Introduction to Lex and YACC
Lex and YACC are essential tools in computer science theory for building lexical analyzers and parsers. Lex utilizes regular expressions to build a lexer, while YACC uses CFGs to construct a bottom-up parser. Learn about compiling with YACC, printing in C, Lex file format, YACC file format, and how to run and test programs using Lex and YACC.
Download Presentation
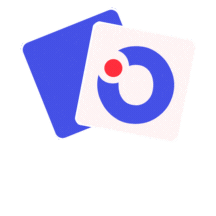
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Intro to Lex and YACC Intro to Lex and YACC CSCI 432 Computer Science Theory CSCI 432 Computer Science Theory
Overview Overview Lex uses regular expressions to build a lexical analyzer. The function is named yylex() YACC uses CFGs to build a bottom-up parser. YACC = Yet Another Compiler Compiler The function yyparse() builds a parse tree. yyparse() calls yylex() over and over. When yyparse() finds a syntax error, it calls yyerror() then dies.
Compiling Compiling yacc -d project.yacc creates two files: y.tab.c and y.tab.h lex project.lex creates the file lex.yy.c cc y.tab.c lex.yy.c -ll -ly combines lex and yacc to create executable a.out
printing in C printing in C #include <stdio.h> int cnt = 0; char var[10] = "hello"; printf("hi mom\n"); printf("int variable cnt contains %d\n", cnt); printf("string var contains %s\n", var);
Lex File Format Lex File Format %{ //any C code you need for yylex() #include <stdio.h> #include <string.h> #include "y.tab.h" %} declarations %% regular_expressions actions %% driver code, eg main()
YACC File Format YACC File Format %{ #include <stdio.h> #include <string.h> probably some global variables %} %token list of tokens (all your terminals) %% grammar rules actions %% main() yyerror()
%{ #include <stdio.h> %} %% "-" printf ("MINUS\n"); "+" printf ("PLUS\n"); [0-9]+ printf ("NUM - %s\n", yytext); Lex file to find numbers, + and - \n ; . ; %% This yylex() is not called by yyparse(). int main() { while (yylex()); }
Compile and Run $ lex calc0.lex $ cc lex.yy.c -ll $ a.out 22 + 77 - 3 NUM - 22 PLUS NUM - 77 MINUS NUM - 3 99 + bob - joe * 13 NUM - 99 PLUS MINUS NUM - 13
%{ #include <stdio.h> #include <string.h> YACC file to create a very simple calculator extern int yylex(); void yyerror(char *); int num=0; int sum=0; part 1 of 3 %} %token NUM PLUS MINUS RETURN %%
YACC file to create a very simple calculator part 2 of 3 %% prog: stmts stmts: stmt | stmt stmts stmt: NUM { sum = num; } | PLUS NUM { sum = sum + num; } | MINUS NUM { sum = sum - num; } | RETURN { printf("--> %d\n",sum); } %%
%% int main() { yyparse(); } YACC file to create a very simple calculator void yyerror (char *msg) { printf("\n%s\n", msg); } part 3 of 3
%{ #include <stdio.h> #include <string.h> #include "y.tab.h" Lex file to create a very simple calculator extern int num; %} %% "-" return MINUS; "+" return PLUS; [0-9]+ { num=atoi(yytext); return NUM; } \n return RETURN; . ; %%
Sample Run of the Calculator $ a.out 99 --> 99 99 + 1 --> 100 + 50 --> 150 --> 150 10 + 10 + 10 + 10 - 5 --> 35 -35 --> 0 hi mom --> 0
Makefile Makefile lex.yy.c y.tab.h: calc.lex lex calc.lex y.tab.c: calc.yacc yacc -d calc.yacc calc: y.tab.c lex.yy.c cc y.tab.c lex.yy.c -ll -ly -o calc Tab, not spaces