Computational Thinking Example for Image Storage Calculation
Explore a computational thinking example involving calculating how many images of different formats can be stored on a flash drive based on user input drive size in gigabytes. Understand image compression ratios and pixel resolutions to develop a Python program that outputs the number of images that can be stored for GIF, JPEG, PNG, and TIFF formats.
Download Presentation
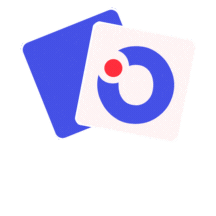
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
ESSENTIAL COMPUTATIONAL THINKING: CHAPTER 03 - EXAMPLE Dr. Ricky J. Sethi Essential Computational Thinking
Chapter 3 Computational Thinking and Structured Programming EXAMPLE PROBLEM
Computational Thinking Example Let s imagine we need to store a bunch of images on a flash drive We might have different kinds of images and different sizes of drives How might we do so?
Initial Problem Specification Problem Description: Calculate how many pictures can fit on a drive Problem Requirement: Develop a computational solution in Python that determines how many images can be stored on a given size drive Problem Specification: The size of the drive is to be entered by the user in gigabytes (GB). The number of images that can be stored must be calculated for GIF, JPEG, PNG, and TIFF image file formats. The program output should be formatted as given below. Enter drive size (GB): 4 xxxxx images in GIF format can be stored xxxxx images in JPEG format can be stored xxxxx images in PNG format can be stored xxxxx images in TIFF format can be stored
Details and Assumptions The ultimate file size of a given image depends not only on the image format used, but also on the image itself. In addition, formats such as JPEG allow the user to select the degree of compression for the image quality desired. For this program, we assume the image compression ratios given below. Also assume that all the images have a resolution of 800 x 600 pixels. Thus, for example, an 800 x 600 resolution image with 16-bit (2 bytes) color depth would have a total number of bytes of 800 x 600 x 2 = 960,000. For a compression rate of 25:1, the total number of bytes needed to store the image would be 960000/25 = 38400. Finally, assume that a GB (gigabyte) equals 1,000,000,000 bytes.
Solving the problem How do we start? Conceptual vs Pragmatic Understanding Let s start by exploring how images are represented digitally and biologically Then, we can start applying computational thinking to solve the problem
Image Characteristics Biological Vision: Rods and Cones: distributed on retina and RGB cones are concentrated in fovea Photoreceptors are actually neurons that fire all the time Transform 11-cis-retinal when a photon of light impacts the molecule Vision seems continuous but is actually discretized Brain interprets, rather than represents, physical stimuli V1 Occipital Cortex with Dorsal and Ventral processing Images are an interpretation not exact representation Digital images are also made of pixels
First: Simplify Let s start by simplifying the problem so we can solve it Let s start by ignoring details we consider unessential for this first approximation We thus introduce constraints We can relax these constraints later when we construct the more general solution Use Divide-And-Conquer Simplify the problem by dividing it into small chunks E.g., compute just for GIFs Romans decimate disloyal legionnaires and barbarians ABSTRACTION: one of 5 essential Computational Thinking Ideas
Second: Find an Appropriate Data Representation First get the (data) representation Simplify the problem by simplifying the representation as needed E.g., assume USB = 300 bytes and 1 image = 30 bytes Algorithms + Data Structures = Programs by Niklaus Wirth
Third: Find the Algorithm NOW we can find the algorithm Express the math/solution in simple English as if explaining to a friend THEN convert to Python E.g., might say compute size of a GIF image then use that as a conversion ratio (300 bytes x 1 image/30 bytes) ALGORITHM: Mathematical Calculation: Computable Function! 1 ????? ????_???_??? ???_????? = ????_??? Example of GENERALIZATION in that you already know how to do the calculation of 300/30 but now we systematize and formalize it so we can co-opt it and apply it to other problems, as well.
Summary: Computational Thinking Tips Use Divide-And-Conquer Simplify the problem by dividing it into small chunks E.g., compute just for GIFs First get the (data) representation Simplify the problem by simplifying the representation as needed E.g., assume USB = 300 bytes and 1 image = 30 bytes THEN find the algorithm Express the math/solution in simple English as if explaining to a friend THEN convert to Python E.g., might say compute the size of a GIF image then use that as a conversion ratio (300 bytes x 1 image/30 bytes) Algorithms + Data Structures = Computational Solutions
Final Algorithm Step 1: Prompt User for size_usb_drive Step 2: Calculate size_one_gif_file Step 3: Calculate num_files that fit on the drive This is like y = f(x) where: X is INPUT (Step 1) Y is the OUTPUT (Step 3) F is the formula in Step 3 This is the ALGORITHM: it s a Mathematical Calculation: it s a Computable Function! 1 ????? ????_???_??? ???_????? = ????_??? y=f(x) num_files=f(size_usb)
Implementation (1st Approximation) # What Program Does: Given a drive of a certain size, it computes how many GIF files will fit on there # 1) Prompt User for size_usb_drive size_usb_drive = int( input('What is the size of your USB drive (in GB please)? ') ) # 2) Calculate size_gif_file size_one_gif_file = 30 # 3) Calculate num_files that fit on the drive and output to the user using truncated division num_files = size_usb_drive // size_gif_file print(num_files)
Summary: Do Algorithm Development Determine Specifications Describe exactly what your program will do. Don t worry about how the program will work, but what it will do. Includes describing the inputs, outputs, and how they relate to one another. Create a Design Formulate the overall structure of the program. This is where the how of the program gets worked out. You choose or develop your own algorithm that meets the specifications. Implement the Design Translate the design into a computer language.
Outline of Program Specifications: What does the program do? Inputs Outputs How are outputs related to inputs? Design: Overall structure of how to solve the problem Implementation: Translate design to Python
Some Next Steps: Additonal Specifications Can start to relax constraints like adding color depth, compression ratios, different image types, etc. Inputs: Size of the drive: size_usb_drive = 100 bytes Size of each file? Resolution: resolution = 10 pixels Color Depth: color_depth = 2 bytes Compression Ratio: compression = 2 Outputs: Number of files that will fit on the drive: num_files ALGORITHM: Mathematical Calculation: Computable Function! Num_files = size_usb * 1 image/size_one_gif Translate to Python: num_of_files = size_usb_drive/size_one_compressed_gif
More General Implementation (relaxed constraints) # What Program Does: Given a drive of a certain size, it computes how many GIF files will fit on there # 1) Prompt User for size_usb_drive size_usb_drive = int( input('What is the size of your USB drive (in GB please)? ') ) # 2) Calculate size_gif_file # Set our input variables first! resolution = 10 # units of pixels (px) color_depth = 2 compression = 2 # First calculate the size of one UNCOMPRESSED GIF file size_one_gif_file = resolution * color_depth # Caclulate the size of a compressed gif file: size_gif_file = size_one_gif_file / compression # 3) Calculate num_files that fit on the drive and output to the user using truncated division num_files = size_usb_drive // size_gif_file print(num_files)