Mathematical Functions Library in C Programming
Explore a collection of mathematical functions in C programming that operate on double values, including functions for square root, power, absolute value, ceil, and floor operations, with code examples and output explanations.
Download Presentation
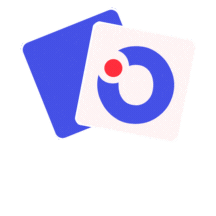
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CSC 215 Mathematical Functions
math.h Include mathematical functions All functions in this library take double as an argument All functions in this library return double as the result.
double sqrt(double x) Parameter: x This is the floating point value. Return Value: Returns the square root of x. Example: #include <stdio.h> #include <math.h> int main () { printf("Square root of %lf is %lf\n", 16.0, sqrt(16.0) ); return(0); } Output: Square root of 16.000000 is 4.000000
double pow (double x, double y) Parameters x This is the floating point base value. y This is the floating point power value. Return Value: This function returns the result of raising x to the power y. Example: #include <stdio.h> #include <math.h> int main () { printf("Value 2.0 ^ 3 = %lf\n", pow(2.0, 3)); return(0); } Output: Value 2.0 ^ 3 = 8.000000
double fabs(double x) Parameters x This is the floating point value. Return Value This function returns the absolute value of x. Example: #include <stdio.h> #include <math.h> int main () { int a, b; a = 1234; b = -344; double c = -135; printf("The absolute value of %d is %lf\n", a, fabs(a)); printf("The absolute value of %d is %lf\n", b, fabs(b)); printf("The absolute value of %lf is %lf\n", c, fabs(c)); return(0); } Output: The absolute value of 1234 is 1234.000000 The absolute value of -344 is 344.000000 The absolute value of -135.000000 is 135.000000
double ceil (double x) Parameters x This is the floating point value. Return Value This function returns the smallest integral value not less than x. Example: #include <stdio.h> #include <math.h> int main () { float val1=1.6, val2=1.2, val3=2.8; int val4 = 2; printf ("value1 = %.1lf\n", ceil(val1)); printf ("value2 = %.1lf\n", ceil(val2)); printf ("value3 = %.1lf\n", ceil(val3)); printf ("value4 = %.1lf\n", ceil(val4)); return(0); } Output: value1 = 2.0 value2 = 2.0 value3 = 3.0 value4 = 2.0
double floor (double x) Parameters x This is the floating point value. Return Value This function returns the largest integral value not greater than x. Example: #include <stdio.h> #include <math.h> int main () { float val1 = 1.6, val2 = -9.8, val3 = 2.8; int val4 = 2; printf("Value1 = %.1lf\n", floor(val1)); printf("Value2 = %.1lf\n", floor(val2)); printf("Value3 = %.1lf\n", floor(val3)); printf("Value4 = %.1lf\n", floor(val4)); return(0); } Output: Value1 = 1.0 Value2 = -10.0 Value3 = 2.0 Value4 = 2.0
double fmod(double x, double y) Parameters x This is the floating point value with the division numerator i.e. x. y This is the floating point value with the division denominator i.e. y .Return Value This function returns the remainder of dividing x/y. Example: #include <stdio.h> #include <math.h> int main () { float a = 9.2; float b = 3.2; int c = 2; printf("Remainder of %f / %d is %lf\n", a, c, fmod(a,c)); printf("Remainder of %f / %f is %lf\n", a, b, fmod(a,b)); return(0); } Output: Remainder of 9.200000 / 2 is 1.200000 Remainder of 9.200000 / 3.200000 is 2.800000
double log10(double x) Parameters x This is the floating point value. .Return Value This function returns the common logarithm of x, for values of x greater than zero Example: #include <stdio.h> #include <math.h> int main () {double x, ret; x = 10000; /* finding value of log1010000 */ ret = log10(x); printf("log10(%lf) = %lf\n", x, ret); Output: log10(10000.000000) = 4.000000 return(0); }
Random Number Generation The function rand() computes a sequence of pseudo-random integers in the range zero to RAND_MAX, which is defined in <stdlib.h>. RAND_MAX is a constant whose default value may vary between implementations but it is granted to be at least 32767. int rand(void) Return Value: This function returns an integer value between 0 and RAND_MAX.
Example: #include <stdio.h> #include <stdlib.h> int main() { int i, n = 5; /* Print 5 random numbers from 0 to 49 */ for( i = 0 ; i < n ; i++ ) { printf("%d\n", rand() % 50); } Output: 33 36 27 15 43 return(0); }