Introduction to Animation and Graphics
This lecture covers various topics such as animation, graphics, and drawing, providing an overview of different types of animations like property animation, view animation, and drawable animation. It delves into techniques for changing values and properties over time using tools like ValueAnimator and ObjectAnimator. Additionally, it discusses how to animate views through scaling, rotating, and translating, along with defining animations in XML for implementation. Explore references related to Android graphics and get started with creating captivating animations and visuals.
Download Presentation
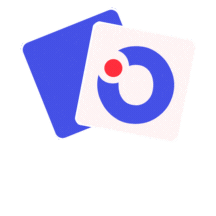
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Lecture 3: Animation & Graphics Lecture 3: Animation & Graphics Topics: Animation, Graphics, Drawing Date: Jan 31, 2016
References (study these) References (study these) http://developer.android.com/guide/topics/graphics/overview.html http://developer.android.com/guide/topics/graphics/prop-animation.html http://developer.android.com/guide/topics/graphics/view-animation.html http://developer.android.com/guide/topics/graphics/drawable-animation.html http://developer.android.com/guide/topics/graphics/2d-graphics.html
High Five! High Five! https://youtu.be/uFpoXq73HHY
Animation Overview Animation Overview 1. Property Animation 2. View Animation 3. Drawable Animation
1. Property Animation (Value) 1. Property Animation (Value) Changing value of a variable over a period: ValueAnimator anim = ValueAnimator.ofFloat(0f, 40f); anim.setDuration(40); anim.start(); Use setInterpolator() to change behavior. Limitation?
1. Property Animation (Object) 1. Property Animation (Object) Changing a property of an object. Hello World Hello World Hello World ObjectAnimator anim = ObjectAnimator.ofFloat(myTextView, textSize , 10f, 30f); anim.setDuration(5000); anim.start(); Object Variable
2. View Animation 2. View Animation You can animate a View e.g., by scaling, rotating, translating an ImageView.
2. View Animation 2. View Animation Define the Animation in XML: res/anim <?xml version="1.0" encoding="utf-8"?> <rotate xmlns:android="http://schemas.android.com/apk/res/android" android:fromDegrees="0" android:toDegrees="360" android:toYScale="0.0" android:pivotX="50%" android:pivotY="50%" android:startOffset= 1000" android:duration="10000" /> Use Animation to fetch it, then apply it to a View. Animation x = AnimationUtils.loadAnimation( getApplicationContext(), R.anim.abovexmlfile ); someView.startAnimation(x);
3. 3. Drawable Drawable Animation We can load and display a series of Drawable resources (e.g. images) one after another. Animation
3. 3. Drawable Drawable Animation Define animation-list in XML: res/drawable Animation <?xml version="1.0" encoding="utf-8"?> <animation-list xmlns:android="http://schemas.android.com/apk/res/android" android:oneshot= false"> <item android:drawable="@drawable/pic1" android:duration= 1000" /> <item android:drawable="@drawable/pic2" android:duration= 1000" /> <item android:drawable="@drawable/pic1" android:duration= 1000" /> <item android:drawable="@drawable/pic2" android:duration= 1000" /> </animation-list> Use AnimationDrawable inside your code someImgView.setBackgroundResource(R.drawable.abovexml); ((AnimationDrawable)someImgView.getBackground()).start();
Code Practice: Code Practice: Get a few images and copy: res/drawable/ Create an XML file: res/drawable/my_anim_list Add an ImageView and set Background Use AnimationDrawable to start animation
Graphics Overview Graphics Overview Canvas and Drawables Hardware Acceleration (think GPU) OpenGL (framework API and NDK) drawRect() drawRect() drawCircle() Bitmap Canvas
2D Drawing 2D Drawing 1. Drawable: Put Drawables (into a View) Less work, less control, less efficiency 2. Canvas: Draw on the Canvas (of a View) More work, more control, more efficiency
1. Using 1. Using Drawables Drawables Putting Drawables into a View Four ButtonViews res/drawable/Queen.png res/drawable/tfade.xml
1. Using 1. Using Drawables Drawables android.graphics.drawable BitmapDrawable, Transition Drawable, Animation Drawable, ShapeDrawable, etc. Two ways to add Drawables: a) Image from Project Resource b) XML file defining Drawable properties
1(a) Image from Project Resource 1(a) Image from Project Resource Copy images into res/drawable/ Use it inside the layout xml <ImageView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/img1" android:src="@drawable/my_image"/> You can also do the same by writing code: ImageView i = new ImageView(this); i.setImageResource(R.drawable.my_image); LinearLayout ll = new LinearLayout(this); ll.addView(i); setContentView(ll); //instead of setContentView(R.layout.somexmllayoutfile)
1(b) XML with 1(b) XML with drawable e.g. Transition Drawable: res/drawable/something.xml drawable properties properties <transition xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/image1"> <item android:drawable="@drawable/image2"> </transition> Now, take an ImageView to show it (them): TransitionDrawable td; td = (TransitionDrawable) getResources().getDrawable(R.drawable.something); td.setCrossFadeEnabled(true); ImageView img; img = (ImageView) findViewById(R.id.img1); img.setImageDrawable(td); td.startTransition(5000);
Nine Patch Image Nine Patch Image Regular .png images + defining stretchable regions From a terminal: Run the draw9patch command from your SDK sdk/tools directory to launch the tool.
2. Using Canvas 2. Using Canvas Canvas holds all of your draw*() calls. Drawing is performed upon an underlying Bitmap. Bitmap b = Bitmap.createBitmap(100, 100, Bitmap.Config.ARGB_8888); Canvas c = new Canvas(b); Paint p = new Paint(); p.setColor(Color.GREEN); c.drawRect(10, 10, 90, 90, p); Two ways to use the Canvas of a View: Custom View Surface View
2(a) Canvas of a Custom View 2(a) Canvas of a Custom View Good for low frame-rate applications (e.g. chess or snake game). You define a new View and add it in the layout XML file (like you do for a TextView, ImageView etc.) Android provides you the Canvas as you extend a View and override its onDraw() method. To request a redraw, use: invalidate(). Outside main Activity s thread, use postInvalidate().
2(a) Canvas of a Custom View 2(a) Canvas of a Custom View Create your own View Class (CustomView.java) public class CustomView extends View { //Declare all four types of constructors here. @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); //Use canvas.draw*() } } Use the View in the layout xml <edu.unc.nirjon.projectname.CustomView android:id="@+id/mycustomview" android:layout_width="match_parent" android:layout_height="match_parent" /> To force a redraw: invalidate()
Code Practice: Code Practice: Create 2 Buttons: Up and Down Create a Custom View Draw a circle at location (X, Y) Every time the buttons are clicked, the point will move. (Hint: use invalidate() to force redraw).