Understanding Concurrency in Go Programming
Concurrency in Go involves the composition of independently executing functions, not parallelism. Key concepts include goroutines, channels for communication, achieving concurrency through communicating sequential processes (CSP), and Go's philosophy of memory sharing through communication. This article explores the fundamentals of concurrency in Go, including examples, potential issues, and practical demonstrations.
Download Presentation
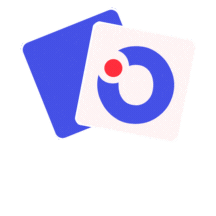
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Concurrency in GO CS240 23/8/2017
What is concurrency? Concurrency is Programming the composition of independently executing functions It s not parallelism: simultaneous execution of different functions Nice talk by Rob Pike: https://vimeo.com/49718712 Examples: I/O Processes Servers communications What could go wrong? Order violations - races Deadlocks
Achieving concurrency Concurrency can be achieved using two ways: Communicating sequential processes (CSP). Using communication for synchronization. Shared memory using locks We are going to focus on CSP today
GOs philosophy: Do not communicate by sharing memory; instead, share memory by communicating.
goroutines Think of them like threads. They have smaller stacks Allows for easy scalability Managed by the process itself The goroutines terminate when main exits
Channels Channels are used to send and receive values from goroutines Send to a channel: ch <- x Receive from a channel: x = <- ch There are two types of channels, buffered and unbuffered channels.
Channels Unbuffered channels: Unbuffered channels blocks sending until some routine receives Receivers are blocked until a goroutine sends This would result in channels having a synchronizes behavior Buffered channels: Allows the channel to accept more elements before releasing it to the receiver
Demo: Redundancy using channels
Concurrency with shared memory? GO still supports the usual shared memory primitives Key functions include: sync.Mutex: mutual exclusion (lock/unlock) sync.Once: do an action exactly once sync.RWMutex: more control on read/write locking More in documentation and tour
To do before next class Finish the Go tour (concurrency) do the Web Crawler exercise Read the MapReduce paper Suggested: Go Concurrency Patterns: https://youtu.be/f6kdp27TYZs Advanced Go Concurrency Patterns: https://youtu.be/QDDwwePbDtw