File I/O in C++: File Management and Operations
Learn about file input/output operations in C++. Understand how to open files with different modes using functions like `open()` in `fstream`, `ifstream`, and `ofstream` classes. Explore file opening modes such as reading, writing, appending, truncating, and more. Discover how to handle file formats and work with streams of characters and numbers. Dive into examples of writing to and reading from files in C++.
Download Presentation
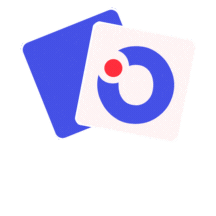
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
You are allowed to download the files provided on this website for personal or commercial use, subject to the condition that they are used lawfully. All files are the property of their respective owners.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author.
E N D
Presentation Transcript
Open() function Open() is a member function in each classes ( fstream, ifstream, ofstream) The name of file Void fstream :: open ( const char *filename, openmode mode); Optiional to determine how the file is opened Void ifstream ::open ( const char *filename, openmode mode= ios:: in); Void ofstream :: open ( const char *filename, openmode mode= ios:: out | iso :: trunc); You can combine 2 or more of these value by ORing them together
File Open Mode Name Description ios::in ios::out ios::app Open file to read Open file to write All the data you write, is put at the end of the file. It calls ios::out All the data you write, is put at the end of the file. It does not call ios::out Deletes all previous content in the file. (empties the file) If the file does not exists, opening it with the open() function gets impossible. If the file exists, trying to open it with the open() function, returns an error. Opens the file in binary mode. ios::ate ios::trunc ios::nocreate ios::noreplace ios::binary
File Open Mode #include <fstream> using namespace std; void main() { ofstream outFile("file1.txt", ios::out); outFile << "That's new!\n"; outFile.close(); } If you want to set more than one open mode, just use the OR operator- |. This way: ios::ate | ios::binary
File format In c++ files we (read from/ write to) them as a stream of characters What if I want to write or read numbers ?
Example writing to file #include <iostream> #include <fstream> using namespace std; void main() { ofstream outFile; // open an exist file fout.txt outFile.open("number.txt",ios::app); if (!outFile.is_open()) { cout << " problem with opening the file ";} else {outFile <<200 <<endl ; cout << "done writing" <<endl;} outFile.close(); }
Example Reading from file #include <iostream> #include <fstream> #include <string> #include <sstream> using namespace std; void main() {//Declare and open a text file ifstream INFile("number.txt"); string line; int total=0; while(! INFile.eof()) {//fetch line from number.txt and put it in a string getline(INFile, line); //converting line string to int stringstream(line) >> total; cout << line <<endl; cout <<total +1<<endl;} INFile.close(); // close the file }