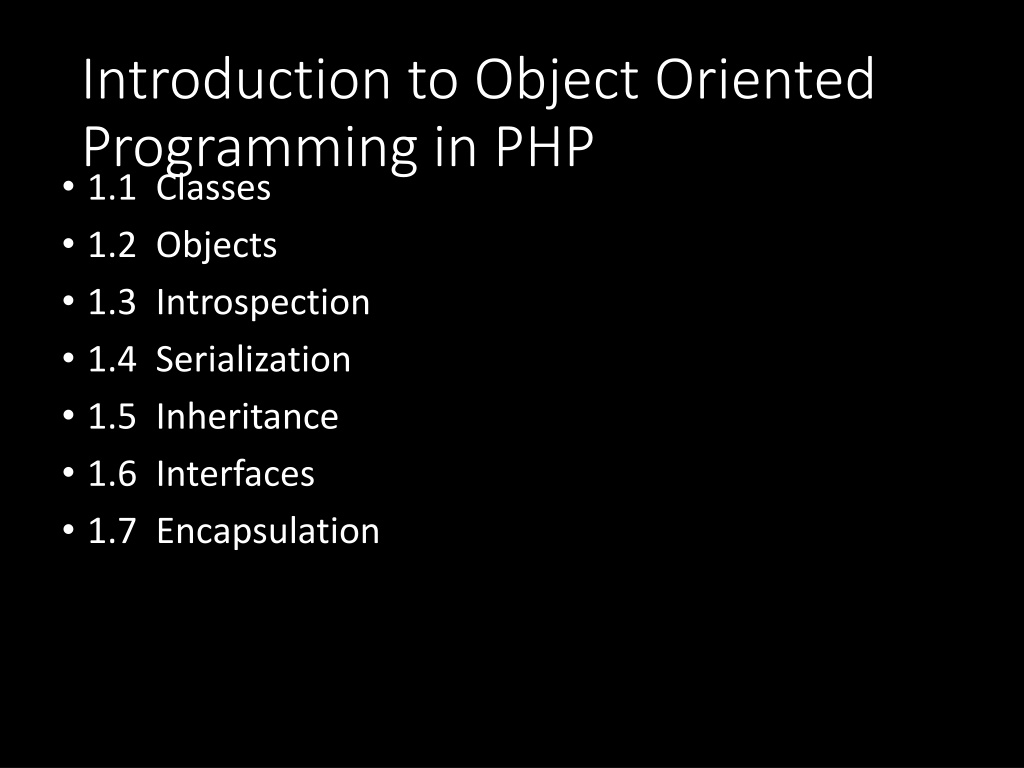
Introduction to Object-Oriented Programming in PHP
Explore the fundamentals of Object-Oriented Programming in PHP, covering classes, objects, introspection, serialization, inheritance, interfaces, encapsulation, and more. Learn how to create classes, add properties and methods, utilize the $this variable, and work with objects to build powerful applications.
Download Presentation
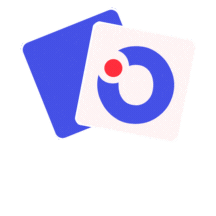
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Introduction to Object Oriented Programming in PHP 1.1 Classes 1.2 Objects 1.3 Introspection 1.4 Serialization 1.5 Inheritance 1.6 Interfaces 1.7 Encapsulation
Class Class is a way to bind the data and its associated functions together. It allows data and functions to be hidden if necessary , from external use. When defining class, we are creating abstract data types that can be used like any other built-in data types.
Creating class in php <?php class Student { } class emp { } ?>
Adding property to class When you create a variable inside a class, it is called a 'property'. It shows attributes of real object. It is same as data member in c++. You can add default values to properties. But default value must be constant.
Adding properties in class <?php class student { var $name= abc ; var $marks=90; } ?>
Adding methods A function defined in a class is called a method. It is same as member functions in class. -> operator is used to access properties or methods.
Adding methods to class <?php class student { var $name; var $marks; function setdata($nm,$mk) { $this->name=$nm; $this->marks=$mk; } } ?>
$this variable The $this is a built-in variable (built into all objects) which points to the current object. Or in other words, $this is a special self-referencing variable. You use $this to access properties and to call other methods of the current class.
Objects Object is an instance of your class. If you have class then you need to create object of the class to solve your problem using class. You can create object of your class by using new keyword. $obj = new myClass();
Creating objects <?php class student { var $name; var $marks; function setdata($nm,$mk) { $this->name=$nm; $this->marks=$mk; } } $obj1=new student; $obj1->setdata( abc , 90); echo "Name = $obj1->name<br>"; echo "Marks = $obj1->marks<br>"; ?>
Encapsulation Encapsulation means hiding or wrapping the code and data into a single unit to protect the data from outside world. It is used to protect class s internal data( properties and method) from code outside that class and hiding details of implementation. In PHP , encapsulation is provided by visibility specifiers.
Visibility Specifier in PHP There are 3 types of visibility available in php for controlling your property or method. Public: Public method or property can be accessible from anywhere. i.e. from inside the class, out side the class and in child class also. Private: Method or property with private visibility can only be accessible inside the class. You can not access private method or property from outside of your class. Protected: Method or variable with protected visibility can only be access in the derived class. Or in other word in child class. Protected will be used in the process of inheritance.
Public Visibility in PHP Classes Public visibility is least restricted visibility available in php. If you will not define the visibility factor with your method or property then public will be by default applied. Public methods or variables can be accessible from anywhere. For example, It can be accessible from using object(outside the class), or inside the class, or in child class
Example class test { public $abc; public $xyz; public function f1() { echo inside function ; } } $objA = new test(); echo $objA->abc;//accessible from outside $objA->f1();//public method of the class test
Private Visibility in PHP Classes Private method or properties can only be accessible within the class. You can not access private variable or function of the class by making object out side the class. But you can use private function and property within the class using $this object. Private visibility in php classes is used when you do not want your property or function to be exposed outside the class.
Class test { public $abc; private $xyz; public function pubDo($a) { echo $a; } private function privDo($b) { echo $b; } public function pubPrivDo() { $this->xyz = 1; $this->privDo(1); } } $objT = new test(); $objT->abc = 3;//Works fine $objT->xyz = 1;//Throw fatal error of visibility $objT->pubDo("test");//Print "test" $objT->privDo(1);//Fatal error of visibility $objT->pubPrivDo();//Within this method private function privDo and variable xyz is called using $this variable.
Protected Visibility in PHP Classes Protected visibility in php classes are only useful in case of inheritance and interface. Protected method or variable can be accessible either within class or child class
<?php class parent1 { protected function testParent() {echo "this is test"; }} class child extends parent1 {public function testChild() {$this->testParent(); //will work because it }} $objParent = new parent1(); $objParent->testParent();//Throw error $objChild = new child(); $objChild->testChild();//work because test child will ?> .
Constructors Constructor Functions are special type of functions which are called automatically whenever an object is created. It is a special function that initializes the properties of the class. There are two ways to create constructors in php.
Creating constructors PHP provides a special function called __construct() (two underscores) to define a constructor. You can pass as many as arguments you like into the constructor function. A constructor is a function with the same name as the class in which it is defined.
Method1 in PHP 5 onwards <?php class student { public $name; public $marks; function __construct($nm,$mk) { $this->name=$nm; $this->marks=$mk; } } $obj1=new student( abc ,90); echo "Name = $obj1->name<br>"; echo "Marks = $obj1->marks<br>"; ?>
Method2 in PHP4 <?php class student { var $name; var $marks; function student($nm,$mk) { $this->name=$nm; $this->marks=$mk; } } $obj1=new student( abc ,90); echo "Name = $obj1->name<br>"; echo "Marks = $obj1->marks<br>"; ?>
destructors Destructor: Like a constructor function you can define a destructor function using function __destruct(). You can release all the resources with-in a destructor.
<?php class student { public $name; public $marks; public function __construct($nm,$mk) { $this->name=$nm; $this->marks=$mk; } public function __destruct() { echo "calling destructor"; } } $obj1=new student("abc", 90); echo "Name = $obj1->name<br>"; echo "Marks = $obj1->marks<br>"; ?>
Inheritance in PHP Inheritance is nothing but a design principle in oop. With the help of inheritance you can increase re- usability of code By implementing inheritance you can inherit(or get) all properties and methods of one class to another class. The class who inherit feature of another class known as child class. The class which is being inherited is know as parent class. For example, child inherits characteristics of their parent. Same is here in oop. One class is inheriting characteristics of another class.
Types of inheritance in php Single level Inheritance Multi-level Inheritance Hierarchical Inheritance Multiple Inheritance is not supported in php. But you can implement it through interface.
Single level inheritance In this you can derive child class from base class using extends keyword. class base_name { } class derived_name extends base_name { }
Multi-Level Inheritance class A { } class B extends A { } class C extends B { }
Hierarchical Level Inheritance class A { } class B extends A { } class C extends A { }