential Implementations insert deleteMin Unsorted list (Array) O(1) O(n) Unsorted list (Linked-List) O(1) O(n) Sorted list (Array) O(n) O(1)* Sorted list (Linked-List) O(n) O(1) 4
Heaps and Priority Queues 2 5 6 9 7
Balanced binary trees Recall: The depth of a node is its distance from the root The depth of a tree is the depth of the deepest node A binary tree of depth n is balanced if all the nodes at depths 0 through n-1 have two children n-2 n-1 n Balanced Not balanced 6
Left-justified binary trees A balanced binary tree of depth n is left- justified if: it has 2nnodes at depth n(the tree is full ), or it has 2knodes at depth k, for all k < n, and all the leaves at depth n are as far left as possible Left-justified Not left-justified 7
What is a heap? A heap is a left-justified balanced binary tree storing keys at its internal nodes and satisfying the following properties: Heap-Order: for every internal node v other than the root, key(v) key(parent(v)) Complete Binary Tree: let h be the height of the heap for i = 0, , h 1, there are 2i nodes of depth i at depth h 1, the internal nodes are to the left of the leaf nodes The last node of a heap is the rightmost internal node of depth h 1 2 5 6 9 7 last node Max-heap vs Min-heap? We do Max-heap here
Height of a Heap Theorem: A heap storing nkeys has height O(log n) Proof: (we apply the complete binary tree property) Let h be the height of a heap storing n keys Since there are 2i keys at depth i=0, , h 2 and at least one key at depth h 1, we have n 1 + 2 + 4 + + 2h 2 + 1 Thus, n 2h 1 , i.e., h log n + 1 depth keys 0 1 1 2 h 2 2h 2 h 1 1
Building up to heap sort How to build a heap How to maintain a heap How to use a heap to sort data 10
The heap property A node has the heap property if the value in the node is as large as or larger than the values in its children 12 12 12 8 3 8 12 8 14 Orange node has heap property Orange node has heap property Orange node does not have heap property All leaf nodes automatically have the heap property A binary tree is a heap if all nodes in it have the heap property 11
Heapify Given a node that does not have the heap property, you can give it the heap property by exchanging its value with the value of the larger child 12 14 8 14 8 12 Orange node does not have heap property Orange node has heap property This is sometimes called Heapify (sifting down) 12
Heapify void heapify(int arr[], int n, int i) { int largest = i; // Initialize largest as root int l = 2*i ; // left = 2*i int r = 2*i + 1; // right = 2*i + 1 // If left child is larger than root if (l < n && arr[l] > arr[largest]) largest = l; // If right child is larger than largest so far if (r < n && arr[r] > arr[largest]) largest = r; // If largest is not root if (largest != i) { swap(arr[i], arr[largest]); // Recursively heapify the affected sub-tree heapify(arr, n, largest); } }
Constructing a heap I A tree consisting of a single node is automatically a heap We construct a heap by adding nodes one at a time: Add the node just to the right of the rightmost node in the deepest level If the deepest level is full, start a new level Examples: Add a new node here Add a new node here 14
Constructing a heap II Each time we add a node, we may destroy the heap property of its parent node To fix this, we sift up But each time we sift up, the value of the topmost node in the sift may increase, and this may destroy the heap property of its parent node We repeat the sifting up process, moving up in the tree, until either We reach nodes whose values don t need to be swapped (because the parent is still larger than both children), or We reach the root 15
Constructing a heap III 8 8 10 10 10 8 8 5 1 2 3 10 10 12 8 5 12 5 10 5 12 8 8 4 16
Other children are not affected 12 12 14 10 5 14 5 12 5 8 14 8 10 8 10 The node containing 8 is not affected because its parent gets larger, not smaller The node containing 5 is not affected because its parent gets larger, not smaller The node containing 8 is still not affected because, although its parent got smaller, its parent is still greater than it was originally 17
Exercise: Constructing Heap Build a heap from the following input sequence: (22, 15, 36, 44, 10, 3, 9, 13, 29, 25, 2, 11, 7, 1, 17)
A sample heap Here s a sample binary tree after it has been heapified 25 22 17 19 22 14 15 18 14 21 3 9 11 Notice that heapified does not mean sorted Heapifying does not change the shape of the binary tree; this binary tree is balanced and left-justified because it started out that way 19
Removing the root (animated) Notice that the largest number is now in the root Suppose we discard the root: 25 22 17 19 22 14 15 18 14 21 3 9 11 How can we fix the binary tree so it is once again balanced and left-justified? Solution: remove the rightmost leaf at the deepest level and use it for the new root 20
Removing the root (animated) Notice that the largest number is now in the root Suppose we discard the root: 11 22 17 19 22 14 15 18 14 21 3 9 11 How can we fix the binary tree so it is once again balanced and left-justified? Solution: remove the rightmost leaf at the deepest level and use it for the new root 21
The heapify method I Our tree is balanced and left-justified, but no longer a heap However, only the root lacks the heap property 11 22 17 19 22 14 15 18 14 21 3 9 We can siftDown() the root After doing this, one and only one of its children may have lost the heap property 22
The heapify method II Now the left child of the root (still the number 11) lacks the heap property 22 11 17 19 22 14 15 18 14 21 3 9 We can siftDown() this node After doing this, one and only one of its children may have lost the heap property 23
The heapify method III Now the right child of the left child of the root (still the number 11) lacks the heap property: 22 22 17 19 11 14 15 18 14 21 3 9 We can siftDown() this node After doing this, one and only one of its children may have lost the heap property but it doesn t, because it s a leaf 24
The heapify method IV Our tree is once again a heap, because every node in it has the heap property 22 22 17 19 21 14 15 18 14 11 3 9 Once again, the largest (or a largest) value is in the root We can repeat this process until the tree becomes empty This produces a sequence of values in order largest to smallest 25
Analysis To reheap the root node, we have to follow one path from the root to a leaf node (and we might stop before we reach a leaf) The binary tree is perfectly balanced Therefore, this path is O(log n) long And we only do O(1) operations at each node Therefore, reheaping takes O(log n) times Since we reheap inside a while loop that we do n times, the total time for the while loop is n*O(log n), or O(n log n) 26
Heap-Sort Consider a priority queue with n items implemented by means of a heap the space used is O(n) methods insertItem and removeMax take O(log n) time methods size, isEmpty, maxKey, and maxElement take time O(1) time Using a heap-based priority queue, we can sort a sequence of n elements in O(n log n) time The resulting algorithm is called heap-sort Heap-sort is much faster than quadratic sorting algorithms, such as insertion-sort and selection-sort
Exercise: Heap-Sort Heap-sort is the variation of PQ-sort where the priority queue is implemented with a heap (first n insertItems, then n removeMins) 4 5 2 3 1 Illustrate the performance of heap-sort on the following input sequence: (22, 15, 36, 44, 10, 3, 9, 13, 29, 25)
Analysis Construct the heap O(n log n) Remove and re-heap Do this n times O(log n) O(n log n) Total time O(n log n) + O(n log n) 29