Understanding Global and Local Variables in Programming
Global and local variables play essential roles in programming. Global variables can be accessed by all functions within a program, while local variables are restricted to specific functions. This text explains the concepts with analogies and code examples to illustrate how variables are scoped in programming languages. It also highlights the importance of properly managing data sharing between functions in a program.
Download Presentation
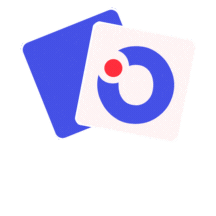
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
def incx(): x = 1 x = x + 1 return incx() print(x) # What is printed here? def decx(): x = 1 x = x - 1 return decx() print(x) # What is printed here? def squrx(): x = 2 x = x * x return squrx() print(x) # What is printed here?
Local Variables Remember the story of how functions work? Islands that come into existence when they are called (made to run) When they re done running, a volcano erupts and the function island is destroyed All parts of the island go away EXCEPT the return value That means that if a variable comes into existence within the function, it ONLY exists while the function is running. Unless that variable is returned, it ceases to exist when the function is done running
Programs: Programs are a set of functions running on a set of data. Each function has its own, well, function, or purpose. But together they make a whole program. You ve written a program for hangman. You wrote a bunch of individual functions. The functions all worked together to make one cohesive whole program The first function in a program is the main() function it calls the other functions, which may or may not call other functions, etc. Problem: how do all these functions share data so that if one function changes the data, another function can the use that updated data? One solution (relatively quick and dirty, but it works) are global variables
Another example: def incx(x): x = x + 1 return k = 2 #k is outside of the function incx(k) print(k) #what do you think is printed?
Global Variables x = 3 def incx(): global x Global variables outlast functions #This lets python know x is like #the moon and global Back to our analogy global variables are kind of like the moon all the islands see the same moon, and all islands share the light from the moon. x = x + 1 return Pushing the analogy a bit if one island changed the moon, all the other islands would then be dealing with a changed moon (pretend the moon can be changed). incx() print(x) By making a variable global, all functions can use it def decx(): global x #This is the same x used in incx() x = x - 1 return decx() print(x) If one function changes it, it stays changed for other functions NOTE: You MUST state you re using the global variable, or python will assume you want to use a local variable. If in the code -> you said: def squrx(): x = x * x return squrx() print(x) def squrx(): global x #this is the same x as in incx and decx x = x * x return squrx() print(x) You d get an error
st = "lli" TRY: def fun1(): st = "croc" st += "us" print(st) return() fun1() print(st) def fun2(): global st print(st) st = "po"+st+"wog" print(st) return() fun2() print(st) def fun3(): global st print(st) st = "umbre"+st[2:4] + "a" print(st) return fun3() print(st)
ls = [] def f1(x): global ls ls.append(x) return TRY: def f7(): global ls for y in range(len(ls)): print(ls[y],end="") print() return def f2(x,y): global ls ls.insert(x,y) return def main(): f1('s') f1('b') f2(1,'u') f2(0,'l') f6('yu',3,2) f3() f2(3,'b') f6('aim',1,2) f4('i') f2(6,'g') f5('d',2) f7() main() def f3(): global ls ls.pop() return def f4(x): global ls ls.remove(x) return def f5(x,y): global ls if (len(ls)>y): ls[y]=x return def f6(x,y,z): global ls if (y < z): if z<= len(ls): ls[y:z]=x elif (z < y): if y <= len(ls): ls[z:y]=x