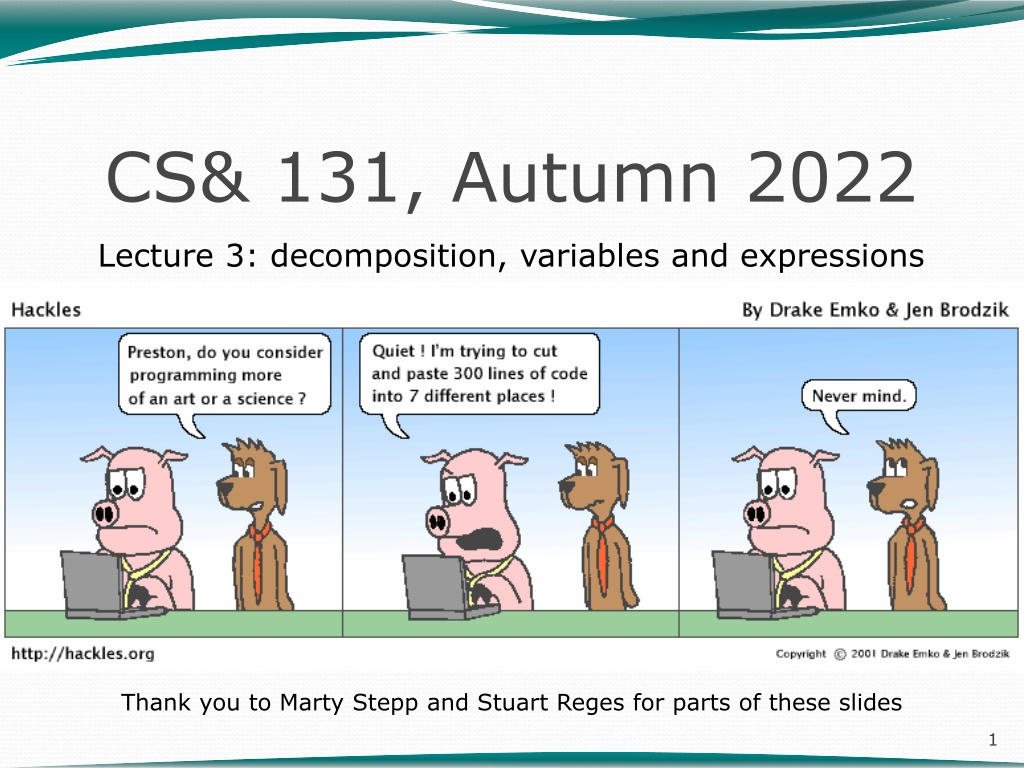
Decomposition and Functions in Programming
Explore the concept of decomposition in programming through the use of functions to create various figures in a structured manner. Learn how to approach program development from unstructured to structured versions, identifying and organizing the output structure based on different figures such as an egg, teacup, stop sign, and hat. Dive into the development strategies and program versions presented in the slides for a comprehensive understanding of programming concepts.
Download Presentation
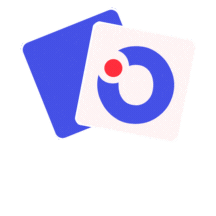
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
CS& 131, Autumn 2022 Lecture 3: decomposition, variables and expressions Thank you to Marty Stepp and Stuart Reges for parts of these slides 1
Functions question Write a program to print these figures using functions. ______ / \ / \ \ / \______/ \ / \______/ +--------+ ______ / \ / \ | STOP | \ / \______/ ______ / \ / \ +--------+ 2
Development strategy ______ / \ / \ \ / \______/ First version (unstructured): Create an empty program and main function. \ / \______/ +--------+ Copy the expected output into it, surrounding each line with printf syntax. ______ / \ / \ | STOP | \ / \______/ Run it to verify the output. ______ / \ / \ +--------+ 3
Program version 1 int main() { printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("\\ /\n"); printf(" \\______/\n"); printf("\n"); printf("\\ /\n"); printf(" \\______/\n"); printf("+--------+\n"); printf("\n"); printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("| STOP |\n"); printf("\\ /\n"); printf(" \\______/\n"); printf("\n"); printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("+--------+\n"); } 4
Development strategy 2 ______ / \ / \ \ / \______/ Second version (structured, with redundancy): Identify the structure of the output. \ / \______/ +--------+ Divide the main function into functions based on this structure. ______ / \ / \ | STOP | \ / \______/ ______ / \ / \ +--------+ 5
Output structure ______ / \ / \ \ / \______/ The structure of the output: initial "egg" figure second "teacup" figure third "stop sign" figure fourth "hat" figure \ / \______/ +--------+ ______ / \ / \ | STOP | \ / \______/ This structure can be represented by functions: egg tea_cup stop_sign ______ / \ / \ +--------+ hat 6
Program version 2 void egg() { printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("\\ /\n"); printf(" \\______/\n"); printf("\n"); } void tea_cup() { printf("\\ /\n"); printf(" \\______/\n"); printf("+--------+\n"); printf("\n"); } ... 7
Program version 2, cont'd. ... void stop_sign() { printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("| STOP |\n"); printf("\\ /\n"); printf(" \\______/\n"); printf("\n"); } void hat() { printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); printf("+--------+\n"); } int main() { egg(); tea_cup(); stop_sign(); hat(); } 8
Development strategy 3 ______ / \ / \ \ / \______/ Third version (structured, without redundancy): Identify redundancy in the output, and create fubctions to eliminate as much as possible. \ / \______/ +--------+ Add comments to the program. ______ / \ / \ | STOP | \ / \______/ ______ / \ / \ +--------+ 9
Output redundancy ______ / \ / \ \ / \______/ The redundancy in the output: egg top: egg bottom: divider line: reused on stop sign, hat reused on teacup, stop sign used on teacup, hat \ / \______/ +--------+ ______ / \ / \ | STOP | \ / \______/ This redundancy can be fixed by functions: egg_top egg_bottom ______ / \ / \ +--------+ line 10
Program version 3 // Suzy Student, CS& 138, Spring 2094 // Prints several figures, with functions for structure and redundancy. // Draws a line of dashes. void line() { printf("+--------+\n"); } // Draws the top half of an an egg figure. void egg_top() { printf(" ______\n"); printf(" / \\\n"); printf("/ \\\n"); } // Draws the bottom half of an egg figure. void egg_bottom() { printf("\\ /\n"); printf(" \\______/\n"); } // Draws a complete egg figure. void egg() { egg_top(); egg_bottom(); printf("\n"); } ... 11
Program version 3, cont'd. ... // Draws a teacup figure. void tea_cup() { egg_bottom(); line(); printf("\n"); } // Draws a stop sign figure. void stop_sign() { egg_top(); printf("| STOP |\n"); egg_bottom(); printf("\n"); } // Draws a figure that looks sort of like a hat. void hat() { egg_top(); line(); } int main() { egg(); tea_cup(); stop_sign(); hat(); } 12
Data types Internally, computers store everything as 1s and 0s 104 01101000 "hi" 0110100001101001 h 01101000 How are h and 104 differentiated? type: A category or set of data values. Constrains the operations that can be performed on data Many languages ask the programmer to specify types Examples: integer, real number, string 13
C's types We will start with these and discuss more later: Name Description integers real numbers single text characters logical values Examples 42, -3, 0, 926394 int double char bool (up to 231 - 1 usually) (up to 15 decimal places)3.1, -0.25, 9.4e3 'a', 'X', '?', '\n' true, false Why does C distinguish integers vs. real numbers? 14
Integer or real number? Which category is more appropriate? integer (int) real number (double) 1. Temperature in degrees Celsius 2. The population of lemmings 3. Your grade point average 4. A person's age in years 5. A person's weight in pounds 6. A person's height in meters 7. Number of miles traveled 8. Number of dry days in the past month 9. Your locker number 10. Number of seconds left in a game 11. The sum of a group of integers 12. The average of a group of integers credit: Kate Deibel, http://www.cs.washington.edu/homes/deibel/CATs/ 15
Expressions expression: A value or operation that computes a value. Examples: 1 + 4 * 5 (7 + 2) * 6 / 3 42 The simplest expression is a literal value. A complex expression can use operators and parentheses. 16
Arithmetic operators operator: Combines multiple values or expressions. + - * / % addition subtraction (or negation) multiplication division modulus (a.k.a. remainder) As a program runs, its expressions are evaluated. 1 + 1 evaluates to 2 3 * 4 evaluates to 12 17
Integer division with / When we divide integers, the quotient is also an integer. 14 / 4 is 3, not 3.5 3 4 52 4 ) 14 10 ) 45 27 ) 1425 12 40 135 2 5 75 54 21 More examples: 32 / 5 is 6 84 / 10 is 8 156 / 100 is 1 Dividing by 0 causes undefined behavior. Don't do this! 18
Integer remainder with % The % operator computes the remainder from integer division. 14 % 4 is 2 218 % 5 is 3 3 43 4 ) 14 5 ) 218 12 20 2 18 15 3 What is the result? 45 % 6 2 % 2 8 % 20 11 % 0 Applications of % operator: Obtain last digit of a number: Obtain last 4 digits: See whether a number is odd: 7 % 2 is 1, 42 % 2 is 0 230857 % 10 is 7 658236489 % 10000 is 6489 19
Precedence precedence: Order in which operators are evaluated. Generally operators evaluate left-to-right. 1 - 2 - 3 is (1 - 2) - 3 which is -4 But */% have a higher level of precedence than +- 1 + 3 * 4 is 13 6 + 8 / 2 * 3 6 + 4 * 3 6 + 12 is 18 Parentheses can force a certain order of evaluation: (1 + 3) * 4 is 16 Spacing does not affect order of evaluation 1+3 * 4-2 is 11 20
Precedence examples 1 * 2 + 3 * 5 % 4 \_/ | 2 + 3 * 5 % 4 \_/ | 2 + 15 % 4 \___/ | 2 + 3 \________/ | 5 1 + 8 % 3 * 2 - 9 \_/ | 1 + 2 * 2 - 9 \___/ | 1 + 4 - 9 \______/ | 5 - 9 \_________/ | -4 21
Precedence questions What values result from the following expressions? 9 / 5 695 % 20 7 + 6 * 5 7 * 6 + 5 248 % 100 / 5 6 * 3 - 9 / 4 (5 - 7) * 4 6 + (18 % (17 - 12)) 22
Real numbers (type double) Examples: 6.022 , -42.0 , 2.143e17 Placing .0 or . after an integer makes it a double. The operators +-*/%() all still work with double. / produces an exact answer: 15.0 / 2.0 is 7.5 Precedence is the same: () before */% before +- 23
Mixing types When int and double are mixed, the result is a double. 4.2 * 3 is 12.6 The conversion is per-operator, affecting only its operands. 2.0 + 10 / 3 * 2.5 - 6 / 4 \___/ | 2.0 + 3 * 2.5 - 6 / 4 \_____/ | 2.0 + 7.5 - 6 / 4 \_/ | 2.0 + 7.5 - 1 \_________/ | 9.5 - 1 \______________/ | 8.5 7 / 3 * 1.2 + 3 / 2 \_/ | 2 * 1.2 + 3 / 2 \___/ | 2.4 + 3 / 2 \_/ | 2.4 + 1 \________/ | 3.4 3 / 2 is 1 above, not 1.5. 25
Printing numbers printf("format string", number1, number2, ); A format string can contain placeholders to insert numbers: %d integer %f real number Examples: printf("x is %d and y is %d!\n", 3, -17); Output: x is 3 and y is -17! printf("Grade: %f", (95.1 + 71.9) / 2); Output: Grade: 83.5 26
Variables 27
Receipt example What's bad about the following code? int main() { // Calculate total owed, assuming 8% tax / 15% tip printf("Subtotal:\n"); printf("%d\n", 38 + 40 + 30); printf("Tax:\n"); printf(("%d\n", (38 + 40 + 30) * .08); printf("Tip:\n"); printf(("%d\n", (38 + 40 + 30) * .15); printf("Total:\n"); printf(("%d\n", 38 + 40 + 30 + (38 + 40 + 30) * .08 + (38 + 40 + 30) * .15); } The subtotal expression (38 + 40 + 30) is repeated So many printf statements 28
Variables variable: A piece of the computer's memory that is given a name and type, and can store a value. Like preset stations on a car stereo, or cell phone speed dial: Steps for using a variable: Declare it Initialize it Use it - state its name and type - store a value into it - print it or use it as part of an expression 29
Declaration variable declaration: Sets aside memory for storing a value. Variables must be declaredbefore they can be used. Syntax: typename; The name is an identifier. zipcode int zipcode; double my_gpa; my_gpa 30
Assignment assignment: Stores a value into a variable. The value can be an expression; the variable stores its result. Syntax: name = expression; zipcode 90210 int zipcode; zipcode = 90210; double my_gpa; myGPA = 1.0 + 2.25; my_gpa 3.25 31
Using variables Once given a value, a variable can be used in expressions: int x; x = 3; printf("x is %d\n", x); // x is 3 printf("%d\n", 5 * x - 1); // 14 You can assign a value more than once: x x 3 11 int x; x = 3; printf("%d here\n", x); // 3 here x = 4 + 7; printf("now x is %d\n", x); // now x is 11 32
Declaration/initialization A variable can be declared/initialized in one statement. Syntax: typename = value; my_gpa 3.95 double my_gpa = 3.95; int x = (11 % 3) + 12; x 14 33
Assignment and types A variable can only store a value of its own type. int x = 2.5; // ERROR: x will store 2 An int value can be stored accurately in a double variable. The value is converted into the equivalent real number. my_gpa 4.0 double my_gpa = 4; double avg = 11 / 2; avg 5.0 Why does avg store 5.0 and not 5.5 ? 35
Compiler errors You may not declare the same variable twice. int x; int x; // ERROR: x already exists int x = 3; int x = 5; // ERROR: x already exists How can this code be fixed? 36
Printing a variable's value Use printf just as we did for literal number values. double grade = (95.1 + 71.9 + 82.6) / 3.0; printf("Your grade was %f\n", grade); int students = 11 + 17 + 4 + 19 + 14; printf("There are %d students in the course.\n", students); Output: Your grade was 83.2 There are 65 students in the course. 37
logical errors A variable can't be used until it is assigned a value. int x; printf("%d", x); // ERROR: you haven't given x a value yet so whatever happens to be stored in memory will print variables will not print correctly if printf is given the wrong type. int x = 4; printf("x is %f\n", x); // ERROR: tries to read x as How can this code be fixed? a double so prints incorrectly 38
Receipt question Improve the receipt program using variables. int main() { // Calculate total owed, assuming 8% tax / 15% tip printf("Subtotal:\n"); printf("%d\n", 38 + 40 + 30); printf("Tax:\n"); printf("%d\n", (38 + 40 + 30) * .08); printf("Tip:\n"); printf("%d\n", (38 + 40 + 30) * .15); printf("Total:\n"); printf("%d\n", 38 + 40 + 30 + (38 + 40 + 30) * .08 + (38 + 40 + 30) * .15); } 39
Receipt answer int main() { // Calculate total owed, assuming 8% tax / 15% tip int subtotal = 38 + 40 + 30; double tax = subtotal * .08; double tip = subtotal * .15; double total = subtotal + tax + tip; printf("Subtotal: %d\n", subtotal); printf("Tax: %f\n", tax); printf("Tip: %f\n", tip); printf("Total: %f\n", total); } 40
printf precision %.Df real number, rounded to D digits after decimal double gpa = 3.253764; printf("your GPA is %.1f\n", gpa); printf("more precisely: %.3f\n", gpa); Output: your GPA is 3.3 more precisely: 3.254 3 41
Better receipt answer int main() { // Calculate total owed, assuming 8% tax / 15% tip int subtotal = 38 + 40 + 30; double tax = subtotal * .08; double tip = subtotal * .15; double total = subtotal + tax + tip; printf("Subtotal: %d\n", subtotal); printf("Tax: %.2f\n", tax); printf("Tip: %.2f\n", tip); printf("Total: %.2f\n", total); } 42
Names and identifiers You must give your C file a name. helloworld.c Naming convention: all lowercase (e.g. myfilename.c) identifier: A name given to an item in your program. must start with a letter or _ or $ subsequent characters can be any of those or a number legal: _myName TheCure ANSWER_IS_42 $bling$ illegal: me+u 49ers side-swipe Ph.D's it is considered good style to use all lowercase letters and separate words with underscores (_) good names: count_times, student_percent, width 43
Keywords keyword: An identifier that you cannot use because it already has a reserved meaning in C. auto break case char const continue default do double else enum extern float for goto if int long register return short signed sizeof static struct switch typedef union unsigned void volatile while 44