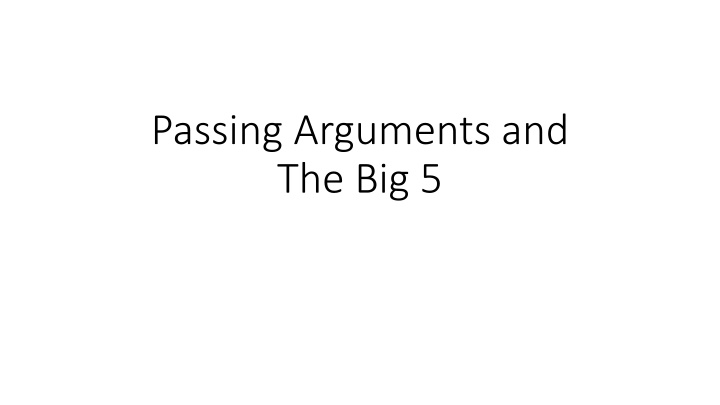
Understanding Passing Arguments in Programming
Learn about passing arguments in programming languages, including by value, by pointer, and by reference. Explore the advantages and disadvantages of each method to improve your coding skills.
Download Presentation
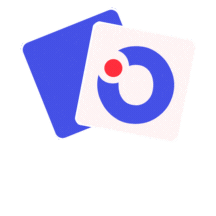
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Passing Arguments and The Big 5
Passing Arguments by Value Pass to the function a copy of a variable Advantages Safe the variable will not be changed in the calling context Disadvantages Slow copying can be slow to copy a large objects as a variable Arrays Not passed by value
Passing Arguments by Pointer Pass to the function a copy of the address of a variable Advantages Flexible can change the value of the object Fast only need to copy a pointer not the whole object Disadvantages Unsafe can change the value of the variable in the calling function Complex the variable is a pointer rather then and needs * or -> to access Arrays Always passed as a pointer since the name of an array is a pointer
Reference Variables Not pointers Can not be null or uninitialized C++ Reference Variables Declare like pointers but use & rather then * They "refer" to another variable, but act like a variable Must be initialized when created so can never be null int my_int = 7; int &my_ref = my_int; my_ref = 8; cout << my_int << endl;
Passing Arguments by Reference Pass to the function a reference to a variable Advantages Flexible can change the value of the object Fast only need to copy an address not the whole object Disadvantages Unsafe can change the value of the variable in the calling function Confusing can not tell the difference at the call site Const Ref Safe like value but fast like a pointer
How hard was week 8 code review assignment? A. Easy B. Moderate C. Challenging D. Unreasonable
How long did week 8 assignment take? A. Less than 3 hours B. 3 to 6 hours C. 6 to 9 hours D. 9 to 12 hours E. More than 12 hours
Return Values Advantages Safe Will always work correctly Disadvantages Slow If a large object must be copied it could be slow With C++11 usually avoided more so with C++14
Return Pointers Advantages Allocations Allows returning of heap allocated objects Disadvantages Unsafe returning a stack address no longer be valid after the return
Return Reference Advantages Fast always fast since only copying an address Disadvantages Unsafe returning a reference to a stack variable will no longer be valid after the return Use Returning values from outside the function
Classes No Internal "new" Member variables Methods Constructor Rule of Zero
Case Study: StringHolder class StringHolder { std::string *string_; public: StringHolder() : string_(nullptr) {}; StringHolder(const char *initial_string); const char* c_str() const noexcept; void ChangeString(const char *input_string); };
Classes Containers Use "new" Rule of Zero won't work Deep vs Shallow Copy
Rule of Three Copy Assignment Operator StringHolder& operator=(const StringHolder &source)
Rule of Three - Copy Constructor StringHolder(const StringHolder &source)
Rule of Three - Destructor ~StringHolder()
Rule of Three vs Rule of Five Rule of five (move semantics new with C++11) Move Constructor Move Assignment Operator rvalue reference && Can bind to a temporary (rvalue)
Rule of Three - Move Constructor StringHolder(StringHolder&& source)
Rule of Three Move Assignment Operator StringHolder& operator=(StringHolder&& source)