Java Method Parameters
Learn about passing parameters in Java methods, including implicit and explicit parameters, formal parameters, and actual parameters. Understand the relationship between formal and actual parameters and how values are passed during method calls. Explore the concept of parameter passing and its implications on method execution in Java programming.
Download Presentation
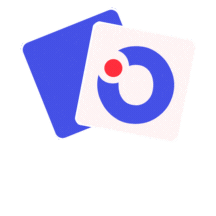
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Primary Models for Writing Code It will be helpful to learn a little more terminology about passing parameters and to discuss their use more specifically. Here is one of the primary models for writing code in Java: object.method(parameters);
Method Example The object is referred to as the implicit parameter, and any variable declared inside the parentheses is an explicit parameter. More than one parameter may be declared inside the parentheses: public void myMethod(double x, double y) { }
Method Example, cont. The variables x and y, declared in the method definition, can be referred to as formal parameters. This terminology means that they are placeholders for values which they will receive at run time. These variables are active when the method code is being run.
Method Example, cont. Their names are valid between the braces enclosing the method code, like the names of variables that are declared within those braces. When the method call is finished, whether the method returns anything or not, the formal parameter variables do not retain any values.
Clarification about methods There is only one copy of the code for each method of a class. Each object gets its own copies of the instance variables but the method code is shared by all objects of that class that are in existence. As time passes, different objects may call the same method. However, no parameter value from a previous call will be retained when a subsequent call to the method is made.
Parameter Passing In a program, the call of the method above would take this form: double q, r; object.myMethod(q, r);
Parameter Passing, cont. The variables q and r are referred to as actual parameters. In the program they are given values which are then passed to the method during the call. These actual parameters should be of the same type as the formal parameters declared in the method. From the point of view of the variables, the first thing that happens in the execution of the method call is the following: The value in the actual parameter q is copied into the formal parameter x. The value in the actual parameter r is copied into the formal parameter y.
Parameter Passing, cont. Parameter passing is like an assignment that crosses the boundary between the program and the method. There is no connection between q and x, and r and y except for the copying of values from one to another. No changes to x and y in the method have any effect on q and r. At the end of the call, when the method returns, the values in x and y are lost. The values of q and r in the calling program remain unchanged. If it is desirable to obtain a result from a method, it is necessary to write the method to return that result.
Parameter Passing, cont. It is important to remember that the parameters for these examples are numeric, ints and doubles. Everything that is said here applies to simple parameters that are of simple types. This is important because other parameter types work in different ways. This topic will be pursued in the following section.
Parameter Passing, cont. Because the copying of actual parameter values to the formal parameters is like an assignment, the actual parameters do not always have to be of exactly the same type as the formal parameters. It is sufficient that the actual parameters be of a type that can be successfully assigned to the formal parameters. For example, it is possible to assign an integer value to a double variable without casting.
Parameter Passing, cont. That means that an integer parameter value could be passed to a double formal parameter without problems. If the actual parameter value cannot be assigned to the formal parameter without casting, the compiler will give an error message.
Something confusing Here is something that sometimes confuses beginning programmers. Suppose that the formal parameters were declared as follows: public void myMethod(double x, double y) { }
Something confusing, cont. Then consider the following declarations and call in a program: double x, y; object.myMethod(x, y);
Something confusing, cont. Is there any relationship between the x and y in the program and the x and y in the method? The only relationship is this: When the method is executed, the value of the x in the program is copied to the x in the method, and the value of the y in the program is copied to the y in the method. There are two different variables x with the same name and two different variables y with the same name.
Something confusing, cont. They are different from each other because they are declared within different sets of braces, and for this reason the system can tell them apart. Changes to x or y in the method code still have no effect on x or y in the calling code.
Another source of confusion public class CupX { } There is a related issue that sometimes confuses beginning programmers. Consider the following class code. It will compile, but it is wrong: private int seedCount; public CupX() { } seedCount = 0; public int getSeedCount() { return seedCount; } /* NO! NO! NO! */ public void setSeedCount(int seedCount) { seedCount = seedCount; }
Another source of confusion, cont. The problem lies in the assignment statement in the setSeedCount() method: seedCount = seedCount. /* NO! NO! NO! */ public void setSeedCount(int seedCount) { seedCount = seedCount; }
Another source of confusion, cont. public class TestCupX { public static void main(String[] args) { CupX myCup = new CupX(); myCup.setSeedCount(5); int result = myCup.getSeedCount(); System.out.println(result); } } Consider what would happen when the following program, which tests the class, is run: This program will give 0 as output. When the cup object is constructed, seedCount is set to 0.
Another source of confusion, cont. The call to setSeedCount() has no effect. It is possible to explain why this is the case. The instance variable seedCount is declared at the top of the set of braces containing the class definition. It is in existence within that set of braces. The formal parameter seedCount is defined in the parentheses at the beginning of the definition of the setSeedCount() method.
Another source of confusion, cont. It is in existence within the set of braces containing that definition. The system will not complain that there are two different variables named seedCount because they are distinct. Within the method, the variable named seedCount is the parameter. Anywhere else in the class definition, the variable named seedCount is the instance variable.
Another source of confusion, cont. The line of code in the method, seedCount = seedCount, only involves the parameter. It does not affect the instance variable of the same name. When the example program is run, in the method code the current value of the parameter seedCount, 5, is assigned to the parameter again. The instance variable, which contains the value 0, is unchanged by the execution of the method.
Another source of confusion, cont. The system can keep track of the variables with the same names and distinguish where each is valid. However, the class code given above is both useless and misleading to a programmer because it suggests that it does something that it doesn t.
Actual Parameters, Formal Parameters, and Instance Variables To sum up the foregoing, actual parameters and formal parameters can have the same names, while formal parameters and instance variables cannot have the same names. You might also wonder whether actual parameters and instance variables can have the same names. The answer is yes, because the system can distinguish between them.
Actual Parameters, Formal Parameters, and Instance Variables, cont. Actual parameters are declared and exist within the braces of the calling program. Instance variables are declared and exist within the braces of the class definition. You may wish to use different names so that you don t get confused. However, for example, the following would be perfectly good code in a calling program: int seedCount = 5; myCup.setSeedCount(seedCount);
Constructors and their formal parameters There is one more topic related to the names and types of formal parameters. We have seen that it is possible to have more than one constructor for the same class, and the names of the constructors are the same. The system distinguishes between them based on the parameter list. A class can also have more than one method with the same name. The types and sequence of the formal parameters allow the system to distinguish between the different constructors and methods.
Constructors and their formal parameters, cont. The names of the formal parameters don t allow the system to distinguish between different constructors with the same name or different methods with the same name. For example you could not have two different methods in a single class with parameter lists like these: public void myMethod(double x, int y) public void myMethod(double v, int w)
Constructors and their formal parameters, cont. The system would detect an error. The names of the formal parameters are different, but each method of the same name has a parameter list containing one double and one int, in that order. On the other hand, it would be possible to have two methods such as these: public void myMethod(int x, double y) public void myMethod(double x, int y)
Constructors and their formal parameters, cont. Alternatively, it would also be possible to have two methods such as these: public void myMethod(int x, double y) public void myMethod(double y, int x)
Constructors and their formal parameters, cont. In both cases the system notes that the first method has a parameter list consisting of an int followed by a double, while the second method has a double followed by an int. This is all that matters. The names of the formal parameters, x and y, do not. Clearly there are limitations to allowing different methods to have the same name and distinguishing them by their parameter lists.
Constructors and their formal parameters, cont. The important thing to grasp is that having distinct names is important for instance variables and formal parameters. However, it is not possible to distinguish between two methods of the same name simply on the basis of distinct formal parameter names.