The Rust Programming Language
This presentation provides an in-depth look at The Rust Programming Language, highlighting its evolution, key features like borrow checking and generics, installation steps, and code examples. It covers essential concepts such as state-of-the-art LLVM, Rust's powerful borrow checker, and usage of standard libraries for filesystem operations. The content also includes a detailed explanation of file metadata retrieval in Rust. Dive into the world of Rust programming with this comprehensive guide.
Download Presentation
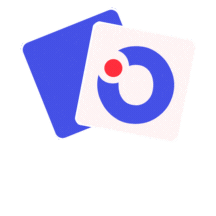
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
The Rust Programming Language Loukia Christina Ioannou Georgios Evangelou Winter 2023 1
2006 Graydon Hoare Rust 1.0 2015 ( LLVM) , C/C++ 8 Stack Overflow, 2
Unix: $ curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh Windows: rustup-init.exe https://rust- lang.org 5
Hello, world! // main.rs fn main() { println!("Hello, world!"); } : $ rustc main.rs $ ./main > Hello, world! 6
stat(1) Rust use std::env; use std::fs; use std::io::{Error, ErrorKind}; use std::os::unix::fs::{FileTypeExt, MetadataExt}; fn main() -> std::io::Result<()> { let args: Vec<String> = env::args().collect(); if args.len() != 2 { let errmsg = format!("usage: {} filename", args[0]); return Err(Error::new(ErrorKind::Other, errmsg)); } stat_print(&args[1])? Ok(()) } 7
fn stat_print(filename: &String) -> std::io::Result<()> { let md = fs::metadata(filename)?; println!("File: {}", filename); println!("Size: {}", md.len()); println!("Blocks: {}", md.blocks()); println!("IO Block: {}", md.blksize()); println!("{}", file_type_str(md.file_type())); println!("Device: {:x}h/{}d", md.dev(), md.dev()); println!("Inode: {}", md.ino()); println!("Links: {}", md.nlink()); println!("Access: {:04o}", md.mode() & 0o7777); println!("Uid: {}", md.uid()); println!("Gid: {}", md.gid()); println!("Access: {}", md.atime()); println!("Modify: {}", md.mtime()); println!("Change: {}", md.ctime()); Ok(()) } 8
fn file_type_str(ft: fs::FileType) -> &'static str { if ft.is_dir() { return "directory"; } else if ft.is_file() { return "regular file"; } else if ft.is_symlink() { return "symbolic link"; } else if ft.is_block_device() { return "block special file"; } else if ft.is_char_device() { return "character special file"; } else if ft.is_fifo() { return "fifo"; } else if ft.is_socket() { return "socket"; } else { return "unknown file type"; } } 9
'./stat' $ ./stat ./stat File: ./stat Size: 4718584 Blocks: 9216 IO Block: 4096 regular file Device: 820h/2080d Inode: 23279 Links: 1 Access: 0755 Uid: 1000 Gid: 1000 Access: 1701016966 Modify: 1701016961 Change: 1701016961 10
SMTP client Rust fn main() -> Result<(), std::io::Error> { let args: Vec<String> = std::env::args().collect(); if args.len() != 4 { eprintln!("Usage: {} emaildir smtpserver username", args[0]); return Ok(()); } let mut args = args.into_iter(); let _prog_name = args.next().unwrap(); let email_str = args.next().unwrap(); let email_dir = Path::new(&email_str); 11
let mut smtp_conn = { let smtp_server = args.next().unwrap(); let username = args.next().unwrap(); SMTPConnection::new(smtp_server, username)? }; for entry in fs::read_dir(&email_dir)? { let email = entry?.path(); if email.is_file() { smtp_conn.send_email(&email)?; } } smtp_conn.close()?; Ok(()) } 12
use std::fs::{self, File}; use std::io::{BufRead, BufReader, Error, ErrorKind, Write}; use std::net::TcpStream; use std::path::Path; struct SMTPConnection { stream: TcpStream, reader: BufReader<TcpStream>, username: String, response: String, } 13
impl SMTPConnection { pub const SMTP_PORT: u16 = 25; pub fn new(server: String, username: String) -> Result<Self, std::io::Error> { let stream = TcpStream::connect((server, Self::SMTP_PORT))?; let reader = BufReader::new(stream.try_clone()?); let mut conn = SMTPConnection { stream, reader, username, response: String::new(), }; conn.check_response(220)?; conn.write(format!("HELO {}\r\n", conn.username))?; conn.check_response(250)?; Ok(conn) } 14
fn check_response(&mut self, code: u32) -> Result<(), std::io::Error> { self.response.clear(); self.reader.read_line(&mut self.response)?; let actual = self .response .split(' ') .next() .and_then(|c| c.parse::<u32>().ok()) .unwrap(); if actual != code { Err(Error::new(ErrorKind::Other, self.response.clone())) } else { Ok(()) } } 15
pub fn send_email(&mut self, email: &Path) -> Result<(), std::io::Error> { self.write(format!("MAIL FROM: <{}>\r\n", &self.username))?; self.check_response(250)?; self.write(format!("RCPT TO: <{}>\r\n", &self.username))?; self.check_response(250)?; self.write("DATA\r\n")?; self.check_response(354)?; let lines = BufReader::new(File::open(email)?).lines(); 16
let mut header = true; for line in lines { let line = line?; if line == "\r\n" { header = false; } if !header && line.starts_with('.') { self.write(".")?; } self.write(line.replace('\n', "\r\n"))?; } self.write("\r\n.\r\n")?; self.check_response(250)?; Ok(()) } 17
fn write(&mut self, str: impl ToString) -> Result<(), std::io::Error> { self.stream.write(str.to_string().as_bytes())?; Ok(()) } pub fn close(mut self) -> Result<(), std::io::Error> { self.write("QUIT\r\n")?; self.check_response(221)?; self.stream.shutdown(std::net::Shutdown::Both)?; Ok(()) } } 18
standard library standard library (signal handling, time conversion/formatting) unix ffi libc C bash natively, `struct passwd *getpwuid(uid_t uid)` `struct group *getgrgid(gid_t gid)` 20
C borrow checker (dangling pointers, memory leaks, invalid memory accesses) bash 21
(`Optional<T>` `Result<T,E>` ) (question mark operator '?') (try catch blocks, callbacks) 22
https://survey.stackoverflow.co/2023/#section-admired-and- desired-programming-scripting-and-markup-languages https://www.rust-lang.org/ https://doc.rust-lang.org/std/index.html https://en.wikipedia.org/wiki/Rust_(programming_language) 24