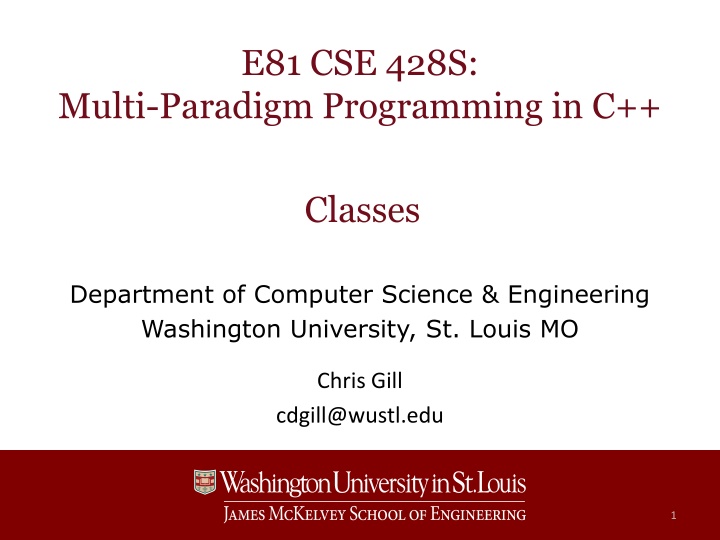
C++ Classes and Structs: Encapsulation and Declaration
Learn about the concepts of encapsulation and declaration in C++ classes and structs, including how functions encapsulate behavior and data usage, when to use structs vs. classes, and the structure of a simple C++ class declaration.
Download Presentation
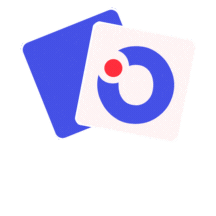
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
E81 CSE 428S: Multi-Paradigm Programming in C++ Classes Department of Computer Science & Engineering Washington University, St. Louis MO Chris Gill cdgill@wustl.edu 1
From C++ Functions to C++ Structs/Classes C++ functions encapsulate behavior/transformation Data used/modified by a function is passed in via parameters Can export data a function produces via return statement Classes (and structs) encapsulate state and behavior Member variables maintain each object s state Member functions and operators have direct access to member variables of the object on which they are called Class members are private by default, struct members public by default When to use a struct Use a struct for things that are mostly about the data Add constructors and operators to them to work with library containers/algorithms When to use a class Use a class to encapsulate state behind an interface Prefer classes to support substitution and polymorphism CSE 428S Multi-Paradigm Programming in C++ 2
Declaring and Defining C++ Structs/Classes // struct declaration (in point2d.h) struct Point2D { Point2D (int x, int y); bool operator< (const Point2D &) const; int x_; int y_; }; // method definitions (in point2d.cpp) Point2D::Point2D (int x, int y) : x_(x), y_(y) {} bool Point2D::operator< (const Point2D & p2d) const { return (x_ < p2d.x_) || ((x_ == p2d.x_) && (y_ < p2d.y_)); } promises not to modify object on which it s called base class/struct and member initialization list CSE 428S Multi-Paradigm Programming in C++ 3
Structure of a Simple C++ Class Declaration class Date { public: // member functions, visible outside the class compiler defines these* if you don t Date (); // default constructor Date (const Date &); // copy constructor can be members as well operators Date (int d, int m, int y); // another constructor virtual ~Date (); // (virtual) destructor Date & operator= (const Date &); // assignment operator int d () const; int m () const; int y () const; // accessors void d (int); void m (int); void y (int); // mutators string yyyymmdd () const; // generate a formatted string private: // member variables visible only within functions above int d_; int m_; int y_; }; end of the class declaration Don t forget semicolon at the *Compiler omits default constructor if any constructor is declared CSE 428S Multi-Paradigm Programming in C++ 4
Constructors class Date { public: Date (); Date (const Date &); Date (int d, int m, int y); // ... private: int d_, m_, y_; }; // default constructor Date::Date () : d_(0), m_(0), y_(0) {} // copy constructor Date::Date (const Date &d) : d_(d.d_), m_(d.m_), y_(d.y_) {} // another constructor Date::Date (int d, int m, int y) : d_(d), m_(m), y_(y) {} A constructor has the same name as its class Passed parameters can be used in the base class / member initialization list You must not do initializations there that may fail You should do all other member and base class initializations there You must initialize const and reference members there Members are constructed in the order they were declared List should follow that order CSE 428S Multi-Paradigm Programming in C++ 5
Access Specifications Declaring access within a class or struct private: visible only within the class protected: also visible within derived classes public: visible everywhere Access control in a class is private by default but, it s better style to specify access explicitly A struct is the same as a class, except that access to members of a struct is public by default CSE 428S Multi-Paradigm Programming in C++ 6
Studio 6 Practice declaring structs and classes Examine how compiler-provided constructors work Declare and use access specifiers Declare and define accessors and mutators Studios 0 through 11 are due 11:59pm Monday October 16th (the night before Exam 0) Submit as soon as each is done so you get feedback and can resubmit any that may be marked incomplete CSE 428S Multi-Paradigm Programming in C++ 7