Understanding Rust Expressions and Declarations in System Software Development
Rust is an expression language where most things have values. Learn about Rust expressions, blocks, declarations, conditional expressions, multi-case expressions, and iteration expressions in system software development. Explore the execution semantics, syntax, and best practices for writing safe and efficient code in Rust.
Download Presentation
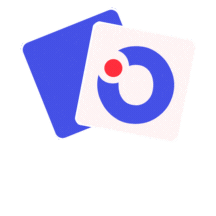
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
E81 CSE 542S: Concurrency and Memory Safe System Software Development Expressions Department of Computer Science & Engineering Chris Gill cdgill@wustl.edu 1
Expressions in Rust Rust is an expression language So, most things in Rust have values E.g., ending a function with Ok(()) (and no semicolon) generates its return value Expressions have execution semantics E.g., operator precedence as well as associativity, implicitly as well as explicitly 2 CSE 542S Concurrency and Memory Safe System Software Development
Blocks and Semicolons A block encapsulates a sequence of expressions and produces a value E.g., None => {println!( all good ); Ok(())} A semicolon drops an expression s value Useful if default expression type isn t what you want (e.g., if without else returns ()) 3 CSE 542S Concurrency and Memory Safe System Software Development
Declarations Some declarations introduce functions, structs, and other items Variable declarations give a name and optionally a type and initializer The compiler often can figure out the type and default initialization if they re omitted The initialization value may come from a function result, block, or other expression 4 CSE 542S Concurrency and Memory Safe System Software Development
Conditional Expressions Blocks can be nested using if and else All branches must return the same type E.g., if i > j {i} else {j} The if let form captures the result of a function call, expression, etc. as well E.g., if let Err(err) = myfunc() {log(err);} else {process_result();} 5 CSE 542S Concurrency and Memory Safe System Software Development
Multi-case Expressions A match expression is similar to a switch or case statement in other languages You must cover all cases either explicitly or via the default match pattern _ The patterns are evaluated in the order they are given (earlier has precedence) Also can destructure tuples using match E.g., match t {(x, y) => do_func(x,y);} 6 CSE 542S Concurrency and Memory Safe System Software Development
Iteration Expressions The while and forexpressions don t, but loop lets you specify/return a value E.g., loop {if done() break val;} Like an if let expression, a while let expression also captures a value A for expression uses closed/open ranges, consumes non-copy types values This suggests using references to iterate over collections of variables (e.g., in vector) 7 CSE 542S Concurrency and Memory Safe System Software Development
Studio 5 Revisit operator precedence and associativity Explore how to capture/use expression values Examine how reference vs. move semantics play into iteration over collections Studios 1 through 11 are due by 11:59pm on the night before Exam 1 Submit as soon as each is done so you get feedback and can resubmit any that may be marked incomplete 8 CSE 542S Concurrency and Memory Safe System Software Development