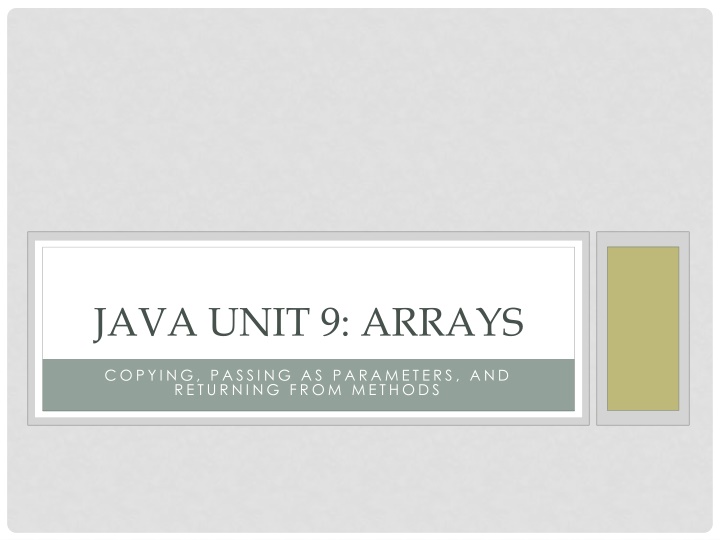
Understanding Array Copying in Java
Learn how to efficiently copy arrays in Java by distinguishing between copying references and contents. Arrays being objects require careful handling to ensure proper copying and avoid confusion. Explore examples and best practices for array copying in Java programming.
Download Presentation
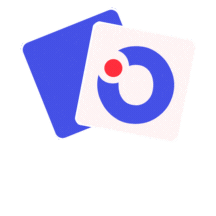
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
JAVA UNIT 9: ARRAYS C O PYI NG, PA SSI NG A S PA R A M ETER S, A ND R ETU R NI NG F R O M M ETHO DS
COPYING Copying is a common operation on arrays. It is not difficult, but it is a possible source of confusion. Arrays are objects. This means that you have to distinguish between copying references and copying contents.
COPYING A REFERENCE Here is an example of copying a reference: double[] myArray = new double[10]; double[] yourArray; yourArray = myArray; myArray is brought into existence, but yourArray is simply a reference to myArray . So, you have two references to the same array.
COPYING A REFERENCE So, if you were to do the following: yourArray[0] = 3.0; System.out.println(myArray[0]); The value 3.0 would be printed. There is no effective difference between myArray[0] and yourArray[0]. They refer to the same value in the same array.
COPYING A REFERENCE myArray double[10] yourArray
COPYING CONTENTS Of course, it is also possible to make copies of the contents of arrays. This could be accomplished as follows: double[] myArray = new double[10]; double[] yourArray = new double[myArray.length]; for(int j = 0; j < myArray.length; j++) { yourArray[j] = myArray[j]; }
COPYING CONTENTS In looking at the code above, it is important to note two things: First of all, yourArray isn t simply declared as an array reference. It is declared and constructed as a double array with the same number of elements as myArray. The loop does an element-by-element copy of the contents from myArray into yourArray.
COPYING CONTENTS Copying array contents is a common enough action that Java provides a static method to do so. In order to have access to the method you need the following import statement: import java.lang.System;
COPYING CONTENTS SYSTEM.ARRAYCOPY In the API documentation for the System class you will find this method static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length) The parameters declared as Objects are the arrays, where src and dest stand for source and destination, respectively. The method is designed to allow partial copying. You specify both the source and destination arrays, the place in the source and destination arrays to start copying to and from (srcPos, destPos), plus a length , which is the number of elements to copy. You must be careful that destPos + length is not greater than the destination array s size.
COPYING CONTENTS SYSTEM.ARRAYCOPY Here is an example: System.arraycopy(myArray, 0, yourArray, 0, myArray.length); This takes the contents of yourArray, and copies it into myArray. Because it is set to start copying from index 0 of myArray to index 0 of yourArray, and it is set to copy an amount of elements equal to myArray, all of the contents from myArray is copied into yourArray.
COPYING CONTENTS SYSTEM.ARRAYCOPY This System method takes arrays as explicit parameters. In other words, in the method definition, the parameters src and dest, which are typed as Object, take array references when the method is called. In the example above arrays are expected as parameters, but the formal method parameters are typed Object. This allows an array containing any type of element to be copied by the method. How this works will be explained in more detail in a future unit. For now, you can just accept that this code works.
PASSING AS PARAMETERS Just as the system has methods which accept arrays as parameters, it is also possible for programmers to write methods which accept arrays as parameters. In programmer written code, it is desirable to declare the parameter to a specific kind of array (rather than Object, as with System.arraycopy).
PASSING AS PARAMETERS Here is a simple static method contained in a class: public class MyMethodContainer1 { public static void squareArray(double[] anArray) { for(int j = 0; j < anArray.length; j++) { anArray[j] = anArray[j] * anArray[j]; } } }
PASSING AS PARAMETERS Notice that this method is declared void. It doesn t return anything. The important thing to remember is that when the method is called, a reference to an array is passed to it. This means that any changes made to the array in the method are reflected in the calling program. There are not two arrays; there are two references to the same array. One reference is in the calling program and the other reference is in the method. The effect of calling the method is reflected directly in the contents of the array in the program after the call is made.
PASSING AS PARAMETERS In a calling program where myArray has been declared and created, a call to the method would look as shown below. MyMethodContainer1.squareArray(myArray); Note that there are no square brackets used. myArray is a one dimensional array and its name alone refers to the array. The method doesn t take an array element (with subscript) as the parameter. It takes the whole array.
RETURNING AN ARRAY It is also possible for a method to have an array as its return type. If so, the returned array is created from scratch within the body of the method. This array may be entirely independent of any other array. You may also want to write a method that accepts an array as a parameter and works with its contents without changing the original. In that case, it is possible to pass an array as a parameter, create a new array in the method, copy the contents of the parameter to the new array, process the new array, and return the new array as the result of the method, leaving the original unchanged.
RETURNING AN ARRAY public class MyMethodContainer2 { public static double[] squareArray(double[] anArray) { double[] returnArray = new double[anArray.length]; for(int j = 0; j < returnArray.length; j++) { returnArray[j] = anArray[j] * anArray[j]; } return returnArray; } }
RETURNING AN ARRAY The method is declared to return an array of the same type as the input parameter. At the beginning of the method a new array of the same type and length as the input parameter is constructed. Then the contents of the input array are copied, element-by-element, into the new array. Finally, the new array is returned.
RETURNING AN ARRAY Assuming that myArray already existed, the call to the method in a program would take this form: double[] yourArray = MyMethodContainer2.squareArray(myArray); There is no need to separately create and give a size to yourArray. It is simply a reference which receives the created and sized array of doubles returned by the method.
RETURNING AN ARRAY Two dimensional arrays can also be passed as parameters. Just like with one dimensional arrays, when doing so, the whole array is passed by means of the name without square brackets. Including brackets and subscripts can indicate individual elements, or with two dimensional arrays, can indicate rows. You need to be careful not to mistakenly include unnecessary things when declaring formal parameters or writing the code for a call.