Understanding Input and Output in Programming: A Comprehensive Guide
This content provides detailed explanations and examples on input and output handling in programming, focusing on concepts such as I/O as State, typed output, and consuming input lists. It covers topics like using print statements, scanning inputs, and building output lists in programming languages. Various code snippets and images are included to illustrate the concepts effectively.
Download Presentation
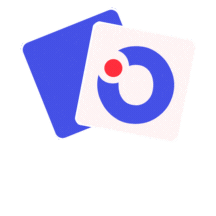
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CS 476 Programming Language Design William Mansky
Input and Output What does this code do? int main(){ printf( Hello world ); } printf ? ,?,? ?
I/O as State What does this code do? int main(){ } printf( Hello world ); printf ? ,?,?,? (skip,?,?,? "Hello world")
I/O as State: Output E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) ?,? ? print ? ,?,?,? (skip,?,?,? ?) ? builds up a list of the output produced by the program
I/O as State: Typed Output E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print_int(E E) | print_string(E E) | ? int ? string print_string(?) ok print_int(?) ok ?,? ? print_int ? ,?,?,? (skip,?,?,? ?)
I/O as State: Input E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) | x = scan() ? = scan(),?,?,? ?,? (skip,? ? ? ,?,?,?) The program consumes the list ? of input
I/O as State: Example ?: ?: print ( Enter two inputs: ) input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ?,? ? print ? ,?,?,? (skip,?,?,? ?)
I/O as State: Example ?: ?: ?: Enter print ( Enter two inputs: )?: input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ?,? ? print ? ,?,?,? (skip,?,?,? ?)
I/O as State: Example ?: ?: ?: Enter print ( Enter two inputs: )?: input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ? = scan(),?,?,? ?,? (skip,? ? ? ,?,?,?)
I/O as State: Example ?: a b ?: print ( Enter two inputs: ) input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2);
I/O as State: Example ?: a b ?: print ( Enter two inputs: )?: a b ?: Enter input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2);
I/O as State: Example ?: a b ?: print ( Enter two inputs: )?: a b ?: Enter input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ?: b ?: Enter
I/O as State: Example ?: a b ?: print ( Enter two inputs: )?: a b ?: Enter input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ?: b ?: ?: Enter ?: Enter
I/O as State: Example ?: a b ?: print ( Enter two inputs: )?: a b ?: Enter input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); ?: b ?: ?: ?: ?: ?: Enter ?: Enter ?: Enter You ?: Enter You a ?: Enter You a b
I/O as State: Example ?: ?1?2 ?: ?: Enter ?: Enter ?: Enter ?: Enter You ?: Enter You ?1 ?: Enter You ?1?2 print ( Enter two inputs: )?: ?1?2 input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); Initial state is ?,{},nil,?,nil , where ? is all the input to be read ?: ?2 ?: ?: ?: ?:
I/O as State State contains list of input to receive and output produced Program builds up output list, consumes input list Initial state is ?,{},nil,?,nil , where ? is all the input to be read Treats I/O as internal to the program What if other programs/threads are also doing I/O?
I/O as State What does this code do? int main(){ } printf( Hello world ); printf ? ,?,?,? (skip,?,?,? "Hello world")
I/O as Labels What does this code do? int main(){ } printf( Hello world ); out("Hello world")(skip,?,?) printf ? ,?,?
I/O as Labels: Syntax and Semantics E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) | x = scan() ?,? ? out(?) (skip,?,?) print ? ,?,? in(?)(skip,? ? ? ,?) ? = scan(),?,?
I/O as Labels: Semantics The behavior of a program is now a sequence of steps ?1?1,?1,?1 The sequence ?1,?2, ,?? is the external behavior of the program (sometimes called the trace) ?2 ?? ?0,?0,?0 ??,??,?? Divides I/O semantics into two separate questions: What I/O events happen in a program? What do those events mean?
I/O as Labels: Example ?0 print ( Enter two inputs: ) input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2);
I/O as Labels: Example ?0 out("Enter ")?0 print ( Enter two inputs: ) input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2);
I/O as Labels: Example ?0 out("Enter ")?0 in(?1)?0[input1 ?1] print ( Enter two inputs: ) input1 = scan(); input2 = scan(); print( You entered: ); print(input1); print(input2); Trace: out "Enter" in ?1 in ?2 out "You" out ?1 out ?2
I/O as Labels: Syntax and Semantics E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) | x = scan() ?,? ? out(?) (skip,?,?) print ? ,?,? in(?)(skip,? ? ? ,?) ? = scan(),?,?
I/O as Labels: From Labels to State in(?)(skip,? ? ? ,?) ? = scan(),?,? in ? ? ,? ,? ?,?,? ?,?,?,? ?,? (? ,? ,? ,?,?) out ? ? ,? ,? ?,?,? ?,?,?,?,? (? ,? ,? ,?,? ?)
I/O as Labels: Interaction Program: ?0 out(?1)?1 in(?2)?2 User: sees ?1 inputs ?2
I/O as Labels: Interaction Program: ?0 out(?1)?1 in(?2)?2 User: ?0 in(?1)?1 out(?2)?2 in ? out ? ,?2 ,?1 ?1,?1 (?1,?1) || (?2,?2) ?1 ?1 ?2,?2 ,?1 ?2 || ?2 ,?2
I/O as Labels: Interaction Each component produces a trace of I/O behavior When running in parallel, one component s input can be another s output The two components synchronize, stepping simultaneously and communicating a value at the same time This is synchronous message-passing concurrency, as opposed to the actor model s asynchronous message-passing Still no shared state between, e.g., program and user Examples: console I/O, network sockets, Go, CSP, pi-calculus
Interaction and Concurrency E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) | x = scan() ?,? ? out(?) (skip,?,?) print ? ,?,? in(?)(skip,? ? ? ,?) ? = scan(),?,?
Interaction and Concurrency E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | print(E E) | x = scan() ?,? ? console!? (skip,?,?) print ? ,?,? console??(skip,? ? ? ,?) ? = scan(),?,? A label is ?!? or ???, where ? is the name of a channel
Interaction and Concurrency E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | <ident> ! E E | <ident> ? <ident> ?,? ? ?!?(skip,?,?) ? !?,?,? ???(skip,? ? ? ,?) ? ??,?,? A label is ?!? or ???, where ? is the name of a channel
Interaction and Concurrency E E := <ident> | <#> | E E + E E| C C := skip | C C; C C| | <ident> ! E E | <ident> ? <ident> | C || C ?,? ? ?!?(skip,?,?) ? !?,?,? ???(skip,? ? ? ,?) ? ??,?,? A label is ?!? or ???, where ? is the name of a channel
Interaction and Concurrency ?!? ??? ,?2 ,?2 ,?2 ,?1 ,?1 (?2,?2,?2) ,?1 ?1,?1,?1 ?1,?1,?1 || ?2,?2,?2 ?1 ?1 ?2 ,?2 ,?1 || ?2 ? ,?1 ,?1 ?1,?1,?1 ?1 ? ,?1 ,?1 || ?2,?2,?2 ?1,?1,?1 || ?2,?2,?2 ?1 ? ,?2 ,?2 ?2,?2,?2 ?2 ? ,?2 ,?2 ?1,?1,?1 || ?2,?2,?2 ?1,?1,?1 || ?2
Internal and External Communication print( Name: ); name = scan(); print( Message: ); msg = scan(); message = name + says + msg; network_send(message); response = network_recv();
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; c! Name: c?name c! Message: c?msg n!(name + says + msg) n?resp
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; }
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; }
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; }
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; }
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; } network! Alicesays hi
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; while(true){ console?x; if(x = Name: ) console! Alice ; else if(x = Message: ) console! hi ; } network! Alicesays hi network?resp
Internal and External Communication console! Name: ; console?name; console! Message: ; console?msg; message = name + says + msg; network!message; network?response; network?x; network! Acknowledged. console! Name: console?name console! Message: console?msg
Internal and External Communication Console I/O, network communication, message-passing, etc. can be considered to be either internal or external to a system Internal communication has known values; external communication is visible to and affected by the outside world Composing processes that communicate on the same channel turns it from external into internal We can draw the lines differently for different purposes All of this is made possible by treating I/O as labels!
Semantics of I/O: Summary If we re only interested in the I/O behavior of one single- threaded program, we can represent I/O as internal state To model concurrency or interaction, we can represent I/O as labels on small-steps Labels have no meaning in and of themselves, but can be composed with a way of interpreting the labels To describe the meaning of a program with I/O, we have to consider not just the program but its external environment!