Arithmetic Operations in 8086 Assembly Language
Learn how to write programs for multiplication and division using different data sizes in 8086 assembly language. Explore arithmetic instructions for binary, BCD, and ASCII arithmetic, and understand the theory behind multiplication and division operations in microprocessors. Get insights into supported operands, types of instructions, and the storage of results. Dive into unsigned and signed multiplication using MUL and IMUL instructions with examples and flag implications.
Download Presentation
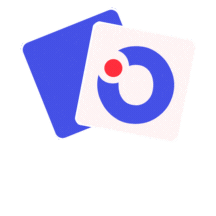
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Arithmetic Operations Multiplication Division System Programming Lab Computer Engineering Department College of Engineering
Objectives Write programs in 8086 assembly language for multiplication and division by using different size of data as bytes or words. Use arithmetic instructions to accomplish simple binary, BCD, andASCII arithmetic.
Theory Another arithmetic instructions found in any microprocessor includes multiplication and division. Also shown are their uses in manipulating register and memory data. These operations used any addressing mode with 8-bit or 16 bit. These arithmetic instructions can be divided into two types of instructions: First Group : MUL, IMUL, TEST Second group: DIV, IDIV, CBW, CWD
Theory These types of operands are supported: REG Memory REG:AX, BX, CX, DX, DI, SI, BP, SP. Memory: [BX], [BX+SI+7], variable, etc... Multiplication is performed on bytes, words, or double words, and can be signed integer or unsigned.
Theory After operation between operands, result is always stored inAL,AX. The product after a multiplication is always a double-width product. Some flag bits (overflow and carry) change when the multiply instruction executes CF, OF
Multiplication MUL unsigned multiply When operand is a byte AX = AL * operand (8 bit) When operand is a word (AX,DX) = AX * operand (8 or 16 bit) IMUL signed multiply When operand is a byte AX = AL * operand (8 bit) When operand is a word (AX,DX) = AX * operand (8 or 16 bit)
Unsigned Multiply Instr. Operands Description Algorithm: when operand is a byte: AX = AL * operand. when operand is a word: (DX AX) = AX * operand. Example: MOV AL, 200 ; AL = 0C8h MOV BL, 4 MUL BL ; AX = 0320h (800) RET Flags: C, O REG Memory MUL
Signed Multiply Instr. Operands Description Algorithm: when operand is a byte: AX = AL * operand. when operand is a word: (DX AX) = AX * operand. Example: MOV AL, -2 MOV BL, -4 IMUL BL ; AX = 8 RET Flags: C, O REG Memory IMUL
TEST Instruction Instr. Operands Description Logical ANDbetween all bits of two operands for flags only. REG , Memory Memory , REG REG , REG Memory , Immed. REG , Immed. 1 AND 1 = 1 1 AND 0 = 0 0 AND 1 = 0 0 AND 0 = 0 TEST MOV AL, 00000101b TEST AL, 1 ; ZF = 0. TEST AL, 10b ; ZF = 1. RET Flags: Z, S, P
Division DIV unsigned division When operand is a byte AL = AX / operand (8 bit) AH = remainder (modulus) When operand is a word AX = (AX,DX) / operand (8 or 16 bit) DX = remainder (modulus) IDIV signed division When operand is a byte AL = AX / operand (8 bit) AH = remainder (modulus) When operand is a word AX = (AX,DX) / operand (8 or 16 bit) DX = remainder (modulus)
Unsigned Divide Instr. Operands Description Algorithm: when operand is a byte: AL = AX / operand AH = remainder (modulus) when operand is a word: AX = (DX AX) / operand DX = remainder (modulus) Example: MOV AL, 203 ; AX = 00CBh MOV BL, 4 DIV BL ; AL = 50 (32h), AH = 3 RET Flags: NON REG Memory DIV
Signed Divide Instr. Operands Description Algorithm: when operand is a byte: AL = AX / operand AH = remainder (modulus) when operand is a word: AX = (DX AX) / operand DX = remainder (modulus) Example: MOV AX, -203 ; AX = 0FF35h MOV BL, 4 IDIV BL ; AL = -50 (0CEh), AH = -3 (0FDh) RET Flags: NON REG Memory IDIV
Convert Byte into Word Instr. Operands Description Algorithm: If high bit of AL = 1 then: AH = 255 (0FFh) else AH = 0 CBW No operands Example: MOV AX, 0 ; AH = 0, AL = 0 MOV AL, -5 ; AX = 000FBh (251) CBW ; AX = 0FFFBh (-5) RET Flags: NON
Convert Word to Double word Instr. Operands Description Algorithm: if high bit of AX = 1 then: DX = 65535 (0FFFFh) else DX = 0 Example: MOV DX, 0 ; DX = 0 MOV AX, 0 ; AX = 0 MOV AX, -5 ; DX AX = 00000h:0FFFBh CWD ; DX AX = 0FFFFh:0FFFBh RET Flags: NON CWD No operands
Examples Multiply two 8-bit unsigned numbers the first in the register BL, and the second in CL, the result is stored in DX (assumed BL=05, CL=10) ORG 100 MOV BL, 05H MOV CL, 10 MOV AL, CL MUL BL MOV DX, AX HLT
Examples Divide the unsigned byte contents of memory location NUMB by the contents of memory location NUMB1. Store the quotient in location ANSQ and reminder in location ANSR (assume [NUMB]=15 and [NUMR]=4). ORG 100 HLT MOV AL, NUMB NUMB DB 15H MOV AH, 00H NUMB1 DB 04H DIV NUMB1 ANSQ DB 0H MOV ANSQ,AL ANSR DB 0H MOV ANSR,AH
Examples Divide two 16-bit signed numbers, 1st number= -100 the 2nd = +9, store the result in DX, AX. ORG 100h After the division the results MOV AX, -100 appear in DX, AX MOV CX, 9 AX = -11 quotient CWD DX = -1 reminder IDIV CX HLT
Examples Write 8086 program that generate a byte size integer in the memory location define as RESULT, the value of integer is to be calculated from the following equation: (RESULT) = ( AL . NUM1 ) + ( NUM2 . AL ) + BL MOV AL, 01H MOV BX, 0001H MOV DL, AL ADC AX, BX MOV CL, NUM1 MOV RESULT, AX MUL CL ; ( AL . NUM1 ) HLT MOV SI, AX NUM1 DB 02h MOV AL, DL NUM2 DB 02h MUL NUM2 ; ( AL . NUM2 ) RESULT DW ? ADD AX, SI
Examples Write the program to find the value of AX=((3*A+B ))/2 ;A = 2H, B = 2H A) By using successive addition. B) By using multiplication and division instruction. MOV AL, A MOV AL, A 10+10+10 =30 30 + 6 = 36 ADD AL, AL MOV BL, 03H ADC AL, A MUL BL 36 2 2 2 2 2 2 2 2 = 16 ADC AL, B ADD AL, B SUB AL,02H MOV BL, 02H 2+2+2 =6 6 + 2 = 8 SUB AL,02H DIV BL 8 2 2 = 4 HLT HLT A DB 02H A DB 02H B DB 02H B DB 02H
Examples ??(?? ??)+ 2 Write 8086 program to compute SUM = ?=? Where Xi & Yi are signed 8-bit numbers, N=10, assume no overflow MOV SI, OFFSET X ADC DX, AX MOV DI, OFFSET Y INC BX MOV BX, 00H LOOP L1 MOV DX, 00H MOV SUM, DX MOV CX, 0BH HLT L1: X DB 11 DUP (+2) MOV AL, [SI+BX] Y DB 11 DUP (+3) IMUL [DI+BX] SUM DW ? ADD AX, 0002H