Understanding Scala's Implicit Conversions and Parameters
Scala's implicit keyword allows for type conversions by the compiler, inferring parameters when not directly stated, and is useful for integrating external code. Implicit conversion rules include marking, scope, one-at-a-time, and explicits-first rules. This guide provides examples and insights into leveraging implicit conversions effectively in Scala programming.
Download Presentation
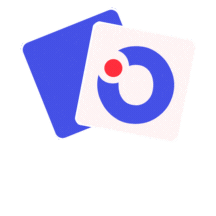
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Implicit Conversions and Parameters in Scala Abraham Peediakal and Henry Dyer
The implicit keyword What does it do? Provides type conversions to the compiler Infer parameters when they aren t given directly Useful for integrating outside code
val button = new JButton button.addActionListener( new ActionListener { def actionPerformed(event: ActionEvent): Unit = { An Example: JButton println( pressed! ) } } ) // I m supposed to read this?
val button = new JButton button.addActionListener( An Example: JButton (_: ActionEvent) => println( pressed! ) ) // function != ActionListener
implicit def function2ActionListener(f: ActionEvent => Unit): ActionListener = new ActionListener { def actionPerformed(event: ActionEvent): Unit = f(event) }
val button = new JButton button.addActionListener( An Example: JButton (_: ActionEvent) => println( pressed! ) ) // now it works!
Implicit Conversion Rules 1. Marking Rule Only definitions marked implicit are considered
Implicit Conversion Rules 2. Scope Rule An implicit definition must be in scope to be used Import Extends Note: Only needs brought into scope once
Implicit Conversion Rules 3. One-at-a-Time Rule Compiler won t apply multiple conversions at once
Implicit Conversion Rules 4. Explicits-first Rule If the code works, the compiler won t fix it
An Example: Ints and Doubles val i: Int = 3.5 // that s illegal!
implicit def doubleToInt(x: Double) = x.toInt An Example: Ints and Doubles // now it works! val i: Int = 3.5 // i: Int = 3
val oneHalf = new Rational(1, 2) // oneHalf: Rational = 1/2 oneHalf + oneHalf An Example: Rationals // 1/1 oneHalf + 1 // 3/2 1 + oneHalf // that s illegal!
implicit def intToRational(x: Int) = new Rational(x, 1) An Example: Rationals 1 + oneHalf // intToRational(1) + oneHalf // 1/1 + 1/2 // = 3/2
Adding syntax to preexisting classes is easy! Map(1 -> one , 2 -> two , 3 -> three ) ^ Hey how do the arrows work?
Adding syntax to preexisting classes is easy! package scala object Predef { class ArrowAssoc[A](x: A) { def -> [B](y: B): Tuple2[A, B] = Tuple2(x, y) } implicit def any2ArrowAssoc[A](x: A): ArrowAssoc[A] = new ArrowAssoc(x) }
And now Implicit Classes
case class Rectangle(width: Int, height: Int) An Example: Rectangles // shapes are great // constructors are lame // can we make this easier?
implicit class RectangleMaker(width: Int) { An Example: Rectangles def x(height: Int) = Rectangle(width, height) } // automatically generates implicit conversion from int to RectangleMaker