Understanding File Operations in Python Programming
Learn about files in Python programming and how to perform file operations such as opening, reading, and writing. Discover the differences between text files and binary files, along with key attributes of files in Python.
Download Presentation
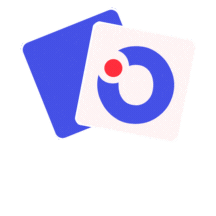
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
GE 8151 Problem Solving and Python Programming Topic: Files Operations Presented by, Dr.G.Rajiv Suresh Kumar, HoD/CSE,JCTCET
FILES Files A File is collection of data stored on a secondary storage device like hard disk. Data on non-volatile storage media is stored in named locations on the media called files. File is a named location on disk to store related information. While a program is running, its data is in memory. When the program ends, or the computer shuts down, data in memory disappears. To store data permanently, you have to put it in a file. Files are usually stored on a hard drive, floppy drive, or CD-ROM.
File continuation When there are a large number of files, they are often organized into directories (also called "folders"). Each file is identified by a unique name, or a combination of a file name and a directory name. Hence, in Python, a file operation takes place in the following order. Open a file Read or write (perform operation) Close the file
File Types Text files Binary files
Text files A text file is a file that contains printable characters and whitespace, organized into lines separated by newline characters. Text files are structured as a sequence of lines, where each line includes a sequence of characters. Since Python is specifically designed to process text files, it provides methods that make the job easy.
Binary files A binary file is any type of file that is not a text file. Because of their nature, binary files can only be processed by an application that know or understand the file s structure. In other words, they must be applications that can read and interpret binary.
File operation Open a file Read or write (perform operation) Close the file
Attributes of a file Attribute file.closed file.mode file.name Description If file is closed return true else false Returns one of the modes in which the current file is opened Returns the name of the file
Example for attributes fileread= open( text.txt , r+ ) print ( Name of the file : ,fileread.name) print ( Closed or not : ,fileread.closed) print( opening mode : ,fileread.mode) OUTPUT: Name of the file : text.txt Closed or not : False opening mode : r+
Opening a file Python has a built-in function open() to open a file. This function returns a file object, also called a handle, as it is used to read or modify the file accordingly. Syntax: Fileobject = open( filename or file path , access mode ) >>> f = open("test.txt") # open file in current directory by directly specifying the name of the file. >>> f = open("C:/Python33/README.txt") # specifying full path. >>> f = open( test.txt , r ) # open the file by mentioning file name and mode
File opening Modes Mode Description r Opens a file for reading only. The file pointer is placed at the beginning of the file. This is the default mode w Opens a file for writing only.Overwrites the file if the file exists.If the file does not exist, creates a new file for writing a Opens a file for appending. The file pointer is placed at the end of the file. If the file does not exist, creates a new file for writing r+ Opens a file for both reading and writing. The file pointer is placed at the beginning of the file. W+ Opens a file for both reading and writing. Overwrites the file if the file exists.If the file does not exist, creates a new file for reading and writing. Opens a file for both reading and appending. The file pointer is placed at the end of the file. If the file does not exist, creates a new file for reading and writing. A+
File opening Modes Mode Description Opens a file for both reading in binary format. The file pointer is placed at the beginning of the file rb Opens a file for writing only in binary format.Overwrites the file if the file exists.If the file does not exist, creates a new file for writing wb Opens a file for appending in binary format. The file pointer is placed at the end of the file. If the file does not exist, creates a new file for writing ab
FILE CLOSING When the operations that are to be performed o n a opened file are finished, we have to close the file in order to release the resources. The closing is done with a built-in function close() Syntax: fileobject.close() Example: f= open( text.txt , w ) #open a file #perform some operations f.close() #close the file
Writing to a file After opening a file, we have to perform some operations on the file. In order to write into a file, we have to open it with w mode, or a mode, or any other writing enabling mode. write() method enables us to write any string to a opened file. Syntax: Fileobject=open( file.txt , w ) Fileobject.write( String to be written ) Example: fo = open("foo.txt", "w") fo.write( "Python is a great language.\nYeah its great!!\n"); print( success ) fo.close()
Reading a file The text files can be read in four different ways listed below: Using read Method Using readlines Method Using readline Method Syntax: read() <file variable name>.read() When size is not specified entire file is read and the contents are placed in the left hand side variable. <file variable name>.read(<size>) When size is specified , the size number of characters are read. When an empty file is read no error is displayed but an empty string is returned.
readlines() <file variable name>.readlines(<size>) #size optional When size is not specified entire file is read and each line is added in a list. When size is specified , the lines that make up the size bytes are read.When an empty file is read no error is displayed but an empty list is returned.
Example for all types of reading a file: Assume the content of a file: Hello Good morning We are learning python Its very interesting
Program fileread = open( text.txt , r ) Str=fileread.read(10) Length=len(Str) print (str) print (Length) Str=fileread.read(20) print (str) Str= fileread.read() print (str) Str=fileread.readlines() print(Str) fileread.close()
Output: Hello Good 10 morning We are learn ing python Its very interesting Hello Good morning We are learning python Its very interesting
Renaming a file An existing file can be renamed by using the method rename() Syntax: os.rename(current filename, new filename) Example: >>> import os >>>os.rename( C:/Python27/myfile.txt , C:/Python27/myfile1.txt )
Deleting a File An existing file can be deleted by using the method remove(). Syntax: os.remove(filename) Example: >>> import os >>>os.remove( C:/Python27/myfile1.txt )
Format Operator Definition: The format operator is used to print the output in a desired format. The % operator is the format operator. It is also called as interpolation operator. The % operator is also used for modulus. The usage varies according to the operands of the operator.
General syntax: <format expression>%(values) # The values is a tuple with exactly the number of items specified by the format expression.
PROGRAM print( %d %(45)) print( %f %(3.14159265358979)) print( %0.2f %(3.141592653589)) print ( Decimal : %u %(24)) Print ( Octal : %o %(24)) Print ( Hexadecimal : %x %(1254)) Print ( Hexadecimal : %X %(1254)) X=40 print( The given integer is %d %X)
OUTPUT 45 3.141592 3.14 Decimal : 24 Octal : 30 Hexadecimal : 4e6 Hexadecimal : 4E6