Understanding Automatic Memory Management in Programming
Dive into the realm of automatic memory management, exploring garbage collection algorithms, dynamic memory handling, common mistakes to avoid, potential undesired outcomes, challenges of deallocation, controlled solutions for managing memory, and the benefits of automatic memory management in simplifying code and reducing errors.
Download Presentation
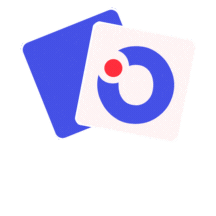
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Automatic Memory Management http://www.cs.tau.ac.il/~maon/teaching/2014- 2015/seminar/seminar1415a.html Semester A. Tuesday, 14:00-16:00. Schreiber 7 Noam Rinetzky Schreiber 123A http://www.cs.tau.ac.il/~maon/
Scope Automatic algorithms for automatic memory management aka garbage collection Sequential GC Parallel GC Concurrent GC Real-time GC Focus on correctness
Programs with dynamic memory Programs manipulate resources Files Processes / threads Connections Memory malloc() / new() free() / delete() . GC
Programming with dynamic memory typedef struct Data {int d; struct Data *n} Da; main(){ Da *p1 = (Da*) malloc(sizeof(Da)); p1 d = SECRET_KEY; Da *p2 = (Da*) malloc(sizeof(Da)); p2 d = 0; p1 n = p2; p2 n = null; } free(p1); free(p2)
Common mistakes Double free Memory leaks (no-free) Accessing dangling references Null-dereference Breaking invariants p1 n = p2; p2 n = null; p1 n = p2; p2 n = p1;
Undesired outcome Crashes Incorrect behavior Security vulnerabilities Loss of life Loss of money Loss of reputation Loss of Job
Deallocation Allocation is easy Deletion is hard Nasty bugs Hard to get right Defensive programming
Controlled Solutions Manual memory management Runtime: Monitoring execution environment Catches errors Expensive Compile-time: Verify memory safety Static analysis Fully automatic / User-provided annotations Conservative Problem is undecidable
Automatic Memory Management Exploit global knowledge Hard to de-allocate based on local reasoning Simplifies code, Reduce coupling, Reduces errors, costs Sensitive & Chaotic (Locality, Program)
GC for the rescue Double free Memory leaks (no-free) Accessing dangling references Null-dereference Breaking invariants p1 n = p2; p2 n = null; p1 n = p2; p2 n = p1;
GC Runtime environment recycles memory that will not be used in the future of the execution Cannot be used = unreachable Pros Safe Simple Cons Runtime overhead Imprecision (drag)
GC Challenges Unbounded number of resources Complicated data structures Efficiency Precision Correctness Multithreading makes things worse!
Comparing GC Algorithms Safety Throughput Completeness and promptness Pause Space overhead Language-specific optimizations Scalability and portability
More issues Performance overhead Experimental methodology
Terminology Heap Mutator & Collector Mutator roots References, fields, addresses Liveness, correctness, reachability Allocator
Schedule Topic Chapter Lesson 1 Date 28-10-14 Overview Chap.1 Mark-and-Sweep and Mark-compact GC Chap. 2,3 2 04-11-14 Copying GC and reference counting Chap. 4,5 3 11-11-14 Comparing GCs and allocation Chap. 6,7 4 18-11-14 Partitioning and generational GC Chap. 8,9,10 5 25-11-14 Runtime interface and language specific concerns Chap. 11,12 6 02-12-14 Concurrency preliminaries Chap. 13 7 09-12-14 Parallel GC Chap. 14 8 16-12-14 Concurrent GC Chap. 15 9 23-12-14 Concurrent mark-sweep GC Chap. 16 10 30-12-14 Concurrent copying and compaction GC Chap. 17 11 06-01-15 Concurrent reference-counting Chap. 18 12 13-01-15 Real-time GC Chap. 19 13 20-01-15
Requirements You are required to be present in every lesson unless coordinated ahead with the lecturer Meet me before lecture Sunday 1600-1700, Schreiber 123A
Requirements Give a 80 minutes talk about his or hers assigned topic. Answer students questions during the talk. Say something original Lead a discussion a summary discussion. Write a short (1 page) summary Participate in the discussions
Grades 70% Presentation 5% Original insight 10% Participation 15% Attendance
Paper Title Names of Authors Your Name + date
Outline of talk Introduction Suggested Solution Evaluation Related work Conclusions Your own conclusions
Introduction Problem area Technical challenged addressed Why is it important What is the main insight How is main insight utilized (high level)
Solution Technical description Algorithm Correctness Complexity Choose key subset of details Use examples + diagrams
Evaluation Experiments Benchmarks Conclusions
Related work What other solutions are out there How do they compare Pros Cons
Conclusions What was done Why is it important Novel idea What we learned
Your own conclusion Surprise me