File Management in C Programming
Managing files in C programming involves utilizing system calls such as fopen, fclose, and file descriptors. These low-level functions interact with file streams and the file descriptor table, allowing for file creation, opening, reading, and writing operations. Learn about I/O system calls, file control libraries, and the various functions involved in handling files efficiently.
Download Presentation
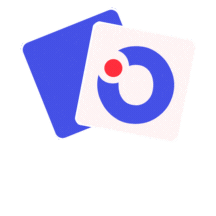
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Opening Files Opening Files Huma Javed
fopen fopen() and () and fclose fclose() () The fopen() and fclose() system call returns a file stream, FILE *. These are C library functions which really use system calls. OS is responsible for managing device I/O resources, such as reading and writing from keyboards, disks, etc. OS is also responsible for managing the file system, such as keeping track of files, directories, and paths. Both I/O resources and file system come into play while opening a file, or reading and writing from that file.
File Descriptor Table File Descriptor Table The system call to open a file is open() The system call to close a file is close() These system calls are low level and do operate over file streams, as FILE *, Return an integer value which is the file descriptor. All open files in the operating system are managed via file descriptors which are indexes into the file descriptor table. The file descriptor table is a kernel data structure which tracks open files for all programs The reference to open files is via an integer value, the file descriptor. The standard file descriptors. Each has an assigned number: 0, stdin; 1, stdout; and, 2 stderr. To open a new file, it will be assigned the next lowest file descriptor number available, which might be 3 for the first file, and then 4, and so on.
I/O System calls I/O System calls Basically there are total 5 types of I/O system calls: Create: Used to Create a new empty file. Syntax in C language: int creat(char *filename, mode_t mode) Parameter : filename : name of the file which you want to create mode : indicates permissions of new file. Returns : return first unused file descriptor (generally 3 when first creat use in process beacuse 0, 1, 2 fd are reserved) return -1 when error How it work in OS: Create new empty file on disk Create file table entry Set first unused file descriptor to point to file table entry Return file descriptor used, -1 upon failure
open() File Descriptor To open a file open() system call is defined in the file control library, fcntl.h The function prototype is as follows: int open(const char *path, int oflag, ... /*mode_t mode*/ ); There are either two or three arguments to open(). If not creating a file, open() only takes two arguments, int fd = open("path/to/file", O_RDONLY); The oflag argument, in the above example, is O_RDONLY flag which means the file at given path is opened for reading, only. If a file is already created, specify the permission mode of that file, such as, read/write/exec.
Open File open: Used to Open the file for reading, writing or both. Syntax in C language #include<sys/types.h> #includ<sys/stat.h> #include <fcntl.h> int open (const char* Path, int flags [, int mode ]); Parameters Path : path to file which to use use absolute path begin with / , when not work in same directory of file. Use relative path which is only file name with extension, when work in same directory of file. flags : O_RDONLY: read only, O_WRONLY: write only, O_RDWR: read and write, O_CREAT: create file if it doesn t exist, O_EXCL: prevent creation if it already exists How it works in OS Find existing file on disk Create file table entry Set first unused file descriptor to point to file table entry Return file descriptor used, -1 upon failure
// C program to illustrate // open system call Output: fd = 3 #include<stdio.h> #include<fcntl.h> #include<errno.h> extern int errno; int main() { // if file does not have in directory // then file foo.txt is created. int fd = open("foo.txt", O_RDONLY | O_CREAT); printf("fd = %d/n", fd); if (fd ==-1) { // print which type of error have in a code printf("Error Number % d\n", errno); // print program detail "Success or failure" perror("Program"); } return 0; }
close close() () File File Finally, to close the file descriptor use the close() system call Defined in unistd.h. It has the following function prototype int close(int filde) All open file descriptors should be closed. Once a program exists, the file descriptors are closed automatically.
Format in C Tells the operating system you are done with a file descriptor and Close the file which pointed by fd. Syntax in C language #include <fcntl.h> int close(int fd); Parameter fd :file descriptor Return 0 on success. -1 on error. How it works in the OS Destroy file table entry referenced by element fd of file descriptor table As long as no other process is pointing to it! Set element fd of file descriptor table to NULL
include<stdio.h> #include <fcntl.h> int main() { int fd1 = open("foo.txt", O_RDONLY); if (fd1 < 0) { perror("c1"); exit(1); } printf("opened the fd = % d\n", fd1); // Using close system Call if (close(fd1) < 0) { perror("c1"); exit(1); } printf("closed the fd.\n"); } Output: opened the fd = 3 closed the fd.