Concurrency and Memory Safe System Software Development
Tour of Rust programming environment in the Linux lab, exploring features like command line arguments, unit tests, and programming style guidelines. Studio tasks involve setting up environment, testing command line arguments, and learning about Rust safety features.
Download Presentation
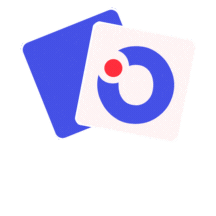
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
E81 CSE 542S: Concurrency and Memory Safe System Software Development A Tour of Rust Department of Computer Science & Engineering Chris Gill cdgill@wustl.edu 1
542S Programming Environment Rust is installed in the Linux Lab Issue command module add rust-2023 Rust cargo tool creates new packages E.g., cargo new hello creates a package named hello, with source code + metadata Rust cargo compiles+runs packages E.g., issuing cargo run within the hello directory or one of its subdirectories compiles an executable binary file, runs it 2 CSE 542S Concurrency and Memory Safe System Software Development
Command Line Arguments A much more modern interface than in C A std::env module provides an iterator over the passed command line arguments Facilitates type conversions, containers E.g., converting argument strings into numbers, storing them in a generic vector Makes it easier to do safer programming E.g., once stored in a vector, indexing is checked at compile time and run-time 3 CSE 542S Concurrency and Memory Safe System Software Development
Other Helpful Features Unit tests E.g., use #[test] attribute and functions that check assert! + assert_eq! macros Many library packages on crates.io E.g., use actix-web, serde for a web server Concurrency Multithreading with safe sharing/ownership File I/O E.g., using functions from std::fs module 4 CSE 542S Concurrency and Memory Safe System Software Development
Programming Style Guidelines Comment your code thoroughly E.g., Give // and/or /// comments for all files, functions, and non-trivial program logic Use descriptively named constants instead of hard-coded numbers E.g., const FIRST_ARG: usize = 1; Please follow the programming guidelines given for this course Will be considered in grading the labs Great idea to practice that in the studios 5 CSE 542S Concurrency and Memory Safe System Software Development
Studio 1 Set up the shell environment for this course Check for the right versions of the tools you ll be using throughout the semester Create/build/run a simple hello, world! program Examine how to capture and print out command line arguments, using iterators and vectors Return different success/failure codes from main Try out a couple different unsafe options to see how and when Rust catches and flags them Studios 1 through 11 are due by 11:59pm on the night before Exam 1 Submit as soon as each is done so you get feedback and can resubmit any that may be marked incomplete 6 CSE 542S Concurrency and Memory Safe System Software Development
A few Hints on Studio 1 BOT textbook pp. 11-14 provides some highly useful syntax and semantics E.g., use Vec::new()to declare dynamically sized vector of generic type (compiler infers) Use constants of type usize to index vectors E.g., use subranges like [FIRST_ARG..] for a slice of a vector with a subset of its elements E.g., use {} and {:?} within println! to print out single elements vs. lists respectively Also, the main function s return type can differ E.g., to wrap unsigned int in an ExitCode vs. a Result 7 CSE 542S Concurrency and Memory Safe System Software Development