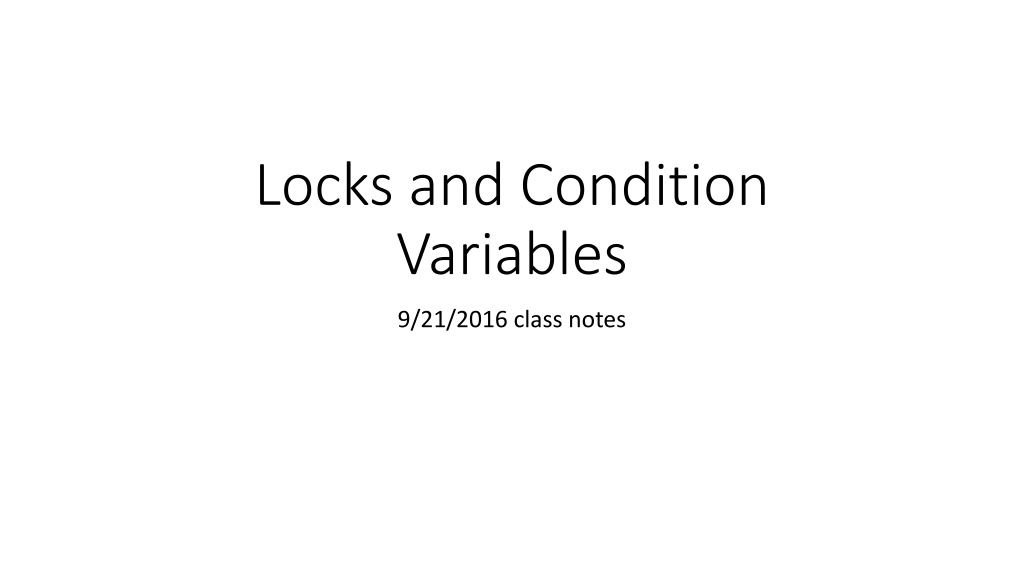
Java Locks and Condition Variables Overview
Learn about implicit and explicit locks, condition variables, and synchronization in Java, including how to use ReentrantLock and ReentrantReadWriteLock. Understand the concepts of guard locking and leveraging Java's built-in synchronization methods to manage multithreaded applications effectively.
Download Presentation
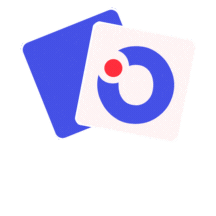
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Locks and Condition Variables 9/21/2016 class notes
Implicit locks in Java Every object in Java has an internal, unnamed lock. public void synchronized METHOD(PARAMS) { // The thread that calls this method must acquire the implicit lock in // *this* object before gaining entry to the method. Returning from the // method releases the lock. } // In any block of code in this object: synchronized(this) { // The code inside this block uses the implicit lock. }
@GuardedBy(lockObject) For every mutable data field accessible to multiple threads that is *not* thread safe: @GuardedBy(lockObject) immediately before that data field declaration states the lock that guards access to the data field.
Implicit condition variables Also built into every Java object. It is a mechanism for releasing the lock *and* waiting for a message from another thread who acquires the lock, and then re-acquiring the lock from within the synchronized. With implicit locks & implicit condvars, use the lock object s wait() to release-wait-reacquire the lock in one thread, and use the lock object s notifyAll() or notify() to send the message from another thread.
Explicit, reentrant locks java.util.concurrent.locks.ReentrantLock A reentrant lock allows a single thread to lock it multiple times. It must release the lock the same number of times. Python and the C/POSIX libraries also support non-reentrant locks. lock() and its variants are explicit methods for acquiring the lock. PUT THE unlock() call in a finally clause, for which the lock was acquired before entering the try clause. Not doing so costs points! Unlike synchronized methods/blocks, a thread can hold an explicit lock even after returning from the acquiring method.
Condition variables for explicit locks mylock.newCondition() returns a java.util.concurrent.locks associated with mylock. The explicit condvar has methods await(), signal() and signalAll() instead of wait()/notify()/notifyAll(), which are associate with an implicit lock contained in each Java Object.
Misc. Thread.start() and Thread.join() are additional synchronization methods. java.util.concurrent.locks.ReentrantReadWriteLock provides a read/ write lock. A read / write lock allows multiple reader threads or a single writer thread to acquire the lock at a given time. Look at java.util.Collections unmodifiable*() (which does not guarantee an immutable object is returned), and synchronized*() which wraps a lock around each access and mutation method. The latter does not solve Iterators being atomic.
Use of a ReentrantLock and @GuardedBy annotation /home/KUTZTOWN/parson/multip/spring2015/spring2015exam/Prod ucerConsumerLListReentrantLock.java