Introduction to C++ String Class and Its Applications
This chapter explores the C++ string class and its various applications through examples and explanations. It covers different ways to initialize strings, such as by raw string, copying from another string, and character occurrence. The chapter delves into accessing and manipulating string elements, including clearing the string, accessing specific characters, and appending part of another string. Additionally, it discusses using functions like find, substr, and erase for efficient string processing.
Download Presentation
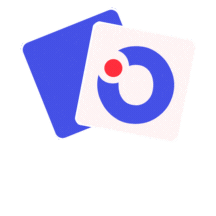
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Introduction to programming with C++ C++ Introduction Chapter Five Yasser Abdulhaleem Abdulkareem Almadany 1
C++ string class and its applications We can declare any string by another way as below. initialization by raw string: string str1("John Wick"); initialization by another string: string str2(str1); initialization by character with number of occurrence: string str3(5,'#'); Yasser Abdulhaleem Abdulkareem Almadany 2
String class and its applications(cont'd.) initialization by part of another string from 6th index (second parameter) and 6 characters (third parameter): string str4(str1,6,6); initialization by part of another string: string str5(str2.begin(), str2.begin() + 5); We can see the output by example below to understand. Example: Yasser Abdulhaleem Abdulkareem Almadany 3
String class and its applications(cont'd.) #include<iostream> #include<string> using namespace std; int main() { string str1("John Wick Resurrection"); string str2(str1); string str3(5,'$'); string str4(str1,6,6); string str5(str2.begin(),str2.begin()+5); cout<<str1<<endl; cout<<str2<<endl; cout<<str3<<endl; cout<<str4<<endl; cout<<str5<<endl; system("pause"); return 0; } Yasser Abdulhaleem Abdulkareem Almadany 4
String class and its applications(cont'd.) We can use clear function to delete all character from string: str4.clear(); A particular character can be accessed using at which is the same as [] operator: char ch = str6.at(2); Another version of append, which appends part of other string: str4.append(str6, 0, 6); Yasser Abdulhaleem Abdulkareem Almadany 5
String class and its applications(cont'd.) We can use find to returns index where pattern is found: str6.find(str4); We can use substr(a, b) function to returns a substring of b length starting from index a: str6.substr(7, 3); We can use erase(a, b) to delete b characters at index a: str6.erase(7, 4); Yasser Abdulhaleem Abdulkareem Almadany 6
String class and its applications(cont'd.) We can use replace(a, b, str) to replace b characters from a index by str: str6.replace(2, 7,"ese are test"); We can use swap(str) to swap values of two strings: str2.swap(str3); We can use insert(a, str) to insert string from a index: str1.insert(0, Welcome to "); Yasser Abdulhaleem Abdulkareem Almadany 7
String class and its applications(cont'd.) We can see the output by example below to understand. Example: #include<iostream> #include<string> using namespace std; int main() { string str1("John Wick Resurrection"); string str4(str1,6,6); string str6(str4); str4.clear(); Yasser Abdulhaleem Abdulkareem Almadany 8
String class and its applications(cont'd.) cout<<str4<<endl; char ch=str6.at(2); cout<<"third character of string is : "<<ch<<endl; str4.append(str6,0,6); cout<<str6<<endl; cout<<str4<<endl; str6=str1; cout<<str6.find(str4)<<endl; cout<<str6.substr(7,3)<<endl; cout<<str6.substr(7)<<endl; Yasser Abdulhaleem Abdulkareem Almadany 9
String class and its applications(cont'd.) str6.erase(7,4); cout<<str6<<endl; str6.swap(str1); cout<<str1<<endl; cout<<str6<<endl; str6.replace(0,9,"Welcome to"); cout<<str6<<endl; str6.insert(10," John Wick"); cout<<str6<<endl; system("pause"); return 0; } Yasser Abdulhaleem Abdulkareem Almadany 10
Operators that are used to compare strings in C ++ its symbol example operator name Equal to == (a == b) Not equal to != (a != b) Greater than > (a > b) Less than < (a < b) Greater than or Equal to >= (a >= b) Less than or Equal to <= (a <= b) Yasser Abdulhaleem Abdulkareem Almadany 11
Operators that are used to compare strings in C ++ (cont'd.) We can see the output by example below to understand. Example: #include<iostream> #include<string> using namespace std; int main() { string str1("John Wick Resurrection"); string str2("Welcome to John Wick Resurrection"); Yasser Abdulhaleem Abdulkareem Almadany 12
Operators that are used to compare strings in C ++ (cont'd.) cout<<(str1==str2)<<endl; cout<<(str1>str2)<<endl; cout<<(str1>=str2)<<endl; cout<<(str1<str2)<<endl; cout<<(str1<=str2)<<endl; cout<<(str1!=str2)<<endl; system("pause"); return 0; } Yasser Abdulhaleem Abdulkareem Almadany 13
C++ Math C++ has many functions that allows you to perform mathematical tasks on numbers. max and min: The max(x,y) function can be used to find the highest value of x and y: Example: #include <iostream> #include <cmath> using namespace std; int main() { cout << max(5, 10); return 0; } Yasser Abdulhaleem Abdulkareem Almadany 14
C++ Math(cont'd.) And the min(x,y) function can be used to find the lowest value of x and y. Example: #include <iostream> #include <cmath> using namespace std; int main() { cout << min(5, 10); return 0; } Yasser Abdulhaleem Abdulkareem Almadany 15
C++ <cmath> Header Other functions, such as sqrt (square root), round (rounds a number) and log (natural logarithm), can be found in the <cmath> header file. Example: #include <iostream> #include <cmath> using namespace std; int main() { cout << sqrt(64) << "\n"; cout << round(2.6) << "\n"; cout << log(2) << "\n"; return 0; } Yasser Abdulhaleem Abdulkareem Almadany 16
Other Math Functions A list of other popular Math functions (from the <cmath> library) can be found in the table below: Function Description abs(x) Returns the absolute value of x cbrt(x) Returns the cube root of x ceil(x) Returns the value of x rounded up to its nearest integer cos(x) Returns the cosine of x, in radians fabs(x) Returns the absolute value of a floating x sqrt(x) Returns the square root of x Yasser Abdulhaleem Abdulkareem Almadany 17
Other Math Functions(cont'd.) fdim(x, y) Returns the positive difference between x and y floor(x) Returns the value of x rounded down to its nearest integer fmax(x, y) Returns the highest value of a floating x and y fmin(x, y) Returns the lowest value of a floating x and y fmod(x, y) Returns the floating point remainder of x/y pow(x, y) Returns the value of x to the power of y sin(x) Returns the sine of x (x is in radians) tan(x) Returns the tangent of an angle Yasser Abdulhaleem Abdulkareem Almadany 18
Other Math Functions(cont'd.) Example: #include <iostream> #include <cmath> using namespace std; int main() { cout<<abs(-64)<<"\n"; cout<<cbrt(64)<<"\n"; cout<<ceil(5.6)<<"\n"; cout<<cos(0)<<"\n"; cout<<fabs(-1.5)<<"\n"; return 0; } Yasser Abdulhaleem Abdulkareem Almadany 19
Other Math Functions(cont'd.) Example: #include <iostream> #include <cmath> using namespace std; int main() { cout<<sqrt(64)<<"\n"; cout<<fdim(5,3 )<<"\n"; cout<<floor(2.65)<<"\n"; cout<<fmax(2.9, 1.2)<<"\n"; cout<<fmin(2.9, 1.2)<<"\n"; cout<<fmod(6.5, 3.1)<<"\n"; cout<<pow(10, 2)<<"\n"; cout<<sin(-23)<<"\n"; cout<<tan(-12.6)<<"\n"; return 0; } Yasser Abdulhaleem Abdulkareem Almadany 20