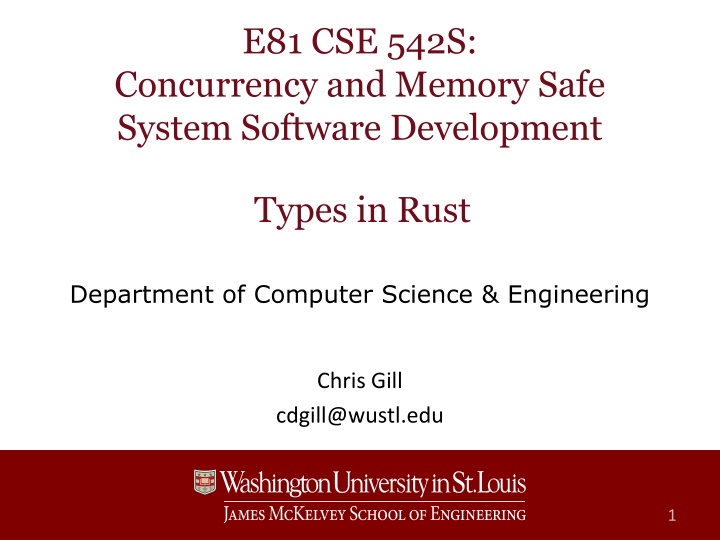
Concurrency and Memory Safe System Software Development in Rust
Discover the types in Rust programming language including fixed width numeric types, pointers, references, numeric operations, arrays, vectors, and slices. Explore Rust's characteristics and operations for developing safe and efficient system software.
Download Presentation
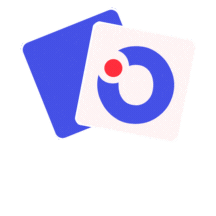
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
E81 CSE 542S: Concurrency and Memory Safe System Software Development Types in Rust Department of Computer Science & Engineering Chris Gill cdgill@wustl.edu 1
Rust Types Fixed width numeric types Signed/unsigned integers, 8 to 128 bits Floating point, 32 or 64 bits Unicode characters, bools, tuples, enums Structs, boxes, references, traits Arrays, vectors, strings, slices Options, results Pointers to functions 2 CSE 542S Concurrency and Memory Safe System Software Development
Fixed Width Numeric Types Names: letter for the type, then size in bits Integer types E.g., u8, u16, u32, u64, u128 E.g., usize has the size of a machine address E.g., i8, i16, i32, i64, i128 E.g., isize has the size of a machine address Floating point types E.g., f32 (single precision), or f64 (double) Numeric literals (type is inferred if unspecified) E.g., 2 (i32 by default), 3.1f64, -7i8, 0xbead_u32 3 CSE 542S Concurrency and Memory Safe System Software Development
Numeric Operations Rust panics if arithmetic operation overflows Can specify overflow handling explicitly Checked operations return an Option that contains either a value or None Overflowing ones return a tuple with the value the wrapped version would have produced, and a bool indicating whether overflow occurred Wrapped ones produce a value modulo a range Saturating ones return value near min or max 4 CSE 542S Concurrency and Memory Safe System Software Development
Pointers and References References (* operator to dereference) Immutable (read-only) reference type &T Mutable reference type &mut T Boxes (act like C++ scoped pointers) Box::new allocates object on the heap, which is deallocated when scope is exited Raw pointers (*mut T and *const T) Only can be dereferenced in usafe blocks 5 CSE 542S Concurrency and Memory Safe System Software Development
Arrays, Vectors, and Slices Array lengths: fixed, part of their type Use square brackets to declare/index them Can resize a vector, query its length Declare using Vec::new or vec! macro A slice grabs a region of data from an array or vector (including Strings) E.g., print(&v[1..]) prints out all of the elements of vector v after the first (0th) one 6 CSE 542S Concurrency and Memory Safe System Software Development
String Types Double-quote delimited string literals E.g., Now is the winter of our Byte strings: u8 rather than Unicode E.g., b hello, world! Strings: vectors of Unicode characters E.g., discontent made .to_string() Can use comparison operators (<= etc.) The format! macro works like println! 7 CSE 542S Concurrency and Memory Safe System Software Development
Type Aliases & User Defined Types The type keyword is like C++ typedef E.g., type Bytevec = Vec<u8> // ASCII E.g., useful for working with external code We ll cover user-defined types in subsequent lectures and studios E.g., structs are covered in BOT Chapter 9 E.g., enumerations are in BOT Chapter 10 E.g., traits are in BOT Chapter 11 8 CSE 542S Concurrency and Memory Safe System Software Development
Studio 2 Checked, overflowing, saturating, wrapping ops Use loop and for to run until overflow Use while to stop just short of overflow Push values into a vector, sort it, print it out Studios 1 through 11 are due by 11:59pm on the night before Exam 1 Submit as soon as each is done so you get feedback and can resubmit any that may be marked incomplete 9 CSE 542S Concurrency and Memory Safe System Software Development
A few Hints on Studio 2 BOT textbook pp. 142-145 shows the syntax for different kinds of looping expressions E.g., use loopto iterate forever (until a panic) E.g., use whileto iterate conditionally (n-1 times) E.g., use forto iterate fixed number of times n: note Rust ranges are right-open as in [1..n+1) Tuples are indexed using . syntax E.g., val.overflowing_mul(MULTIPLE_VALUE).1 Cannot use manifest constants, so comment that! 10 CSE 542S Concurrency and Memory Safe System Software Development