Utilizing Racket for Creative Image Rendering and File Operations
In this comprehensive guide, you will learn how to leverage Racket programming for displaying puzzles through interactive images, designing various picture functions, managing file input/output using ports, and efficiently reading and processing information from files. Explore the versatile capabilities of Racket for solving puzzles and enhancing file operations.
Download Presentation
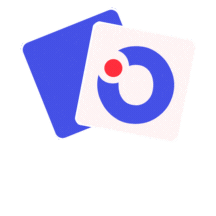
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Using Racket to Display your puzzle Pepper
More Picture Functions Pictures (must add (require picturing-programs) make filled rectangle: (rectangle length width fillMode color) ex: (rectangle 10 30 "outline" "red") or (rectangle 10 30 "solid" "black") make circle: (circle radius fillMode color) ex: (circle 30 "solid" "yellow") make picture of text: (text string font color) ex: (text "words to become pic" 12 "black") place two pictures one under the other: (above pic1 pic2) ex: (above (circle 30) (circle 50)) place two pictures next to each other: (beside pic1 pic2) ex: (beside (circle 30) (circle 50)) place one picture on top of another: (overlay overlayPic basePic) ex: (overlay (circle 30 "solid" "red") (circle 50 "outline" "black")) place one picture on top at a certain point (overlay/xy overlayPic x y basePic) ex: (overlay/xy (circle 30 "solid" "red") 30 10 (circle 50 "outline" "black"))
File Input / Output A port is similar to a file descriptor in unix open a port to connect to a new file: (define out-port (open- output-file "myfolder/tmp.txt")) open a port to connect to an existing file in prep for appending: (define out-port (open-output-file "myfolder/tmp.txt" #:exists 'append)) open a port to connect to an existing file to read it: (define in- port (open-input-file "myfolder/tmp.txt")) read from an open file : (displayln (read-line in-port)) write to the open file: (displayln "hi" out-port) close the output file : (close-output-port out-port) close the input file: (close-input-port in-port)
Reading through a file Create a function that handles one line from the file. Either split the line using a list created from the line ( string-split) or remove the end of line characters (string-replace) and use the entire line as a string. To use pictures, remember (require picturing-programs) (define ( handleline line pic) (let* ([ mystrings (string-split line ",")] [ size (first mystrings) ] [ color (list-ref mystrings 1)] [ mytext (list-ref mystrings 2)]) (above (above pic ( square (string->number size) "solid" color)) (text mytext 12 color) ; (text (string-replace line "\r" ""))) )))
Reading through the file Create a file reading function that reads a line from a file and tests whether the line is an end of file marker. If it is, print your result, otherwise, call your file reading function recursively, passing it the function that handled the line. (define in-port (open-input-file "c:/junk/tea.txt")) (define (filefunc pic) ( let ( [theline (read-line in-port )] ) ( cond [ (eof-object? theline ) pic] [ else (filefunc (handleline theline pic )) ])))