Introduction to C/C++ History and Basics
This material covers the history, syntax, and basics of C/C++. Learn about compiled vs. interpreted languages, the development of C and C++, and essential concepts for writing and understanding programs. Gain insights into compiled languages like C and C++, their significance, and more.
Download Presentation
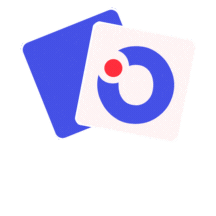
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Introduction to C/C++ Doug Sondak SCV sondak@bu.edu
Information Services & Technology 2/25/2025 Outline Goals C/C++ History Basic syntax makefiles Additional syntax 2
Information Services & Technology 2/25/2025 Goals To be able to write simple C/C++ programs To be able to understand and modify existing C/C++ code To be able to write and use makefiles 3
Information Services & Technology 2/25/2025 Compiled vs. Interpreted Languages Interpreted languages when you type something, e.g., x=y+z , it is immediately converted to machine language and executed examples: Matlab, Python advantage lots off convenient features disadvantage can be slow and memory-intensive 4
Information Services & Technology 2/25/2025 Compiled (cont d) Compiled languages examples: C, C++, Fortran source code is written using a text editor source code does nothing by itself it s just text we will use source file suffix .cpp source code must be processed through a compiler translates source code into machine language creates executable this is the code that you actually run like .exe file in Windows 5
Information Services & Technology 2/25/2025 C History Developed by Dennis Ritchie at Bell Labs in 1972 Originally designed for system software Impetus was porting of Unix to a DEC PDP-11 PDP-11 had 24kB main memory! 1978 book The C Programming Language by Kernighan & Ritchie served as standard Official ANSI standard published in 1989 Updated in 1999 6
Information Services & Technology 2/25/2025 C++ History C++ was developed by Bjarne Stroustrup at Bell Labs in 1979 implemented object-oriented features of another language, Simula, in C originally called C with Classes name changed to C++ in 1983 first commercial compiler in 1985 official standard published in 1988 7
Information Services & Technology 2/25/2025 C vs. C++ C is essentially a subset of C++ There are some convenience features in C++ that we will utilize here Since we will be writing rudimentary codes, we will not get into object-oriented C++ constructs We will use the GNU C++ compiler g++ 8
Information Services & Technology 2/25/2025 Types of Variables each variable and value has a type some common types int short for integer number with no decimal places 1, 857436 float short for floating-point number with decimal places 1.234, 4.0 9
Information Services & Technology 2/25/2025 Types of Variables (cont d) char short for character enclosed in single quotes x , $ character string is string of chars enclosed in double quotes This is a character string. 10
IS&T Organization 2/25/2025 C/C++ Syntax Case-sensitive Spaces don t matter except within character strings I use them liberally to make code easy to read Source lines end with semicolons (as in Matlab) Comments notes for humans that are ignored by the compiler C: enclosed by /* */ C++: // at beginning of comment many C compilers also accept this syntax Use them liberally! 11
IS&T Organization 2/25/2025 C/C++ Syntax (cont d) source code contains functions each one performs some task you write some of them some are intrinsic to the language every code contains at least one function, called main functions often, though not always, return a value function is characterized by the type of value it returns int, float, char, etc. if function does not return anything, we will declare (characterize) it as an int function 12
Information Services & Technology 2/25/2025 C/C++ Syntax (3) functions may, but do not have to, take arguments arguments are input values to the function code blocks, including entire functions, are enclosed within curly braces { } main function is defined in source code as follows: type declaration function name (we have no arguments here but still need parentheses) function arguments int main( ) { function statements } 13
Information Services & Technology 2/25/2025 C/C++ Syntax (4) Style note: some people like to arrange the brackets like int main( ) { function statements } Either way is fine Be consistent! 14
Information Services & Technology 2/25/2025 C/C++ Syntax (5) cout is a stream that is used to direct output to the screen, e.g. cout << my string ; sends the string to cout, i.e., to the screen The above syntax does not include a carriage return at the end of the line. We can add the carriage return with: cout << my string << endl; 15
Information Services & Technology 2/25/2025 C/C++ Syntax (6) some program elements (built-in functions, variables, etc.) are contained in header files to use these program elements you need to include the appropriate header files in your source code in C, header files have .h suffixes may or may not have .h suffix in C++ syntax for inclusion of header files: #include <header_file_name> Included before function definition < and > are part of the syntax Note that the #include statement does not end with a ; 16
Information Services & Technology 2/25/2025 C/C++ Syntax (7) C++ (but not C) has a feature, namespace, that defines packages of functions, etc. This is fairly new, and you might not see it in older C++ programs The cout stream is defined in the iostream header file, which is part of the std namespace The syntax to use the std namespace is using namespace std; 17
Information Services & Technology 2/25/2025 Exercise 1 Write a hello world program in an editor Program should print a character string General structure of code, in order: include the file iostream not iostream.h, just iostream use the std namespace define main function use cout and endl to print string to screen Save it to the file name hello.cpp solution 18
Information Services & Technology 2/25/2025 Compilation A compiler is a program that reads source code and converts it to a form usable by the computer Code compiled for a given type of processor will not generally run on other types AMD and Intel are compatible We ll use g++, since it s free and readily available 19
Information Services & Technology 2/25/2025 Compilation (cont d) Compilers have numerous options See gcc compiler documentation at http://gcc.gnu.org/onlinedocs/ gcc refers to the GNU compiler collection, which includes the C compiler (gcc) and the C++ compiler (g++) For now, we will simply use the o option, which allows you to specify the name of the resulting executable 20
Information Services & Technology 2/25/2025 Compilation (3) In a Unix window: g++ o hello hello.cpp hello is name of executable file (compiler output) hello.cpp is source file name (compiler input) Compile your code If it simply returns a Unix prompt it worked If you get error messages, read them carefully and see if you can fix the source code and re-compile 21
Information Services & Technology 2/25/2025 Compilation (4) Once it compiles correctly, type hello at the Unix prompt, and it will run the program should print the string to the screen 22
Information Services & Technology 2/25/2025 Declarations different variable types (int, float, etc.) are represented differently internally different bit patterns must tell compiler the type of every variable by declaring them example declarations: int i, jmax, k_value; float xval, elapsed_time; char aletter, bletter; 23
Information Services & Technology 2/25/2025 Arithmetic +, -, *, / No power operator (see next bullet) Math functions in math.h pow(x,y) raises x to the y power sin, acos, tanh, exp, sqrt, etc. for some compilers, need to add lm flag to compile command to access math library Exponential notation indicated by letter e 4.2e3 Goodpractice to use decimal points with floats, e.g., x = 1.0 rather than x = 1 2 . 4 3 10 24
Information Services & Technology 2/25/2025 Arithmetic (cont d) ++ and -- operators these are equivalent: i = i+1; i++; always increments/decrements by 1 += these are equivalent: x = x + 46.3*y; x += 46.3*y; 25
Information Services & Technology 2/25/2025 Arithmetic (3) Can convert types with cast operator float xval; int i, j; xval = (float) i / (float) j; Pure integer arithmetic truncates result! 5/2 = 2 2/5 = 0 26
Information Services & Technology 2/25/2025 Exercise 2 Write program to convert a Celcius temperature to Fahrenheit and print the result. Hard-wire the Celcius value to 100.0 We ll make it an input value in a subsequent exercise Don t forget to declare all variables F = (9/5)C + 32 solution 27
Information Services & Technology 2/25/2025 cin cin reads input from the screen cin >> var; Note: >> rather than << as with cout Writes input value to variable var Often use cout and cin to prompt for a value: cout << Enter value: ; cin >> x; 28
Information Services & Technology 2/25/2025 Exercise 3 Modify Celcius program to read value from keyboard Prompt for Celcius value using cout Read value using cin Rest can remain the same as last exercise solution 29
Information Services & Technology 2/25/2025 Arrays Can declare arrays using [ ] float x[100]; char a[25]; Array indices start at zero Declaration of x above creates locations for x[0] through x[99] Multiple-dimension arrays are declared as follows: int a[10][20]; 30
Information Services & Technology 2/25/2025 Arrays (cont d) Character strings (char arrays) always end with the character \0 You usually don t have to worry about it as long as you dimension the string 1 larger than the length of the required string char name[5]; name = Fred ; works char name[4]; name = Fred ; doesn t work 31
Information Services & Technology 2/25/2025 For Loop for loop repeats calculation over range of indices for(i=0; i<n; i++){ a[i] = sqrt( pow(b[i],2) + pow(c[i],2) ); } for statement has 3 parts: initialization completion condition what to do after each iteration 32
Information Services & Technology 2/25/2025 Exercise 4 Write program to: declare two vectors of length 3 prompt for vector values calculate dot product print the result solution n = c a b i i =1 i 33
Information Services & Technology 2/25/2025 Pointers Memory is organized in units of words Word size is architecture-dependent Pentium: 4 bytes Xeon, Itanium: 8 bytes Each word has a numerical address 32 16 8 0 34
Information Services & Technology 2/25/2025 Pointers (cont d) When you declare a variable, a location of appropriate size is reserved in memory When you set its value, the value is placed in that memory location float x; x = 3.2; 32 16 3.2 8 0 address 35
Information Services & Technology 2/25/2025 Pointers (3) A pointer is a variable containing a memory address Declared using * prefix float *p; Address operator & Address of specified variable float x, *p; p = &x; 36
Information Services & Technology 2/25/2025 Pointers (4) float x, *p; p = &x; 1056 32 p 1052 16 16 1048 8 1040 0 address address 37
Information Services & Technology 2/25/2025 Pointers (5) Depending on context, * can also be the dereferencing operator Value stored in memory location pointed to by specified pointer *p = 3.2; Common newbie error float *p; *p = 3.2; Wrong! p doesn t have value yet float x, *p; p = &x; *p = 3.2; correct
Information Services & Technology 2/25/2025 Pointers (6) The name of an array is actually a pointer to the memory location of the first element a[100] a is a pointer to the first element of the array (a[0]) These are equivalent: x[0] = 4.53; *x = 4.53; 39
Information Services & Technology 2/25/2025 Pointers (7) If p is a pointer and n is an integer, the syntax p+n means to advance the pointer by n memory locations These are therefore equivalent: x[4] = 4.53; *(x+4) = 4.53; 40
Information Services & Technology 2/25/2025 Pointers (8) In multi-dimensional arrays, values are stored in memory with last index varying most rapidly (a[0][0], a[0][1], ) Opposite of Matlab and Fortran The two statements in each box are equivalent for an array declared as int a[20][20]: a[0][17] = 1; a[1][0] = 5; *(a+17) = 1; *(a+20) = 5; 41
Information Services & Technology 2/25/2025 sizeof Some functions require size of something in bytes A useful function sizeof(arg) The argument arg can be a variable, an array name, a type Returns no. bytes in arg float x, y[5]; sizeof(x) ( 4) sizeof(y) (20) sizeof(float) ( 4) 42
Information Services & Technology 2/25/2025 Dynamic Allocation Suppose you need an array, but you don t know how big it needs to be until run time. Use malloc function malloc(n) n is no. bytes to be allocated returns pointer to allocated space lives in stdlib.h 43
Information Services & Technology 2/25/2025 Dynamic Allocation (cont d) Declare pointer of required type float *myarray; Suppose we need 101 elements in array malloc requires no. bytes, cast as appropriate pointer myarray = (float *) malloc(101*sizeof(float)); free releases space when it s no longer needed: free(myarray); 44
Information Services & Technology 2/25/2025 Exercise 5 Modify dot-product program to handle vectors of any length Prompt for length of vectors Read length of vectors from screen Dynamically allocate vectors Don t forget to include stdlib.h so you have access to the malloc function solution 45
Information Services & Technology 2/25/2025 if/else if/else Conditional execution of block of source code Based on relational operators < less than > greater than == equal <= less than or equal >= greater than or equal != not equal && and || or 46
Information Services & Technology 2/25/2025 if/else if/else (cont d) if( x > 0.0 && y > 0.0 ){ z = 1.0/(x+y); }else if( x < 0.0 && y < 0.0){ z = -1.0/(x+y); }else{ printf( Error condition\n ); } 47
Information Services & Technology 2/25/2025 if/else if/else (3) Can use if without else if( x > 0.0 && y > 0.0 ){ printf( x and y are both positive\n ); } 48
Information Services & Technology 2/25/2025 Exercise 6 In dot product code, check if the magnitude of the dot product is less than using the absolute value function fabsf. If it is, print a warning message. With some compilers you would need to include math.h for the fabsf function With some compilers you would need to link to the math library by adding the flag lm to the end of your compile/link command solution 10 6 49
Information Services & Technology 2/25/2025 Functions Function returns a single item (number, array, etc.) Return type must be declared Argument types must be declared Sample function definition: float sumsqr(float x, float y){ float z; z = x*x + y*y; return z; } 50