Transitioning EPICS Base to Modern C++ for Enhanced Performance
Enhance your EPICS Base system by utilizing modern C++ features such as strict type checking, zero-cost abstraction, templates, and more. Learn the motivations behind switching to C++ and explore the new C++ features for improved efficiency and maintainability.
Download Presentation
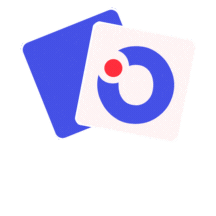
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Moving EPICS Base to Modern C++ Martin Konrad This material is based upon work supported by the U.S. Department of Energy Office of Science under Cooperative Agreement DE-SC0000661, the State of Michigan and Michigan State University. Michigan State University designs and establishes FRIB as a DOE Office of Science National User Facility in support of the mission of the Office of Nuclear Physics.
Motivation: Why C++ for EPICS? Strict type checking Recompiling existing C code with C++ compiler often reveals issues Zero cost abstraction Classes/objects (e. g. std::string vs. C string) Iterators Templates Algorithms like std::copy, std::count_if vs. handcrafted raw loops Compile-time expressions Allows leak free applications without garbage collection (RAII) Some stuff we are maintaining in libCom is now part of standard C++ Bonus With modern compilers we also get Link-time optimization M. Konrad, October 2019 EPICS Workshop, Slide 2
Motivation: Why Switch Now? After being mostly static for a long time C++ has is evolving rapidly since ~10 years Twenty years ago there were many different compilers and standard libraries today there are only three relevant ones: clang, gcc, MSVC Reasonable test matrix Toolchain vendors are working hard to keep up Operating System RTEMS Version 4.9 4.11 5 6 7 Compiler GCC 4.4 GCC 4.9 GCC 7 GCC 4.3 clang C++11 Partial C++14 C++17 Partial Mostly VxWorks Partial M. Konrad, October 2019 EPICS Workshop, Slide 3
Motivation: New C++ Features C++11 C++14 C++17 C++20 Rvalue references, move semantics Better constexpr std::string_view Coroutines Multithreading memory model, std::thread, std::mutex, std::atomic Better lambdas std::optional Modules Binary literals std::any std::span std::unique_ptr, std::shared_ptr Explicitly defaulted/deleted special member functions [[deprecated]] Better unions (std::variant) Filesystem library nullptr Parallel algorithms auto Extended variable initialization [[nodiscard]] Range-based for loop Strongly typed enums Lambda functions Explicit conversion static_assert std::regex Type traits M. Konrad, October 2019 EPICS Workshop, Slide 4
Example: Range-based for Loop vector<int> v; Why do this typedef std::vector<int>::iterator vecIt; for (vecIt it = v.begin(); it != v.end(); ++it) { use(*it); } When you can do this for (auto const& e: v) { use(e); } C++11 M. Konrad, October 2019 EPICS Workshop, Slide 5
Example: Zero-Cost Abstraction uint8_t readByte(); void readBytes(uint8_t* buffer, size_t size) { for (size_t i = 0; i < size; ++i) buffer[i] = readByte(); } M. Konrad, October 2019 EPICS Workshop, Slide 6
Example: Zero-Cost Abstraction uint8_t readByte(); void readBytes(std::span<uint8_t> buffer) C++14 + GSL or C++20 { for (auto& value : buffer) C++11 value = readByte(); } // Can be called with std::array<uint8_t,8> buffer; readBytes(buffer); M. Konrad, October 2019 EPICS Workshop, Slide 7
Example: Zero-Cost Abstraction uint8_t readByte(); void readBytes(std::span<uint8_t> buffer) C++14 + GSL or C++20 { for (auto& value : buffer) C++11 value = readByte(); } // Can be called with std::vector<uint8_t> buffer(8); readBytes(buffer); M. Konrad, October 2019 EPICS Workshop, Slide 8
Example: Zero-Cost Abstraction uint8_t readByte(); void readBytes(std::span<uint8_t> buffer) C++14 + GSL or C++20 { for (auto& value : buffer) C++11 value = readByte(); } // Can be called with uint8_t buffer[8]; readBytes(buffer); M. Konrad, October 2019 EPICS Workshop, Slide 9
Coming up: Networking TS A.k.a. Boost Asio Might become part of the standard soon Programming model for asynchronous communication M. Konrad, October 2019 EPICS Workshop, Slide 10
Potential Path Forward Move to C++ incrementally Compile existing C code with C++ compiler Upgrade toolchains Move more code to C++ Replace existing libCom functions by standard library functions (e.g. replace epicsAtomic by std::atomic) Mark unneeded libCom functions deprecated Make some new features only available with C++11 capable compilers? M. Konrad, October 2019 EPICS Workshop, Slide 11
Discussion M. Konrad, October 2019 EPICS Workshop, Slide 12
Backup Slides M. Konrad, October 2019 EPICS Workshop, Slide 13
Guidelines Support Library I C++ Core Guidelines Helps developers to use modern C++ effectively Lots of real-world advice http://isocpp.github.io/CppCoreGuidelines/CppCoreGuidelines.html Guidelines Support Library Contains a few functions and types that are suggested by the C++ Core Guidelines Important features that the C++ community wants now rather than waiting a few years for the features to make it into the compilers/standard libraries https://github.com/Microsoft/GSL M. Konrad, October 2019 EPICS Workshop, Slide 14
Guidelines Support Library II Example: Function that returns an owning pointer owner<X*> compute(args) { owner<X*> res = new X{}; // ... return res; } This tells analysis tools like clang-tidy that res is an owner. That is, its value must be deleted or transferred to another owner. Owner<X*> decays to X* (no runtime cost) M. Konrad, October 2019 EPICS Workshop, Slide 15
Boost::config Helps Boost library developers adapt to compiler idiosyncrasies; not intended for library users. Defines macros that describe C++ features not supported Example: BOOST_NO_CXX11_CONSTEXPR Would it make sense to have similar facilities in Base? M. Konrad, October 2019 EPICS Workshop, Slide 16