Introduction to React Native Components and APIs
This document provides an overview of React Native components and APIs including hybrid mobile applications, state management, common UI components, iOS and Android wrappers, and other essential features. It also offers useful links for further exploration and reference.
Download Presentation
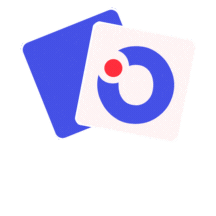
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
1 Hybrid Mobile Applications - ICD0018 TalTech IT College, Andres K ver, 2019-2020, Fall semester Web: http://enos.Itcollege.ee/~akaver/HybridMobile Skype: akaver Email: akaver@itcollege.ee
React N simple state 2 interface State { level: number; } export class Hello extends React.Component<Props, State>{ constructor(props: Props) { super(props); this.state = { level: props.level } } onIcrement = () => this.setState({ level: this.state.level + 1 }); onDecrement = () => this.setState({ level: this.state.level - 1 }); render() {
React N simple state 3 render() { return ( <View style={styles.root}> <Text style={styles.greeting}> Hello, {this.props.name}! Level: {this.state.level}! </Text> <View> <View> <Button title="-" onPress={this.onDecrement} /> </View> <View> <Button title="+" onPress={this.onIcrement} /> </View> </View> </View> ); }
React N Basic components 4 Most common UI On screen list views View Button FlatList Text Switch SectionList Image Picker TextInput ie only on screen content is rendered like RecyclerView in Android Slider ScrollView StyleSheet
React N iOS components 5 Common UIKit wrappers ActionSheetIOS AlertIOS DatePickerIOS ImagePickerIOS ProgressViewIOS PushNotificationIOS SegmentControlIOS TabBarIOS
React N - Android components 6 BackHandler DatePickerAndroid DrawerLayoutAndroid PermissionsAndroid ProgressBarAndroid TimePickerAndroid ToastAndroid ToolbarAndroid ViewPagerAndroid
React N - Other 7 ActivityIndicator Linking Alert Modal Animated PixelRatio Clipboard RefreshControl Dimensions StatusBar KeyboardAvoidingView WebView
React N Components 8 Use the docs https://facebook.github.io/react-native/docs/components-and-apis External components http://www.awesome-react-native.com Or npm registry react-native https://www.npmjs.com/search?q=react-native&page=1&ranking=optimal
React N Style/Dimensions 9 Fixed dimensions Width and height Unitless density independent pixels Or percentage Flex space (along main axis) Similar to layout_weight in Android Default is flex: 1 Space shared proportionally amongst other components with the same parent flex 1 + flex 2 => sum is 3. So first component will use 1/3 and second 2/3 of available space
React N - Flexbox 10 Flexbox is designed to provide a consistent layout on different screen sizes. (similar to web flexDirection default is row and flex supports only single number) Combine with flexDirection, alignItems, and justifyContent to achieve the right layout.
React N - Flexbox 11 flexDirection row align from left to right column align from top to bottom row-reverse column-reverse
React N - Flexbox 12 justifyContent how to align children within the main axis flex-start flex-end center space-between divide empty space evenly between children X___X___X space-around divide empty space evenly around every children _X__X__X_ space-evenly all empty spaces are exactly the same (start edge, end endge and in-betweens) _X_X_X_
React N - Flexbox 13 alignItems cross axis alignment (only, if width is not fixed) stretch default (full possible cross axis size) flex-start flex-end center baseline alignSelf - override parents alignItems seting.
React N - Flexbox 14 alignContent when wrapping to multiple lines flexWrap wrap wraps to multiline when needed nowrap
React N - Flexbox 15 flexBasis, flexGrow, flexShrink flexBasis default size of the item, before grow and shrink flexGrow float, >=0 (0 is default). How any space is divided among the children along the main axis. Similar to layout_weight flexShrink how to shrink the children, if size overflows. Float >=0, (1 is default)
React N - Flexbox 16 position positionTop, positionBottom, positionLeft, positionRight dp pixel to move component padding (top, bottom, left, right) border margin
React N Yoga playground 17 Interactive web based playground for testing layout styling logic https://yogalayout.com/playground/
React N - networking 18 Classical JS. Fetch is built-in. XMLHttpRequest is built-in (so you can use axios or frisbee). WebSocket is supported. getMoviesFromApiAsync = () : Promise<any> => { return fetch('https://facebook.github.io/react-native/movies.json') .then((response) => response.json()) .then((responseJson) => { return responseJson.movies; }) .catch((error) => { console.error(error); }); }
React N Navigation between screens 19 npm install --save react-navigation react-navigation-stack react-native-gesture-handler react-native-reanimated react-native-screens Create navigator, app container, screens and set entry point
React N - Navigation 20 Create navigator and app container import { createAppContainer } from 'react-navigation'; import { createStackNavigator } from 'react-navigation-stack'; import { HomeScreen } from './screens/HomeScreen'; import { ProfileScreen } from './screens/ProfileScreen'; const MainNavigator = createStackNavigator({ Home: { screen: HomeScreen }, Profile: { screen: ProfileScreen } }, { initialRouteName: 'Home'} ); const App = createAppContainer(MainNavigator); export default App;
React N - Navigation 21 Homescreen import React from 'react'; import { Button } from 'react-native'; import { NavigationParams, NavigationScreenProp, NavigationState, } from 'react-navigation'; export interface Props { navigation: NavigationScreenProp<NavigationState, NavigationParams>; } export class HomeScreen extends React.Component<Props> { static navigationOptions = { title: 'Home Screen' }; constructor(props: Props) { super(props); } render() { const { navigate } = this.props.navigation; return ( <Button title="Go to Akaver's profile" onPress={() => navigate('Profile', { text: 'Akaver' })} /> ); } }
React N - Navigation 22 Profile screen import React from 'react'; import { StyleSheet, View, Text, Button } from 'react-native'; import { NavigationScreenProp, NavigationState, NavigationScreenConfig, } from 'react-navigation'; interface NavigationParams { text: string; } interface Props { navigation: NavigationScreenProp<NavigationState, NavigationParams>; } export class ProfileScreen extends React.Component<Props> { constructor(props: Props) { super(props); } static navigationOptions = { title: 'Profile Screen ' }; render() { const navigate = this.props.navigation.navigate; return ( <Button title={this.props.navigation.state.params!.text + ' - go to Home! '} onPress={() => navigate('Home')} /> ); } }
23 THE END!
React N 24