Pointer Initialization Syntax and Operations
Pointer initialization involves syntax such as data type, variable name, and examples. Explore the usage of null pointers, reference operators, and dereference operators in C++. Understand the difference between reference and dereference operations through practical examples to better comprehend how pointers work in programming.
Download Presentation
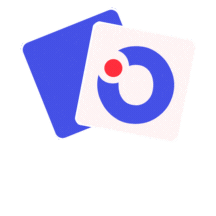
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Lecture of Pointer Lecture of Pointer 2 nd Stage (2020-2021) Assist. Lec. Liqaa S.M.
1. 1. Initialization of Initialization of pointers pointers Syntax Data Type * Pointer variable name Example: int * a; // pointer to an integer double *da; // pointer to a double float *fa; // pointer to afloat char *ch; // character pointer
2 . Pointer void 2 . Pointer void Syntax Void * Pointer variable name Example: void * vp; // pointer to a void
3. Null 3. Null pointer pointer A null pointer is a regular pointer of any pointer type which has a special value that indicates that it is not pointing to any valid reference or memory address. This value is the result of type-casting the integer value zero to any pointer type. int * p; p = 0; // p has a null pointer value
Reference operator (&) Reference operator (&) Syntax & Pointer variable name Example: andy = 25; fred = andy; ted = &andy;
Dereference operator (*) Dereference operator (*) Example: beth = *ted; beth = ted; beth = *ted; // beth equal to ted ( 1776 ) // beth equal to value pointed by ted ( 25 )
difference between the reference and dereference operators & is the reference operator and can be read as "address of . * is the dereference operator and can be read as "value pointed by .
Example: #include <iostream> using namespace std; int main () { int firstvalue = 5, secondvalue = 15; int * p1, * p2; p1 = &firstvalue; p2 = &secondvalue; // p2 = address of second value *p1 = 10; // value pointed by p1 = 10 *p2 = *p1; // value pointed by p2 = value pointed by p1 p1 = p2; // p1 = p2 (value of pointer is copied) *p1 = 20; // value pointed by p1 = 20 cout << "first value is = " << firstvalue << endl; cout << "second value is =" << secondvalue << endl; return 0; } second value is = 20 // p1 = address of first value Result first value is = 10
Pointers to pointers Pointers to pointers char a; // a has type char char * b; // b has type char * char ** c; // C has type char ** a = 'z'; // a has type char and a value of 'z' b = &a; //b has type char* and a value of 7230 c = &b; // c has type char** and a value of 8092
Pointers and Pointers and arrays arrays #include <iostream> using namespace std; int main () { int numbers[5]; int * p; p = numbers; *p = 10; p++; *p = 20; p = &numbers[2]; *p = 30;
p = numbers + 3; *p = 40; p = numbers; *(p+4) = 50; for (int n=0; n<5; n++) cout << numbers[n] << ", "; return 0; } Result = 10, 20, 30, 40, 50
Pointers Arithmetic Operations 1. Increment (++) 2. Decrement ( ) 3. Pointer addition 4. Pointer subtraction
Increment (++) char *mychar; short *myshort; long *mylong; mychar++; myshort++; mylong++;
Pointers to functions Pointers to functions Syntax return_type *Pointer function_name ( parameter list ) { body of the function }
#include <iostream> using namespace std; int addition (int a, int b){ return (a+b); } int subtraction (int a, int b){ return (a-b); } int operation (int x, int y, int (*functocall) (int,int)) { int g; g = (*functocall)(x,y); return (g); } int main () { int m,n; int (*minus)(int,int) = subtraction; m = operation (7, 5, addition); n = operation (20, m, minus); cout <<n; return 0; }