Understanding Data Types, Arithmetic Operators, and Logical Operators in Programming
Learn about data types such as integers, real numbers, booleans, characters, and strings. Explore arithmetic operators including division, floor division, and modulus. Understand relational operators for comparisons and logical operators like AND and OR for decision making in programming. Avoid confusion between assignment (=) and equality (==) operators to ensure accurate code.
Download Presentation
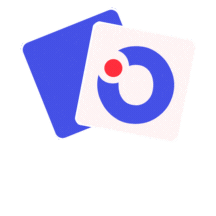
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Programming Paper 1 Data Types Arithmetic Operators Relational Operators AND OR and NOT Logical Operators Constants and Variables Thursday, 03 October 2024
Data Types Teacher Example Programming languages store data as different types Data Type Characteristics Examples Integer Whole numbers only 0, 7, 2453, -653 Real Numbers that have a decimal part 0.15, -8.46, 123.0 Boolean Only has two values True/False, 1/2, Yes/No Character A single letter, number, symbol B , g , & , 1 String A collection of characters (must use speech marks) This is a string , gfhgDtw 2 Thursday, 03 October 2024
Data Types - Students What are these data types? Problem Data Data Type 1 The apple is green 2 FALSE 3 98.23 4 * 5 34 6 S' 7 -9.00 8 23 9 No 10 @% Data Thursday, 03 October 2024
Arithmetic Operators - Teacher Division in code can sometimes result in an inaccurate value. Python has additional operators to handle these. Name Symbol Function True Division / Always returns a real value Floor Division // Rounds down to the nearest integer or real value (depending on what values you are using in your expression) Modulus % Returns the remainder of a sum Thursday, 03 October 2024
Arithmetic Operators - Students What is the result of these outcome Problem Expression Results 1 10 +70 2 3*12 3 523-89 4 60*4 5 100/25 6 250//25 7 375%25 8 50*3 9 300//45 10 6537+185-(34*25) Thursday, 03 October 2024
Relational Operators - = or == DO NOT CONFUSE = WITH == In code, one equals symbol is used to assign a value to a data type. If you want to compare the value of two data types then you must use TWO equals symbols! Thursday, 03 October 2024
Logical Operators - AND For the OR operator, only ONE of the statements have to be TRUE in order to get the key. 8>4 OR 9<20 = TRUE TRUE OR TRUE = Thursday, 03 October 2024
Logical Operators - OR For the AND operator, all of the statements have to be TRUE in order to get the key. 8>4 AND 9<20 = TRUE TRUE AND TRUE = Thursday, 03 October 2024
Logical Operators - NOT The NOT operator is an inverse operator. It creates the opposite boolean result to the statement. NOT (9==20) = TRUE TRUE (Statement) = Thursday, 03 October 2024
Logical Operators Evaluating Expressions - Students Are these expressions TRUE or FALSE? Problem Expression Results (8 > 3) AND (9 <= 21) 1 (41 != 34) OR (890 > 1925) 2 NOT (65 < 34) 3 (923 => 1425) AND (452 != 542) 4 (65 > 78) OR (34 <= 324) 5 NOT (52 => 63) 6 ((34 == 34) AND (96 <= 67)) OR ((627 < 54) AND (26 != 54)) 7 NOT ((54 > 23) OR (58 != 89)) 8 ((38 => 76) OR (84 <= 29)) AND ((37 < 62) OR (40 != 40) 9 ((41 != 34) OR (890 > 1925)) AND (NOT ((54 > 23) OR (58 != 89)) 10 Thursday, 03 October 2024
Variables and Constants Data types can be stored as variables or constants Variables and constants are boxes that we use to store values for data types. They save us time (and therefore memory) because we don t have to keep typing the same value over and over again. Using variables and constants also mean that we don't have to remember the values of data types. Thursday, 03 October 2024
Variables The value of a variable can be changed (vary) throughout your code. Constant The value of a constant is set at the beginning of your code and remains the same (constant) throughout. Thursday, 03 October 2024
Creating variables or constants is called assigning. We assign a variable or constant by using the equals symbol. This is usually done at the beginning of our code. variable_name = value CONSTANT_NAME = value Thursday, 03 October 2024
Variables - When naming variables, we always use lower case characters. int_number = 34 str_name = Sally boo_answer = TRUE c har_letter = p We can also change the value of a variable by using operators. int_num1 = 34 int_num2 = 45 int_num_total = int_num1 + int_num2 Thursday, 03 October 2024
Constants When naming constants, we always use UPPER CASE CHARACTERS characters. INT_MAXLIMIT = 100 STR_NAME1 = Bob BOO_RESULT = NO CHAR_BASE= e We can change the value of a constant at any time, but we should not really do so as this is not the point of using a constant. If we want to change the value of a data type throughout our code, then we need to use a variable. Thursday, 03 October 2024