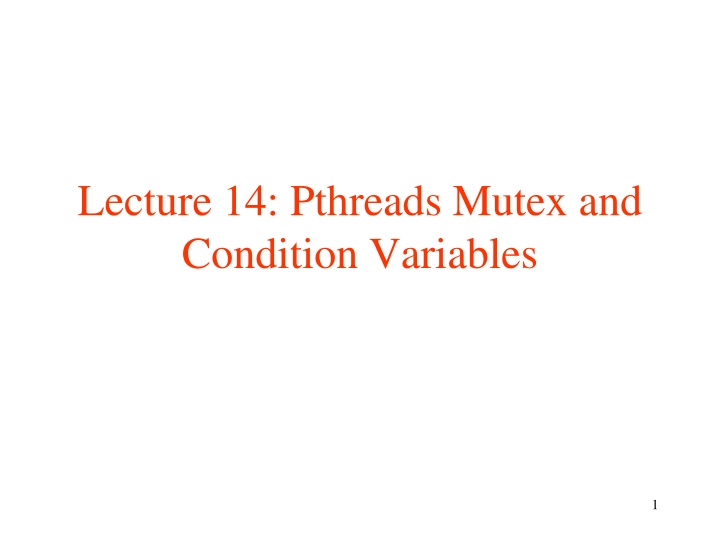
Pthreads Mutex and Condition Variables Explained
Explore the concepts of Pthreads mutex, condition variables, and their APIs with practical examples for achieving mutual exclusion and synchronization in multithreaded programming. Learn about semaphore implementations, mutex usage in POSIX threads, and the role of condition variables in blocking threads based on specific conditions.
Download Presentation
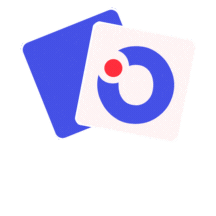
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Lecture 14: Pthreads Mutex and Condition Variables 1
Review: Mutual Exclusion Software solution Disabling interrupts Strict alternation Peterson s solution Hardware solution TSL/XCHG Semaphore 2
Review: Semaphore Implementation down(&S): If (S=0) then Suspend thread, put into a waiting queue Schedule another thread to run Else decrement S and return up(&S): Increment S If any threads in waiting queue, then release one of them (make it ready ) Both the above are done atomically by disabling interrupts by TSL/XCHG 3
In this lecture Pthreads APIs Mutex Condition variables 4
Mutex usage in POSIX Threads pthread_mutex_t m; pthread_mutex_init(&m); pthread_mutex_lock(&m); critical_region(); pthread_mutex_unlock(&m); 6
pthread_mutex_trylock Return fails when the mutex is already locked Used for implementing busy waiting 7
Mutex vs condition variables Mutex is good to guarantee mutual exclusion Allow and block access to the critical regions Conditional variables Block threads due to some condition not met 8
Condition Variables Allows a thread to wait till a condition is satisfied Testing the condition must be done within a mutex With every condition variable, a mutex is associated 9
Comparison with Semaphores If a signal is sent to a conditional variable on which no thread is waiting, the signal is lost Semaphore will accumulate signals by up() 11
Condition Variables pthread_cond_t condition_variable; pthread_mutex_t mutex; Signaling Thread: Waiting Thread: pthread_mutex_lock(&mutex); pthread_mutex_lock(&mutex); /* change variable value */ while (condition not satisfied) { pthread_cond_wait( &condition_variable, &mutex); } if (condition satisfied) { pthread_cond_signal( &condition_variable); } pthread_mutex_unlock(&mutex); /* pthread_mutex_unlock(&mutex); Alternative to cond_signal is pthread_cond_broadcast( &condition_variable); */ 12
Condition variable and mutex A mutex is passed into wait: pthread_cond_wait(cond_var, mutex) Mutex is unlocked before the thread sleeps Mutex is locked again before pthread_cond_wait() returns Safe to use pthread_cond_wait() in a while loop and check condition again before proceeding 13
Example Usage Write a program using two threads Thread 1 prints hello Thread 2 prints world Thread 2 should wait till thread 1 finishes before printing Use a condition variable 14
Using condition variables int thread1_done = 0; pthread_cond_t cv; pthread_mutex_t mutex; Thread 1: Thread 2: printf( hello ); pthread_mutex_lock(&mutex); pthread_mutex_lock(&mutex); thread1_done = 1; pthread_cond_signal(&cv); pthread_mutex_unlock(&mutex); pthread_cond_wait(&cv, &mutex); printf( world\n ); pthread_mutex_unlock(&mutex); What is the problem in this implementation? 15
Using condition variables int thread1_done = 0; pthread_cond_t cv; pthread_mutex_t mutex; Thread 1: Thread 2: printf( hello ); pthread_mutex_lock(&mutex); pthread_mutex_lock(&mutex); thread1_done = 1; pthread_cond_signal(&cv); pthread_mutex_unlock(&mutex); while (thread1_done == 0) { pthread_cond_wait(&cv, &mutex); } printf( world\n ); pthread_mutex_unlock(&mutex); 16
Multiple Locks proc1( ) { pthread_mutex_lock(&m1); /* use object 1 */ pthread_mutex_lock(&m2); proc2( ) { pthread_mutex_lock(&m2); /* use object 2 */ pthread_mutex_lock(&m1); /* use objects 1 and 2 */ /* use objects 1 and 2 */ pthread_mutex_unlock(&m2); pthread_mutex_unlock(&m1); } pthread_mutex_unlock(&m1); pthread_mutex_unlock(&m2); } thread a thread b 17
Deadlock Thread 1 Mutex m1 Mutex m2 Thread 2 18